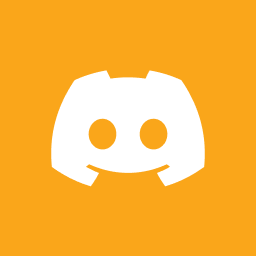
This is my nodejs, cloud function:
import { Client, Databases, ID, Permission, Role } from 'node-appwrite';
export default async ({ req, res, log, error }) => {
const client = new Client()
.setEndpoint('https://cloud.appwrite.io/v1')
.setProject(process.env.APPWRITE_PROJECT_ID)
.setKey(process.env.APPWRITE_API_KEY);
const databases = new Databases(client);
// You can log messages to the console
log('Hello, Logs!');
// If something goes wrong, log an error
error('Hello, Errors!');
// Parse the request body to get IDINPUT
const { IDINPUT } = JSON.parse(req.body);
log(IDINPUT);
if (req.method === 'GET') {
// Send a response with the res object helpers
return res.send(`hello ${process.env.DOG_NAME}`);
} else if (req.method === 'POST') {
try {
/// Create a document in the specified collection
const response = await databases.createDocument(
process.env.APPWRITE_DATABASE_ID,
process.env.APPWRITE_USERINFO_ID,
ID.unique(),
{surname: 'dibba'},
[
Permission.write(Role.user(IDINPUT)),
Permission.read(Role.user(IDINPUT)),
]
);
console.log(response);
return res.send('document created');
} catch (err) {
console.log(err);
return res.send('document not created, internal error');
}
}
return res.json({
motto: 'Build like a team of hundreds_',
learn: 'https://appwrite.io/docs',
connect: 'https://appwrite.io/discord',
getInspired: 'https://builtwith.appwrite.io',
});
};
I know I am parsing IDINPUT correctly because in the log I see the correct user id.
I call this function in my flutter app, where I am logged in with an active email session.
I have document level permission active on my collection. I have set that any user can just create.
My apikey has 2 scopes:
- read documents
- write documents
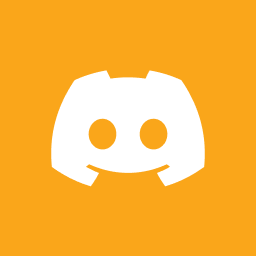
This is the full error:
Hello, Logs!
65cfcc64a2e0faf8ffb8
----------------------------------------------------------------------------
Unsupported logs detected. Use context.log() or context.error() for logging.
----------------------------------------------------------------------------
AppwriteException [Error]: Permissions must be one of: (any, guests)
at Client.call (/usr/local/server/src/function/node_modules/node-appwrite/lib/client.js:172:31)
at process.processTicksAndRejections (node:internal/process/task_queues:95:5)
at async Databases.createDocument (/usr/local/server/src/function/node_modules/node-appwrite/lib/services/databases.js:1666:16)
at async Module.default (file:///usr/local/server/src/function/src/main.js:28:24)
at async execute (/usr/local/server/src/server.js:141:22)
at async /usr/local/server/src/server.js:158:13 {
code: 401,
type: 'user_unauthorized',
response: {
message: 'Permissions must be one of: (any, guests)',
code: 401,
type: 'user_unauthorized',
version: '0.12.89'
}
}
----------------------------------------------------------------------------
Recommended threads
- Is Quick Start for function creation wor...
I am trying to create a Node.js function using the Quick Start feature. It fails and tells me that it could not locate the package.json file. Isn't Quick Start ...
- Connecting server functions to GitHub re...
The project I am working in has recently moved organizations on Appwrite. The same is true for the repo on GitHub, which as moved from a private user to a organ...
- Missing C++ libstdc library in Python fu...
I have a function running Python 3.12 which suddenly started dumping errors (as of today; it worked yesterday). I hadn't changed any code so I found this odd, b...
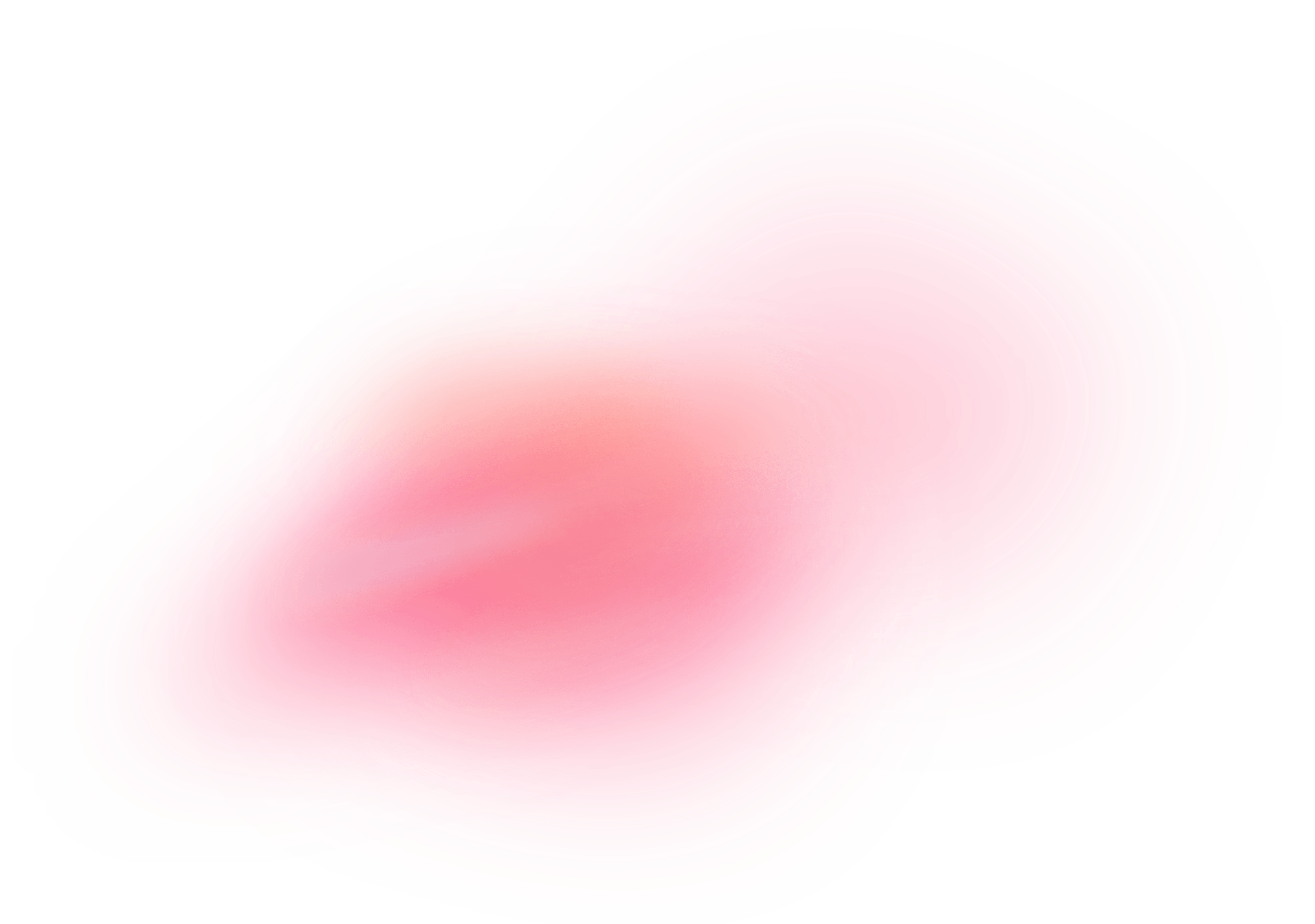