
i'm kinda new in python and i wanna know why i can't run this cloud function in my self-hosted appwrite cloud function. this is my code for image processing, it works on locale runs but i don't know how to make it work on cloud.
import cv2
import dlib
import numpy as np
import pytesseract
from PIL import Image
# Load the detector
detector = dlib.get_frontal_face_detector()
def extract_face(image_path, output_path, padding=35):
# Load the image
img = cv2.imread(image_path)
# Convert to grayscale
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# Use the detector to find faces
faces = detector(gray)
for i, face in enumerate(faces):
# Extract the face with some padding to make the image larger
x1, y1, x2, y2 = face.left() - padding, face.top() - padding, face.right() + padding, face.bottom() + padding
# Ensure the coordinates are within the image boundaries
x1, y1 = max(0, x1), max(0, y1)
x2, y2 = min(img.shape[1]-1, x2), min(img.shape[0]-1, y2)
# Crop the face out of the image
crop = img[y1:y2, x1:x2]
return crop
In the console in the appwrite self-host it logs
Docker Error: tar: short read

also part 2 of the code:
def passportProcessing(image_path):
# Reading the image
img = cv2.imread(image_path)
# Convert to gray scale
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# Adaptive thresholding
adaptive_thresh = cv2.adaptiveThreshold(gray, 255, cv2.ADAPTIVE_THRESH_GAUSSIAN_C,
cv2.THRESH_BINARY, 11, 2)
# Morphological operations to enhance text
kernel = np.ones((1, 1), np.uint8)
img_morph = cv2.morphologyEx(adaptive_thresh, cv2.MORPH_OPEN, kernel)
img_morph = cv2.morphologyEx(img_morph, cv2.MORPH_CLOSE, kernel)
if img is not None:
text = pytesseract.image_to_string(img_morph,lang='eng+ara')
texts = text.split('\n')
# Applying the cleaning function to each text and filtering out single character or empty entries
filtered_texts_corrected = [clean_text(text) for text in texts if text.strip() and len(text.strip()) > 1]
return filtered_texts_corrected
else:
print("Image not loaded correctly. Check the file path and file format: {image_path}")
def clean_text(text):
return ''.join(char for char in text if char not in ('\u200f', '\u200e'))
def main(req, res):
client = Client()
print('Hello from Appwrite Function!')
print("Variables",req.variables)
# You can remove services you don't use
database = Databases(client)
users = Users(client)
if not req.variables.get('APPWRITE_FUNCTION_ENDPOINT') or not req.variables.get('APPWRITE_FUNCTION_API_KEY'):
print('Environment variables are not set. Function cannot use Appwrite SDK.')
else:
(
client
.set_endpoint(req.variables.get('APPWRITE_FUNCTION_ENDPOINT', None))
.set_project(req.variables.get('APPWRITE_FUNCTION_PROJECT_ID', None))
.set_key(req.variables.get('APPWRITE_FUNCTION_API_KEY', None))
.set_self_signed(True)
)
return res.json({
"areDevelopersAwesome": True,
})
Recommended threads
- Cloud Functions Executions partially dow...
Yesterday we reported a wide Function-Downtime. Today we have the same - just not wide-spread across all functions. DEV is down - Prod still working. Its the s...
- Unable to Access Project Dashboard After...
I’m currently working on a team project using Appwrite and ran into an issue accessing my project dashboard. After clearing cookies and cache, I attempted to lo...
- Appwrite Functions Generally Down?
We are getting customer support requests, that our service is down. We tried to pinpoint it, and we can't see any logs of any appwrite cloud function in the pas...
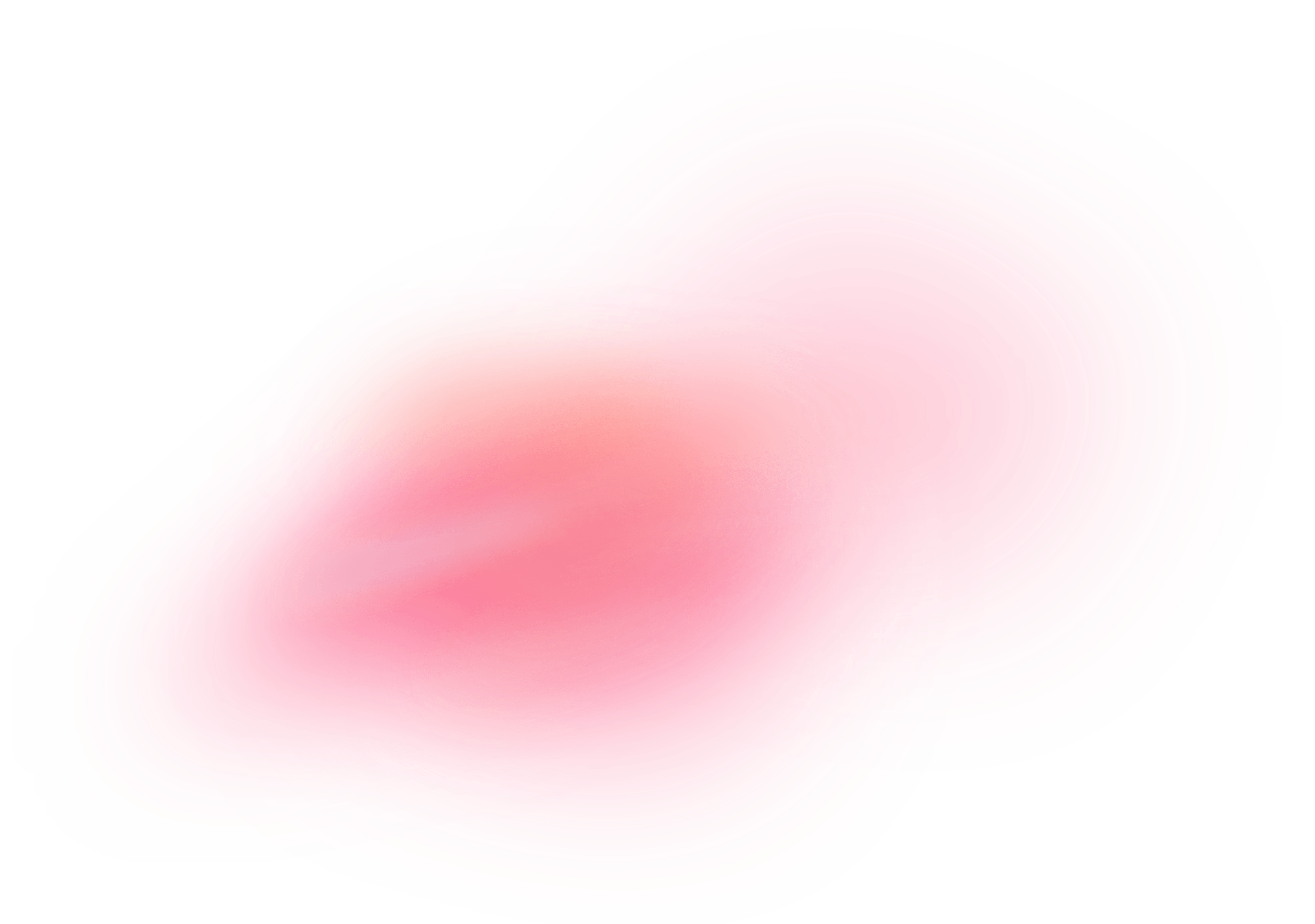