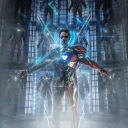
I am facing this issue in my project and I am totally blank as to what to do exactly. Please can you guide me because I am quite new to this things. Thanks
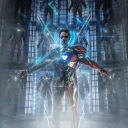
I'll show you my code for login/signup.
// start with appwrite auth service import conf from "../conf/conf.js" import { Client, Account, ID } from "appwrite";
export class AuthService { client = new Client(); account;
constructor() {
this.client
.setEndpoint(conf.appwriteUrl)
.setProject(conf.appwriteProjectId)
// .setKey(conf.appwriteApiKey)
this.account = new Account(this.client)
}
async createAccount({ email, password, name }) {
try {
const userAccount = await this.account.create(ID.unique(), email, password, name)
if (userAccount) {
return this.login({ email, password })
} else {
return userAccount
}
} catch (error) {
throw error
}
}
async login({ email, password }) {
try {
return await this.account.createEmailSession(email, password)
} catch (error) {
throw error
}
}
async getCurrentUser() {
try {
return await this.account.get()
} catch (error) {
console.log("Appwrite service :: getCurrentUser() :: ", error);
}
return null
}
async logout() {
try {
await this.account.deleteSessions()
} catch (error) {
console.log("Appwrite service :: logout() :: ", error);
}
}
// async updateUserRoles(userId, role, scope) {
// try {
// // Use the appropriate method from the Appwrite SDK to update user roles
// await this.account.updateUserRoles(userId, role = "guests", scope = "account");
// } catch (error) {
// console.log("Error updating user roles:", error);
// throw error;
// }
// }
}
const authService = new AuthService()
export default authService
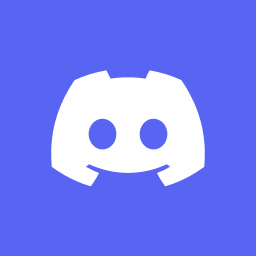
If this is client side, don't set api key
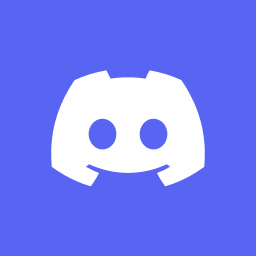
The code looks correct. You're not getting the current user because it's logged out
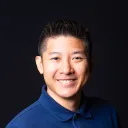
FYI, it's best to wrap code in backticks to format a bit nicer. You can use 1 backtick for inline code (https://www.markdownguide.org/basic-syntax/#code) and 3 backticks for multiline code (https://www.markdownguide.org/extended-syntax/#syntax-highlighting.
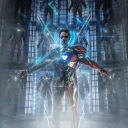
I did try to login through my interface but my login signup buttons aren't responding because of this error
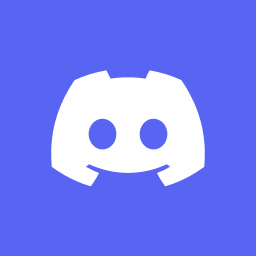
What's te logic performed once clicked the button?
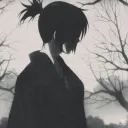
You should check if the onClick event is occurring or not . Also make sure that the routing is correct since I missed Outlet in my App.jsx Once you are Signed and logged in you will get Current User
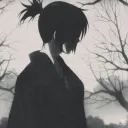
Just an Update Check for CORS error and add your platform in your project
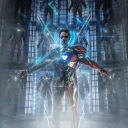
i did add the cors and added the platform
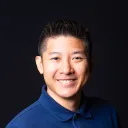
Please share the request headers for the failed request
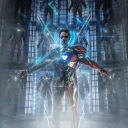
what do you mean by request headers?
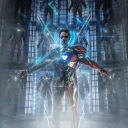
The problem which I am facing is that my Login/signup buttons are not working properly, so that's why I am not able to enter any users and thats the reason why I am getting this error.
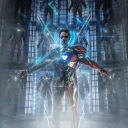
But the now I am not able to fix my bug.
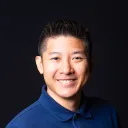
switch to the network tab in the browser dev tools and look for the failed request. when you look at the details, you should be able to see the request headers
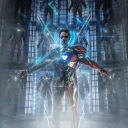
I can't see anything in my network tab
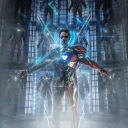
It's all empty in there
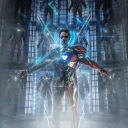
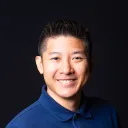
refresh the page and run through the process again
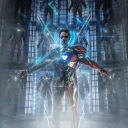
yes now I can see
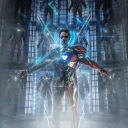
I'll send it right away
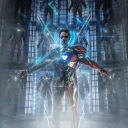
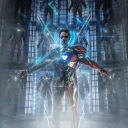
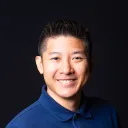
so is this before logging in?
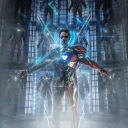
yes
Recommended threads
- Collection Permission issue
I am facing issue in my Pro account. "Add" button is disabled while adding permission in DB collection settings.
- Opened my website after long time and Ba...
I built a website around a year back and and used appwrite for making the backend. At that time the website was working fine but now when i open it the images a...
- Is it possible to cancel an ongoing file...
When uploading a file to storage, is there a way to cancel the upload in progress so the file is not saved or partially stored?
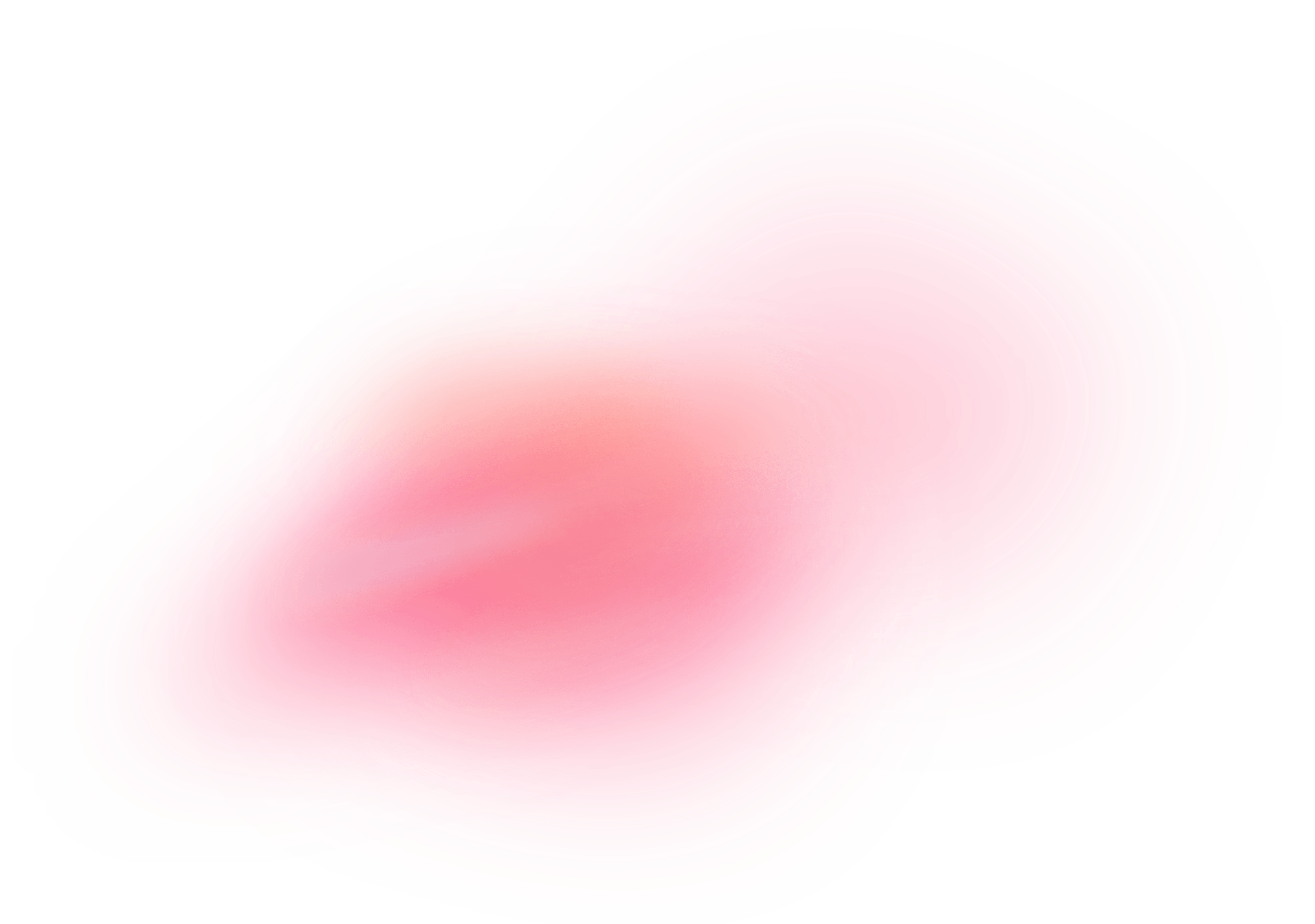