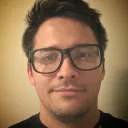
Hello, I'm coming from firebase and am excited to experiment with you guys. I am curious how my react app can create a user with only the following
import { Client, Account, ID } from "appwrite";
const client = new Client()
.setEndpoint('https://cloud.appwrite.io/v1') // Your API Endpoint
.setProject('<PROJECT_ID>'); // Your project ID
const account = new Account(client);
const promise = account.create('[USER_ID]', 'email@example.com', '');
promise.then(function (response) {
console.log(response); // Success
}, function (error) {
console.log(error); // Failure
});
I would expect I would need to set an api key or secret as an env var in my vite react app, for example via VITE_APPWRITE_API_KEY.
If someone sees my code can they write the above code and execute actions on my stuff?
Or is the "protection" coming from the Hostname setting when I add a platform?
Or should there be verbiage in the docs saying that the api endpoint and project id should be secrets?

Key Points:
1.Never expose your API key in client-side code. It grants full access to your Appwrite project. 2.Use server-side SDKs to handle user creation and actions that require authentication. 3.Store your API key securely on the server, protected from public access. 4.The Hostname setting is primarily for customizing API endpoint URLs, not for security.
Recommended Setup:
- Server-side (e.g., Node.js):
- Install the Appwrite Node.js SDK:
npm install appwrite
- Create a server-side route to handle user creation:
TypeScriptconst Appwrite = require('appwrite'); const client = new Appwrite(); client .setEndpoint('https://cloud.appwrite.io/v1') // Your API endpoint .setProject('[YOUR_PROJECT_ID]') .setKey('[YOUR_API_KEY]'); // Securely stored API key app.post('/create-user', async (req, res) => { const { email } = req.body; const account = new Appwrite.Account(client); const response = await account.create('unique()', email, ''); res.json(response); });
- Install the Appwrite Node.js SDK:
- React app:
- Make a POST request to your server-side route when creating a user:
TypeScriptfetch('/create-user', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({ email: 'user@example.com' }), }) .then(response => response.json()) .then(data => console.log('User created:', data)) .catch(error => console.error('Error:', error));
Additional Security Measures:
Input validation: Sanitize user input to prevent code injection attacks. Error handling: Gracefully handle errors and avoid exposing sensitive information. Regular updates: Keep your Appwrite server and SDKs up-to-date with security patches.
Recommended threads
- Custom domain issue
Hello following another post I'm creating dedicated post according to my project ID: 67ffbd800010958ae104 I deployed for debug my React Native app in web, chrom...
- Appwrite DNS Record Invalid on 123reg
So I go to the project settings and add in my domain name. Then when I add the CNAME record to 123reg it says that ''Record data is invalid'' As seen in the s...
- Image preview error
cannot see the image preview ..please help me fix this issue
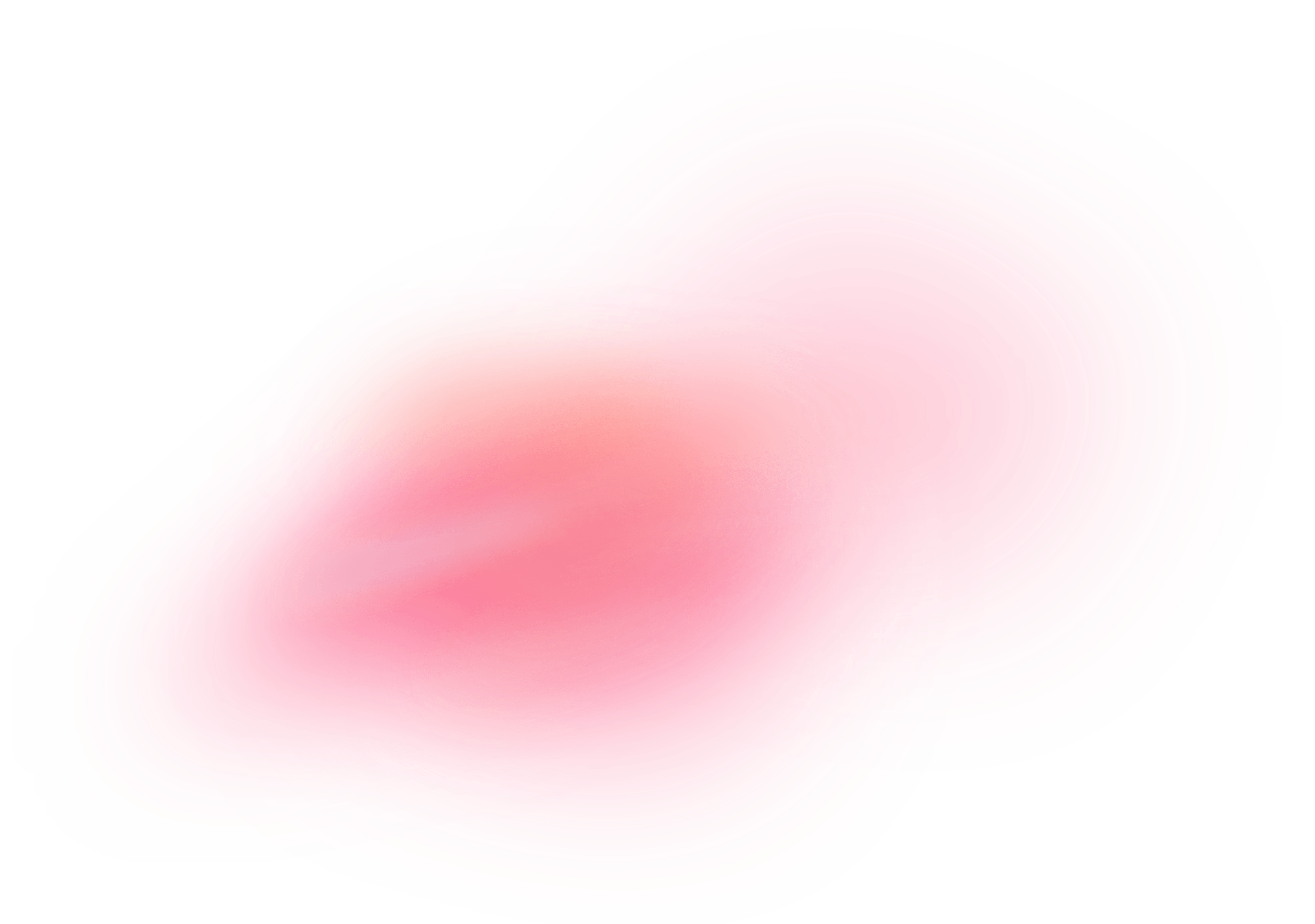