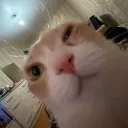
Heyo.
This is a continuation of #Server Action Not Working?.
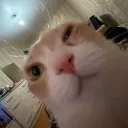
the code using appwrite.ts:
import {
Client,
Account,
Teams,
Databases,
Storage,
Functions,
Messaging,
Locale,
Avatars,
} from 'appwrite'
export const client = new Client()
.setEndpoint(`${process.env.NEXT_PUBLIC_API_URL}/v1`)
.setProject(`${process.env.NEXT_PUBLIC_APPWRITE_PROJECT_ID}`)
export const account: Account = new Account(client)
export const teams: Teams = new Teams(client)
export const databases: Databases = new Databases(client)
export const storage: Storage = new Storage(client)
export const functions: Functions = new Functions(client)
export const messaging: Messaging = new Messaging(client)
export const locale: Locale = new Locale(client)
export const avatars: Avatars = new Avatars(client)
export { ExecutionMethod, ID, Query } from 'appwrite'
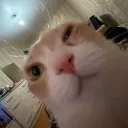
Having a page on SSR, with a client component imported using for example this kind of code:
'use client'
import { storage } from '@/app/appwrite-client'
export default function FetchGallery({ name, gallery }) {
const getGalleryImageUrl = (galleryId: string) => {
console.log(galleryId)
if (!galleryId) return
const imageId = storage.getFileView('655ca6663497d9472539', `${galleryId}`)
return imageId.href
}
const url = getGalleryImageUrl(gallery?.galleryId)
console.log(url)
// The rest of the component remains unchanged with conditional rendering based on the data's availability.
return (
<img
src={url}
alt={name || 'Image'}
className={`imgsinglegallery mx-auto h-[400px] w-auto max-w-full rounded-lg object-contain`}
/>
)
}
Will spam "window is not defined" every time you refresh the page.

example of mine:
//database.jsx
import { account, client, database, storage, teams, userId } from "@/utils/appwrite";
import { Query,ID } from "appwrite";
import { publish } from "./events";
export async function GetServerSpells(IncomingFilters) {
const activeFilters = IncomingFilters || [];
const queryOptions = [Query.limit(850)];
if (activeFilters.length > 0) {
//Include active filters in the query
activeFilters.forEach((filter) => {
switch (filter[0]) {
case "SpellName":
//console.log(`Database.jsx: Got: SpellName with filters ${filter[0]} | ${filter[1]}}`)
queryOptions.push(Query.search(filter[0], `./${filter[1]}/`));
break;
case "Class":
queryOptions.push(Query.search(filter[0], filter[1]));
break;
default:
queryOptions.push(Query.equal(filter[0], filter[1]));
break;
}
});
}
//console.log(`trying to find spells with filters: ${queryOptions}`);
const x = await database.listDocuments(
process.env.NEXT_PUBLIC_DATABASE_ID,
process.env.NEXT_PUBLIC_COLLECTION_SPELL_ID,
queryOptions
);
const list = await SortSpells(x["documents"]);
//console.log(`Database.jsx: Found list: ${JSON.stringify(list)}`);
return list;
}

//spelllist.jsx
"use client"
import { useState, useEffect } from "react";
import { GetServerSpells } from "@/utils/Database";
const data = async () => {
setSpellData(await GetServerSpells());
};
useEffect(() => {
data();
}, []);

Now this is javascript but it might to the same for typescript

cause client to server is just not possible with nextjs
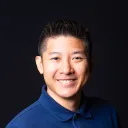
i think you can do the same thing with the node sdk now
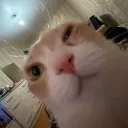
So just use a server action?
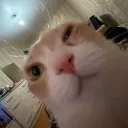
I'll try out some stuff tomorrow.
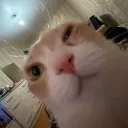
Does realtime work using the node sdk? 🤔
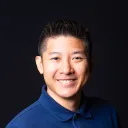
No. Please create a new post for different topics
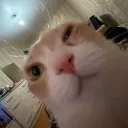
Well, it's still the same topic. You just wanted me to use something else.
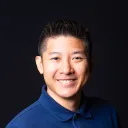
Your original topic was using node sdk and getting window not defined. The new topic is about realtime
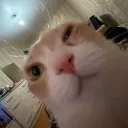
Well, my issue is still about window is not defined, because I basically am not allowed to use the appwrite sdk in any way, to not get the error.
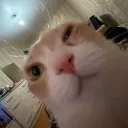
I can try with realtime, but I figure that everything is built the same way and something in the appwrite client sdk shows that error. So far everything else has given the error, so expected is that realtime of all things, will too.
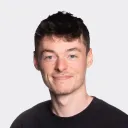
It's spamming on the server console, not the browser right?
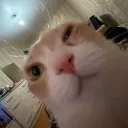
Both
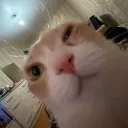
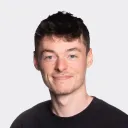
Right. Like xmaniaxz suggested, I think you need to put calls to the @/app/appwrite-clietn
inside a useEffect, that also checks window !== undefined
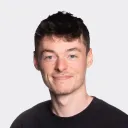
We might be able to solve this in the SDK by not depend on window. We can do is throw a more descriptive error. Is it just the getFileView
endpoint you see this with? Or all services/endpoints
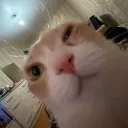
All, just make a simple createEmailPasswordSession auth page
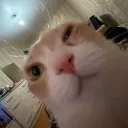
the moment I try to use the client sdk, basically
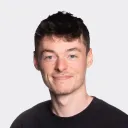
Right, it's not easy to see where that faulty window
access comes from unfortunately, but I will take some time today to track it down
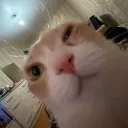
Hey!
Did you come closer towards tracking it down?
Recommended threads
- Stuck in "deleting"
my parent element have relationship that doesnt exist and its stuck in "deleting", i cant delete it gives me error: Collection with the requested ID could not b...
- Help with 409 Error on Relationship Setu...
I ran into a 409 document_already_exists issue. with AppWrite so I tried to debug. Here's what I've set up: Collection A has 3 attributes and a two-way 1-to-m...
- Database Double Requesting Error.
I am getting error for creating new document in an collection with new ID.unique() then too getting error of existing document. When button is pressed one docum...
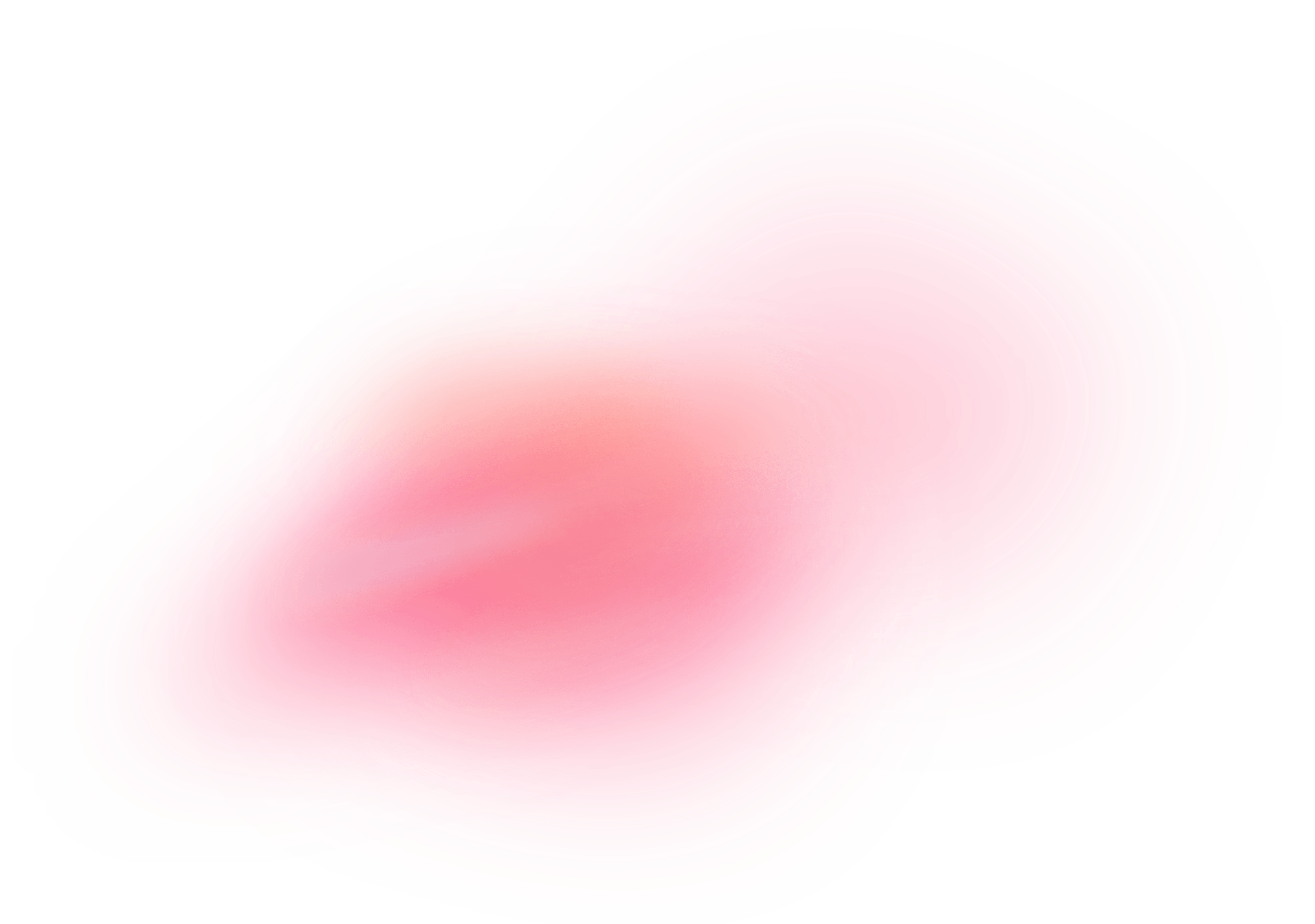