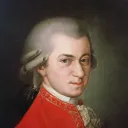
Hi!,
I would like to make a simple Appwrite database that updates emails and cities each time two text fields in my sveltekit website is filled. Very simple. However, it doesnt seem to function. here is the main code:
<script lang="ts">
export async function getIdeas() {
return await databases.listDocuments(
DATABASE_ID,
COLLECTION_ID,
// Use a query to show the latest ideas first
[Query.orderDesc('$createdAt')]
);
}
export async function addIdea(Email, CIty) {
await databases.createDocument(DATABASE_ID, COLLECTION_ID, ID.unique(), {
Email,
CIty
});
}
export async function deleteIdea(id) {
await databases.deleteDocument(DATABASE_ID, COLLECTION_ID, id);
}
async function handleSubmit() {
try {
if (!Email || !CIty) throw new Error("Please fill out both fields.");
// Add the idea with email and city to the database
await addIdea(Email, CIty);
// Clear input fields after successful submission
Email = '';
CIty = '';
alert("Thank you! You've been added to our notification list.");
} catch (error) {
console.log(error);
alert("Something went wrong while submitting your request. Please try again later.");
}
}
</script>
<main class="container px-6 py-8 mx-auto max-w-screen-md">
<h2 class="mb-5 text-xl font-bold leading-tight tracking-tighter md:text-2xl"> subscribe</h2>
<div class="grid grid-cols-2 gap-4 w-full">
<FlowbiteInput bind:value={Email} type="email" placeholder="Your Email Address" />
<FlowbiteInput bind:value={CIty} type="text" placeholder="Your City" />
</div>
<button on:click={handleSubmit} >Subscribe</button>
</main>
i simply follow the guide on the website, but it seems to not communicate with my database_id and collection id

Where are you initializing the appwrite client?
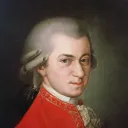
I am initializing it at src/lib/appwrite.js:
const client = new Client();
client
.setEndpoint("https://cloud.appwrite.io/v1")
.setProject("project id"); // Replace with your project ID
export const account = new Account(client);
export const databases = new Databases(client); ```

Are you having any errors thrown?
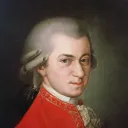
before running it does say "Parameter 'Email' implicitly has an 'any' type." in vs code.
I also get the self-made error:
""Something went wrong while submitting your request. Please try again later.""
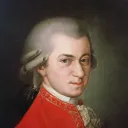
How can i do error handling? how can see wht goes wrong in appwrite?

You can wrap your appwrite code in a try catch block.

Could you in addIdea do something like
export async function addIdea(Email, CIty) {
console.log(DATABASE_ID);
console.log(COLLECTION_ID);
try {
await databases.createDocument(DATABASE_ID, COLLECTION_ID, ID.unique(), {
Email,
CIty
}
);
} catch (error: any) {
console.log(error);
}
}
Recommended threads
- Is my approach for deleting registered u...
A few weeks ago, I was advised not to use the registered users' id in my web app. Instead, I store the publicly viewable information such as username and email ...
- ❗[Help] Function stuck in "waiting" stat...
Hi Appwrite team 👋 I'm trying to contribute to Appwrite and followed the official setup instructions from the CONTRIBUTING.md guide to run the platform locall...
- Stuck in "deleting"
my parent element have relationship that doesnt exist and its stuck in "deleting", i cant delete it gives me error: Collection with the requested ID could not b...
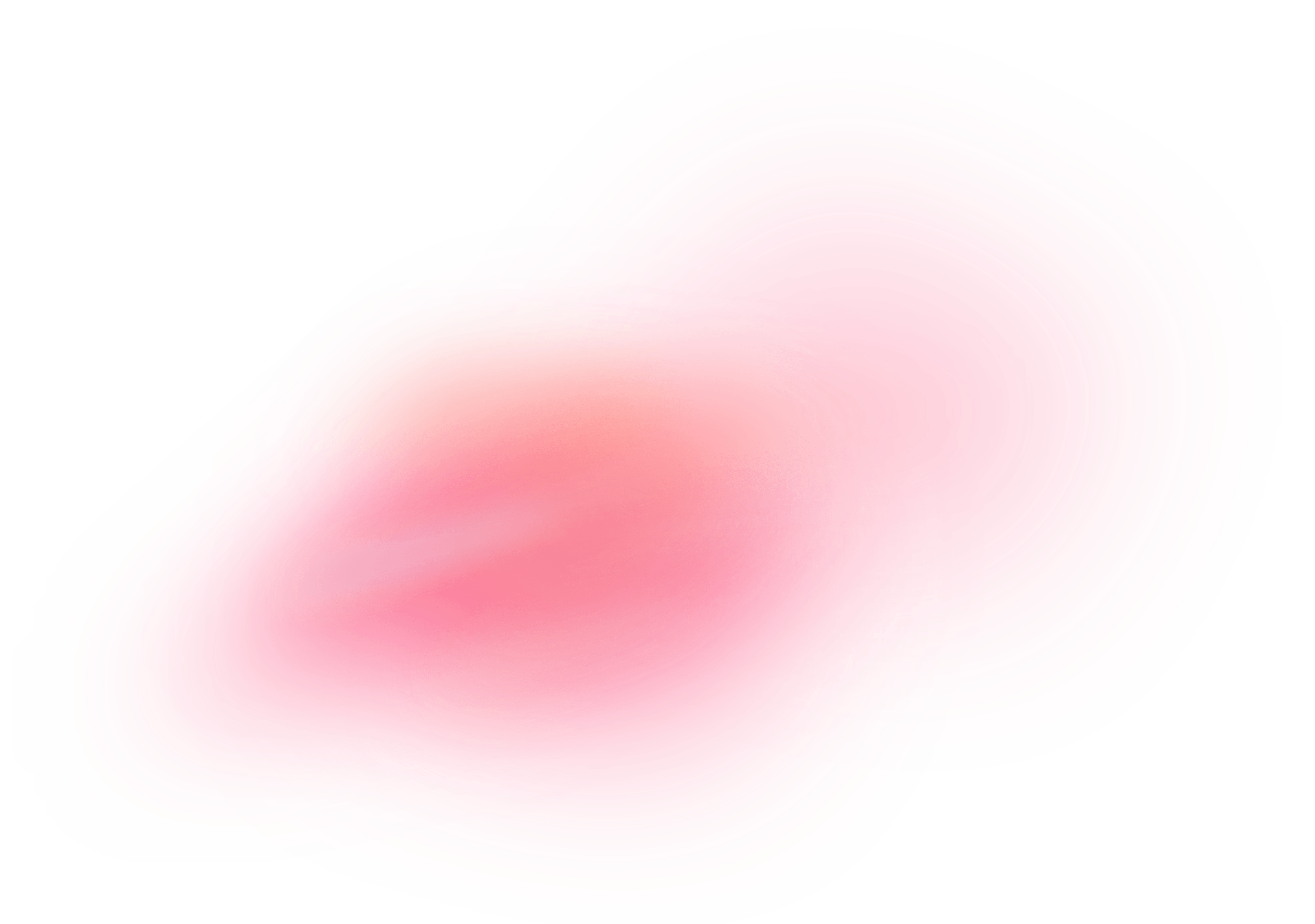