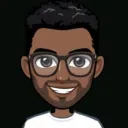
Hi Guys, Could someone please help with the below issue? I am keeping getting the following error, while creating a post and trying to save it on my Appwrite DB. User auth and creation works perfect, user is saved on the DB:
"api.ts:117
POST https://cloud.appwrite.io/v1/databases/65955c1f63e8af139596/collections/65955c743b49830747b8/documents 500 (Internal Server Error)
Error creating post document: AppwriteException: Server Error at Client.<anonymous> (http://localhost:5173/node_modules/.vite/deps/appwrite.js?v=34b59556:850:17) at Generator.next (<anonymous>) at fulfilled (http://localhost:5173/node_modules/.vite/deps/appwrite.js?v=34b59556:488:24)"
my console.log (client side) is showing that the post has been created but is not getting saved to the DB.
Here are my code snippets - React+Vite:
1 - from PostForm.tsx
type PostFormProps = { post?: Models.Document; }
const PostForm = ({ post }: PostFormProps) => {
const { mutateAsync: createPost, isPending: isLoadingCreate } = useCreatePost();
const { user } = useUserContext();
const { toast } = useToast();
const navigate = useNavigate();
// 1. Define your form.
const form = useForm<z.infer<typeof PostValidation>>({ resolver: zodResolver(PostValidation), defaultValues: { caption: post ? post?.caption : "", file: [], location: post ? post?.location : "", tags: post ? post.tags.join(",") : "", }, })
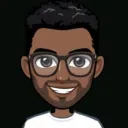
// 2. Define a submit handler. async function onSubmit(value: z.infer<typeof PostValidation>) { // Do something with the form values. // ✅ This will be type-safe and validated. const newPost = await createPost({ ...value, userId: user.id, })
if(!newPost) {
toast({
title: 'Please try again'
})
}
navigate('/')
console.log(value)
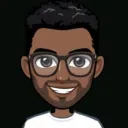
2 - From queriesAndMutations.ts:
export const useCreatePost = () => { const queryClient = useQueryClient(); return useMutation({ mutationFn: (post: INewPost) => createPost(post), onSuccess: () => { queryClient.invalidateQueries({ queryKey: [QUERY_KEYS.GET_RECENT_POSTS], }); }, }); };
3 - from api.ts:
export async function createPost(post: INewPost) { try { // Upload file to appwrite storage const uploadedFile = await uploadFile(post.file[0]);
if (!uploadedFile) throw Error;
// Get file url
const fileUrl = getFilePreview(uploadedFile.$id);
if (!fileUrl) {
await deleteFile(uploadedFile.$id);
throw Error;
}
// Convert tags into array
const tags = post.tags?.replace(/ /g, "").split(",") || [];
// Save the post in DB
const newPost = await databases.createDocument(
appwriteConfig.databaseId,
appwriteConfig.postCollectionId,
ID.unique(),
{
creator: post.userId,
// likes: likes,
caption: post.caption,
imageUrl: fileUrl,
imageId: uploadedFile.$id,
location: post.location,
tags: tags,
}
);
if (!newPost) {
await deleteFile(uploadedFile.$id);
// throw Error;
throw new Error("Failed to create post document.");
}
return newPost;
} catch (error) {
console.error("Error creating post document:", error);
}
}
// ============================== UPLOAD FILE export async function uploadFile(file: File) { try { const uploadedFile = await storage.createFile( appwriteConfig.storageId, ID.unique(), file );
return uploadedFile;
} catch (error) {
console.log(error);
}
}
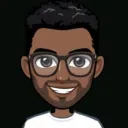
// ============================== GET FILE URL export function getFilePreview(fileId: string) { try { const fileUrl = storage.getFilePreview( appwriteConfig.storageId, fileId, 2000, 2000, "top", 100, );
if (!fileUrl) throw Error;
return fileUrl;
} catch (error) {
console.log(error);
}
}
// ============================== DELETE FILE export async function deleteFile(fileId: string) { try { await storage.deleteFile(appwriteConfig.storageId, fileId);
return { status: "ok" };
} catch (error) {
console.log(error);
}
}
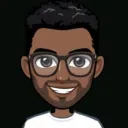
Sorry for the multiple messages, but I wanted to provide the different parts of the code
Recommended threads
- Migration to new region: not so good.
We attempted to do a migration to NYC... and things didn't go well. We followed the steps (exactly) as shown in the video, updated our app with the new endpoint...
- Image Loading issue in Snapgram project
Hello community, I have a issue with the Image Loading I made a Project from youtube of Snapgram, it worked perfectly 8 months ago, but now the images aren't l...
- No Targets Created For Email OTP Users
I use OTP for my users. All emails are verified. No one has their email as a target. What am I doing wrong? The documentation says the targets should be created...
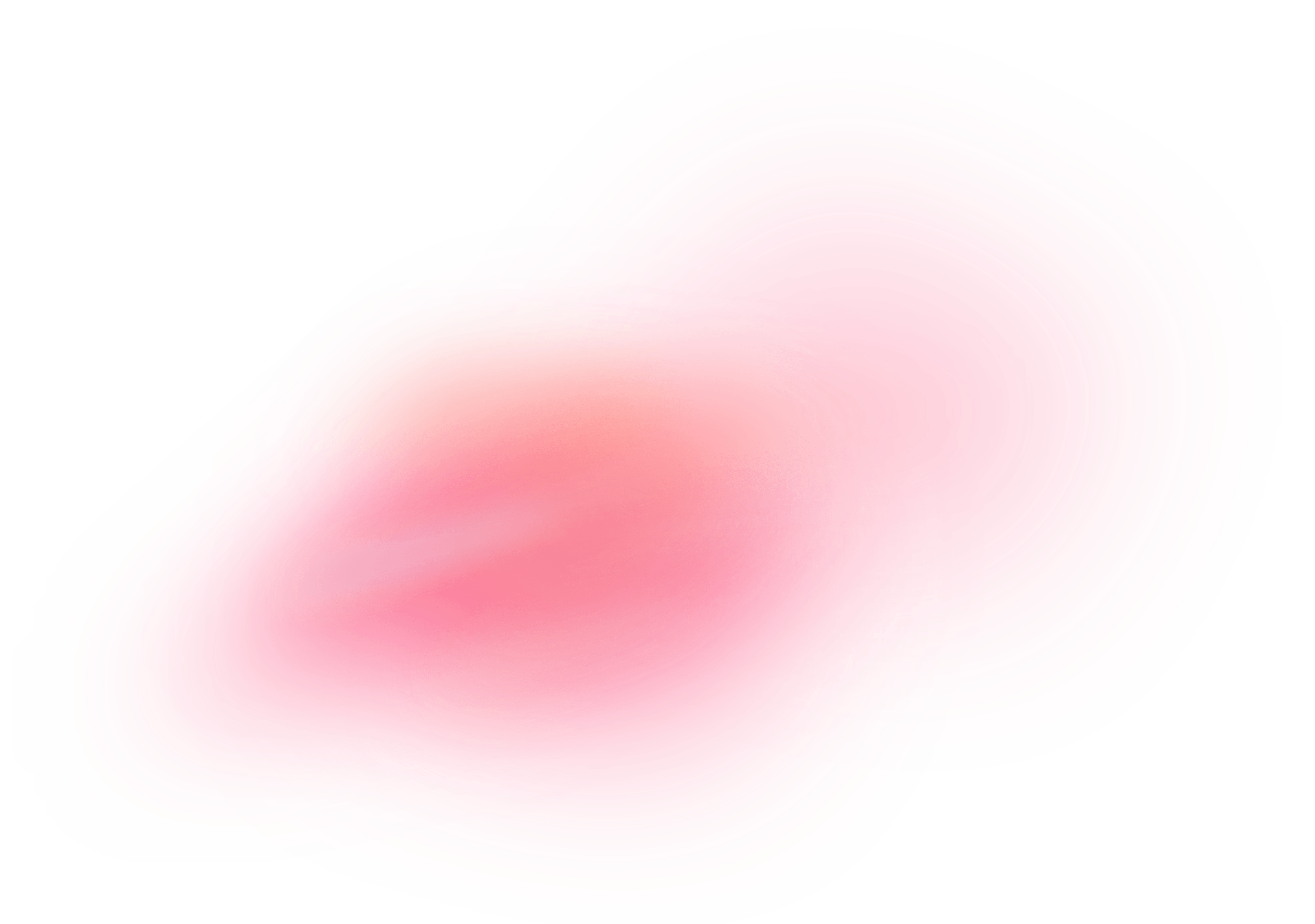