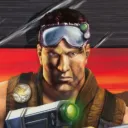
Hi! Is there a way to dynamically build the listDocuments query? In the app, the "base" query I have is
Query.equal('category', 'expenses'),
Query.greaterThan('amount', 100),
];
final response = await databases.listDocuments(
'<DATABASE_ID>',
'<COLLECTION_ID>',
query,
);
I would like to have a checkbox (or a switch) for a select date and then have this date added to the query parameters. How should I do it?
TIA
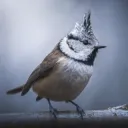
What about adding/removing the Query for the date to the base query
list on checkbox toggle?
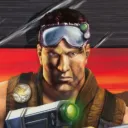
Yes, that's my idea, but how to I add/remove to the query
list? What type is Query.equal('date',<date>)
?
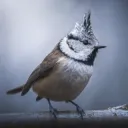
How about just doing
//checkbox enabled
query.add(Query.equal('category', 'expenses')); //This will be the new query to add
//checkbox disabled
query.removeLast();
This method will require a bit of checks to make sure you're not removing any of the base queries. You could use a map for the queries and remove by the query's key. Example
// Query.method() generates Strings
final Map<String, String> query = {
'category': Query.equal('category', 'expenses'),
'amount': Query.greaterThan('amount', 100),
};
//checkbox toggle on
query.addAll({
'date': Query.equal('date', ['2024-2-15'])
});
//checkbox toggle off
query.remove('date');
//To get queries
query.values.toList();
Recommended threads
- OAuth2 with IPhone When users enables "H...
I am using Appwrite OAuth2 to authenticate users in my app (Flutter) Normally, I am using the user's email for the authentication, when he first registers , and...
- Still showing this after complete my pay...
This project is in readonly mode. Please contact the organization admin for details.
- flutter_web_auth_2 needs to be updated!
The appwrite SDK is using an old version of flutter_web_auth_2 which I beleive is now deprecated, or atleast, isn't working for me. iOS, Linux, Web, Windows stu...
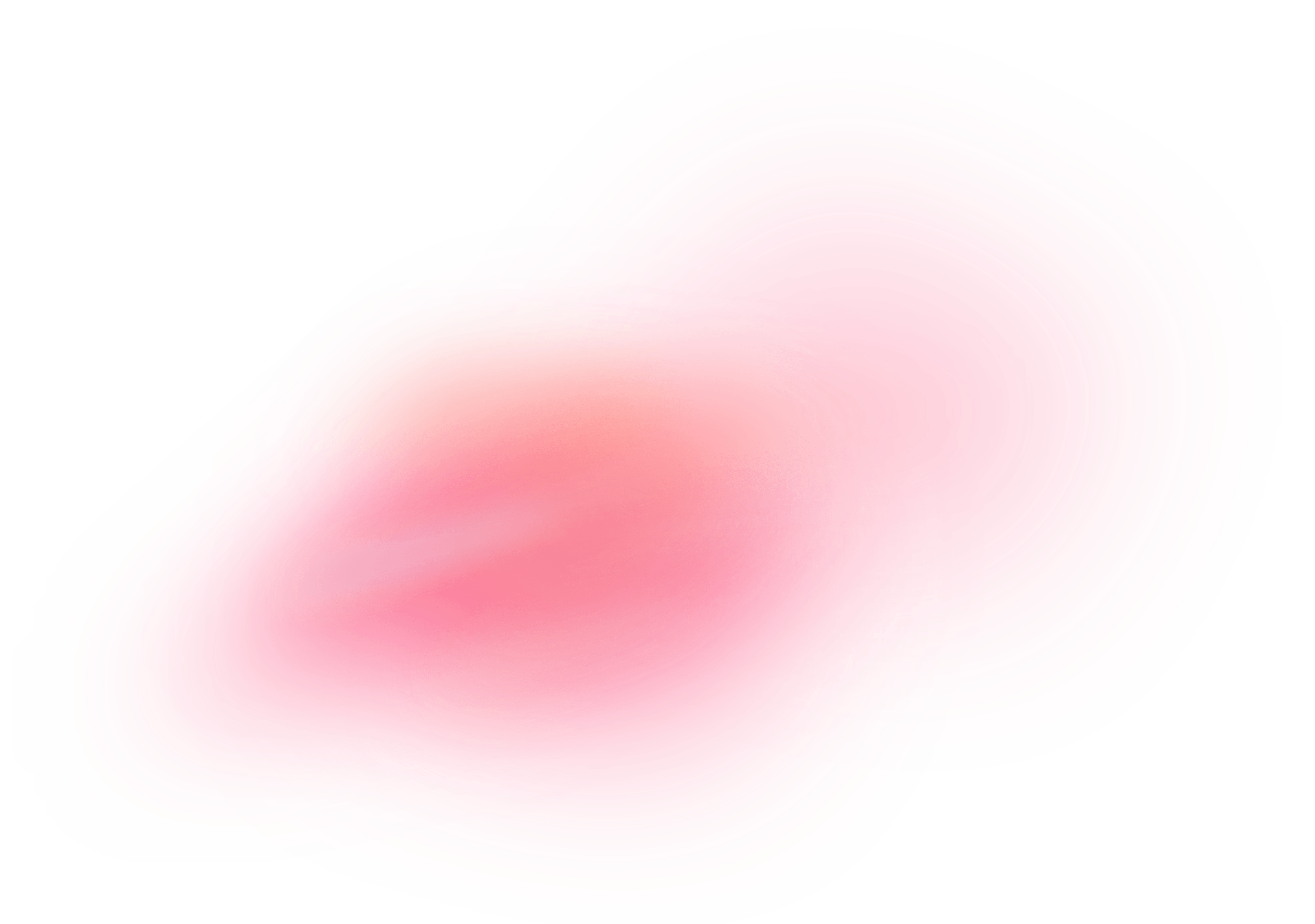