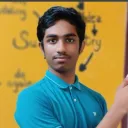
Hey can i create a function which uses whatsapp web js (which under the hood opens chromium browser via pupeteer) because getting this err:-
Error: Failed to launch the browser process! spawn /usr/local/server/src/function/node_modules/puppeteer/.local-chromium/linux-982053/chrome-linux/chrome ENOENT
TROUBLESHOOTING: https://github.com/puppeteer/puppeteer/blob/main/docs/troubleshooting.md
CODE:-
const qrcode = require("qrcode-terminal");
module.exports = async (context) => {
const whatsappclient = new Client({
authStrategy: new LocalAuth()
// puppeteer: { args: ['--no-sandbox', '--disable-setuid-sandbox'] }
});
whatsappclient.initialize();
whatsappclient.on("qr", (qr) => {
qrcode.generate(qr, { small: true });
context.log(qr);
});
whatsappclient.on("message", async (msg) => {
context.log("MESSAGE:- " + msg.body);
});
if (req.method === 'GET') {
if (req.path === '/message') {
const phoneNumber = req.query.phoneNumber;
const message = req.query.message;
const chatId = phoneNumber + "@c.us";
try {
// Wait for client to be ready before sending message
await new Promise((resolve) => whatsappclient.on("ready", resolve));
const response = await whatsappclient.sendMessage(chatId, message);
context.log("Message sent to:", phoneNumber, chatId);
context.log(response);
return res.send("Message sent successfully.");
} catch (err) {
context.error("Error sending message:", err);
return res.send("Error sending message.");
}
}
}
// Consider returning a placeholder response to indicate processing
// (optional, depending on your needs)
// return new Promise((resolve) => setTimeout(resolve, 1000));
};

You'll need to setup your build command to install chromium in your functions container, then set your whatsappclient/puppeteer to reference that new location of chromium.

I believe the function containers are alpine linux.

https://github.com/dishwasher-detergent/screenshot
Here is something I made that uses puppeteer, the two pages that would mean the most to you are the browser.ts
file, and the appwrite.json
file.

Check out https://discord.com/channels/564160730845151244/1215654642353176656, I have a working sample here, just ensure you have the correct environment variables and build settings along with the dependency declared in package.json and you should be good
Recommended threads
- Stuck at pinging the server to finish ad...
I'm not using the starter app and I'm not sure how to finish connecting my app to Appwrite.io. Is there a CURL command I can run to finish setup?
- 500 internal error
I get a 500 internal error when trying to access my database on appwrite cloud. Sometimes it would start working but this time it never corrects.
- Error getting session: AppwriteException...
I get this error `Error getting session: AppwriteException: User (role: guests) missing scope (account)` when running in prod. As soon as I try running my app o...
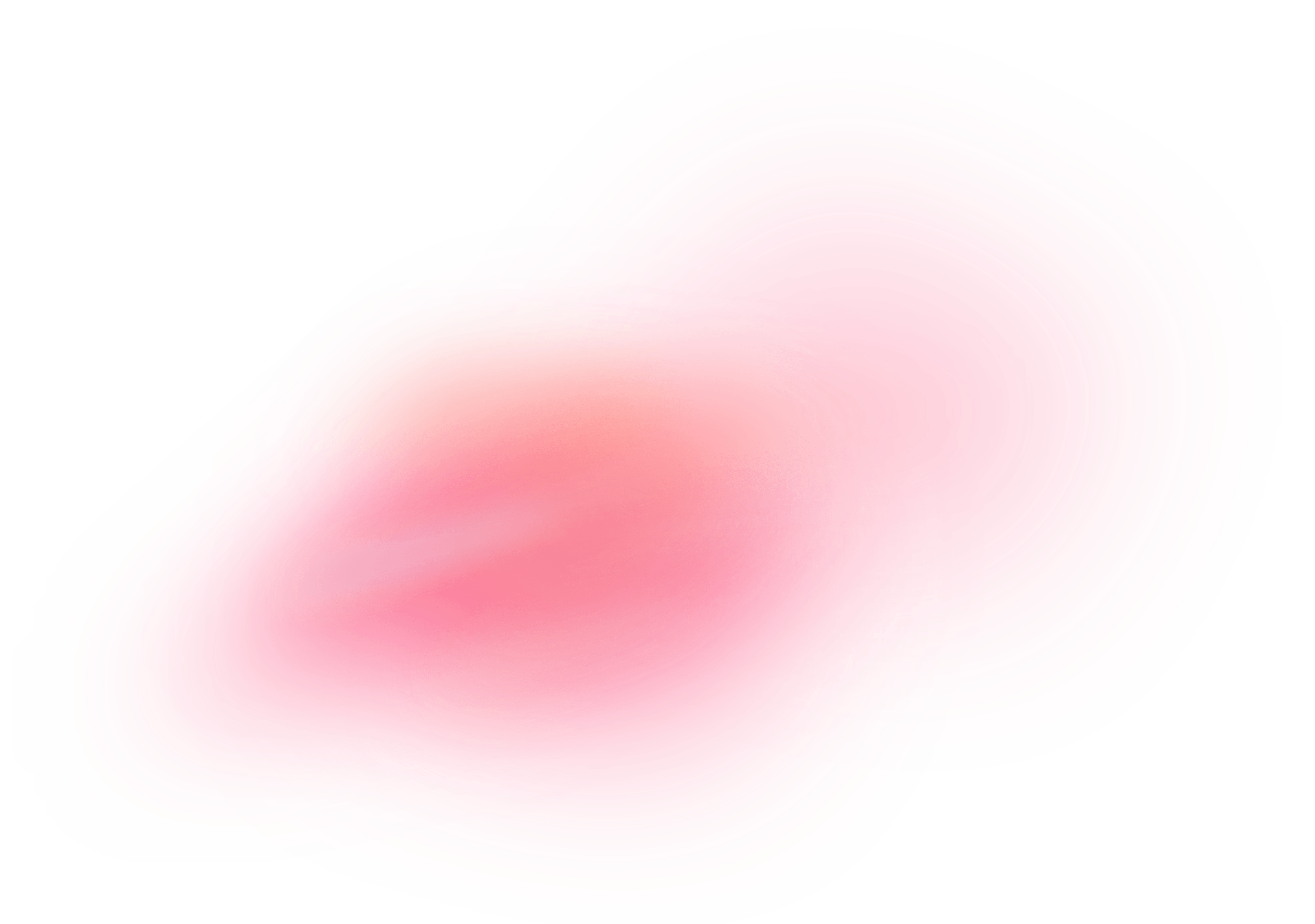