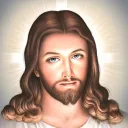
Hi, this a function in dart that subtract a 1 from the LMMS attribute for 6000 documents then update every document with the new subtracted value, the issue is, it takes so long (11 minutes)😭
var response = await database.listDocuments( databaseId: databaseId, collectionId: collectionId, queries: [Query.limit(6000)]); var documents = response.documents;
try { for (var doc = 0; doc < documents.length; doc++) { String documentId = documents[doc].$id; final data = documents[doc].data;
// Subtract 1 from LMMS first
int LMMS = data['LMMS'] - 1;
// Update the document with the initially subtracted LMMS
await database.updateDocument(
databaseId: databaseId,
collectionId: collectionId,
documentId: documentId,
data: {'LMMS': LMMS});
}
return context.res.send(200);
} catch (e) { print('Error updating document: $e'); return context.res.send(401); }
Could any one help me make it faster.

This is a function I've used in the past to update a lot of existing documents, you might see if it helps out any.
import { Client, Databases, Query } from 'node-appwrite';
export default async ({ req, res, log, error }) => {
const client = new Client()
.setEndpoint(process.env.APPWRITE_FUNCTION_ENDPOINT)
.setProject(process.env.APPWRITE_FUNCTION_PROJECT_ID)
.setKey(process.env.APPWRITE_FUNCTION_API_KEY);
const database = new Databases(client);
let response;
const queries = [Query.limit(25), Query.select("$id")];
let cursor = null;
do {
const newQueries = [...queries];
if (cursor) {
newQueries.push(Query.cursorAfter(cursor));
}
response = await database.listDocuments(
DATABASE_ID,
COLLECTION_ID,
newQueries
);
cursor = response.documents[response.documents.length - 1].$id;
await Promise.all(
response.documents.map((document) => {
database.updateDocument(
DATABASE_ID,
COLLECTION_ID,
DOCUMENT_ID,
data
)
})
);
} while (response.documents.length >= 25);
return res.send('Complete!');
};
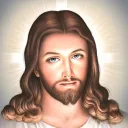

How often are you needing to run this function? Is it a one and done or ?
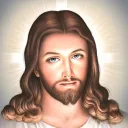
It will run a lot
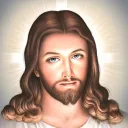
That's why I'm frustrated it takes 11 minutes to complete for only 6000 document
Recommended threads
- Cannot find module failure
Sorry, Newbe question here. I just installed Appwrite and am trying to install my first Function an am having absolutely no luck what-so-ever getting this done...
- Problems with adding my custom domain
- Can't push functions when self-hosting o...
Hello, I'm a bit new to appwrite functions and recently hosted a fresh 1.7.4 on my portainer setup. Tried to create a new function and when trying to push it I ...
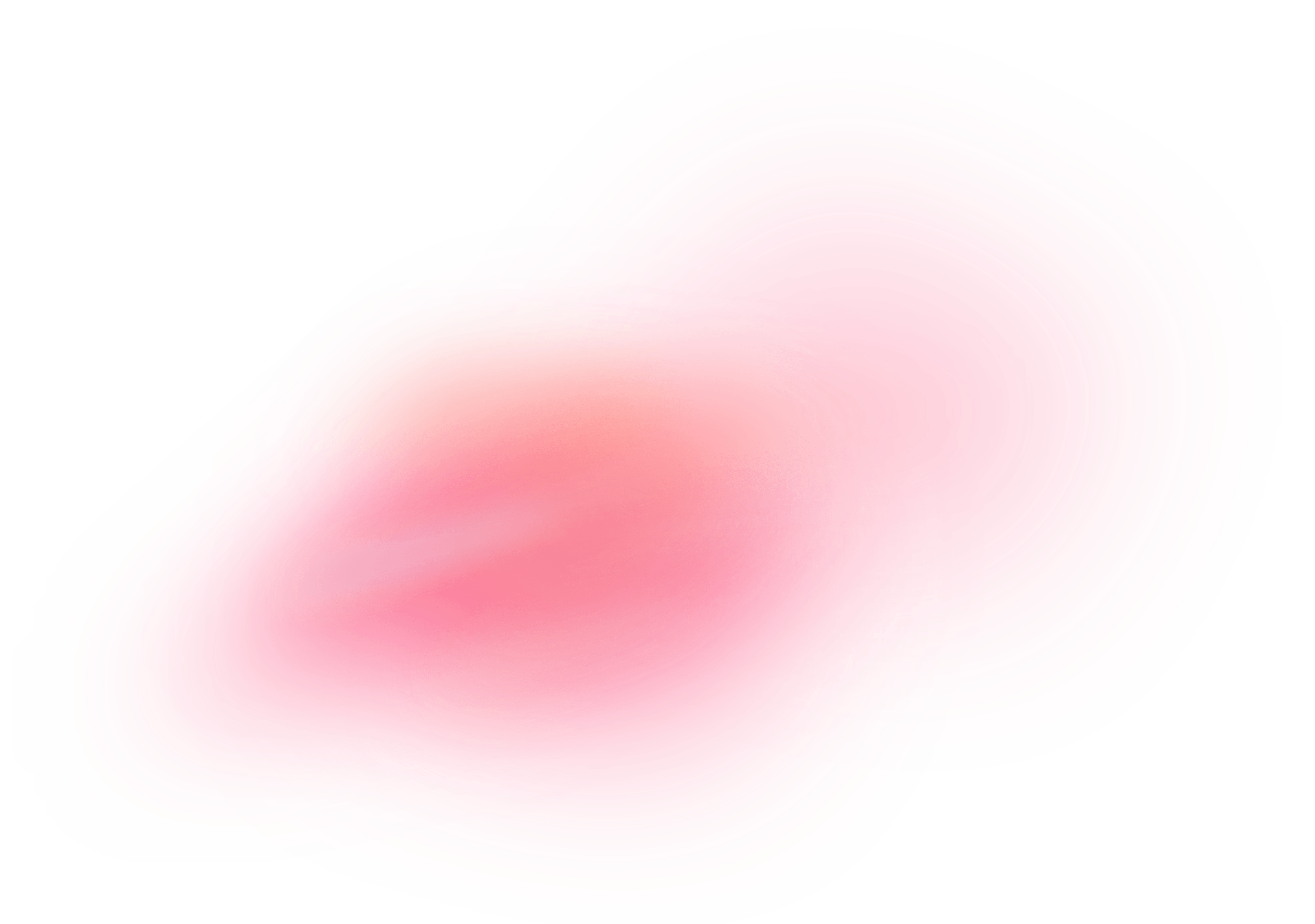