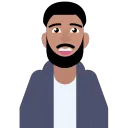
Hey everyone, i'm running into an issue when storing user data in a database collection. Currently, the function does not run or store any data in the collection. The way it works right now is a user first created an account, so I run the "createUser" function, and then in a subsequent webpage they select up to a total of 21 topics, which are added in a database collection associated with their user id. The function I run for this is "createUserTopics". Is there something wrong with the way I am handling my code?
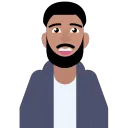
// Registering the User (Sign Up)
Future<String> createUser(String name, String email, String password, String role) async {
try {
final user = await account.create(
userId: ID.unique(),
email: email,
password: password,
name: name);
print("New User created");
print("Working so far");
print("Created user ID: ${user.$id}");
// storing the user role in the database after successful registration
await databases.createDocument(
databaseId: 'xxx',
collectionId: 'xxx',
documentId: user.$id,
data: {
'userid': user.$id,
'role': role
});
// Automatically login user after account creation
try {
Map<String, dynamic> loginResult = await loginUser(email, password);
bool loginSuccess = loginResult['success'];
if (loginSuccess) {
print("User automatically logged in after registration");
} else {
print("Failed to automatically log in");
}
} catch (e) {
// Handle any exceptions thrown by loginUser
if (e is AppwriteException) {
print('Failed to automatically log in: ${e.message}');
} else {
print('An unknown error occurred while trying to log in: $e');
}
}
return user.$id;
} on AppwriteException catch(e) {
return e.message.toString();
}
}
// Function to update user preferences with selected topics
Future<String> createUserTopics(String userId, List<String> topics) async {
try {
// Update the document with the list of topics
await databases.createDocument(
databaseId: 'xxx',
collectionId: 'xxx',
documentId: userId,
data: {
'topics': topics
},
);
print("User preferences updated successfully");
return "User preferences updated successfully";
} catch (e) {
print("Error updating user preferences: $e");
return "Error updating user preferences";
}
}
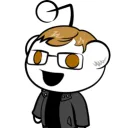
What's the error you're getting, and where?

You should also check whether the right permissions are granted
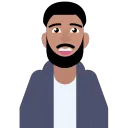
I'm not getting any error at all, which is why i'm a bit confused
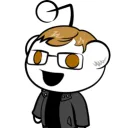
Does the user get created?
Recommended threads
- Appwrite Sites - getaddrinfo ENOTFOUND
Has anyone had a problem having appwrite on a subdomain in the project custom domains? We have an issue with the Appwrite Sites. Sometimes the internal node:dns...
- Incremental/Updates based on OG value wh...
I've set up my own SDK accessed as below ```lua DB["userinfo"]["bankaccounts"]:update({ method = "equal", attribute = "userid", values =...
- Problem while creating new jwt token in ...
I have a component that creates new jwt every 14sec but it seems to not be working , also not sure if that is the best way to do it
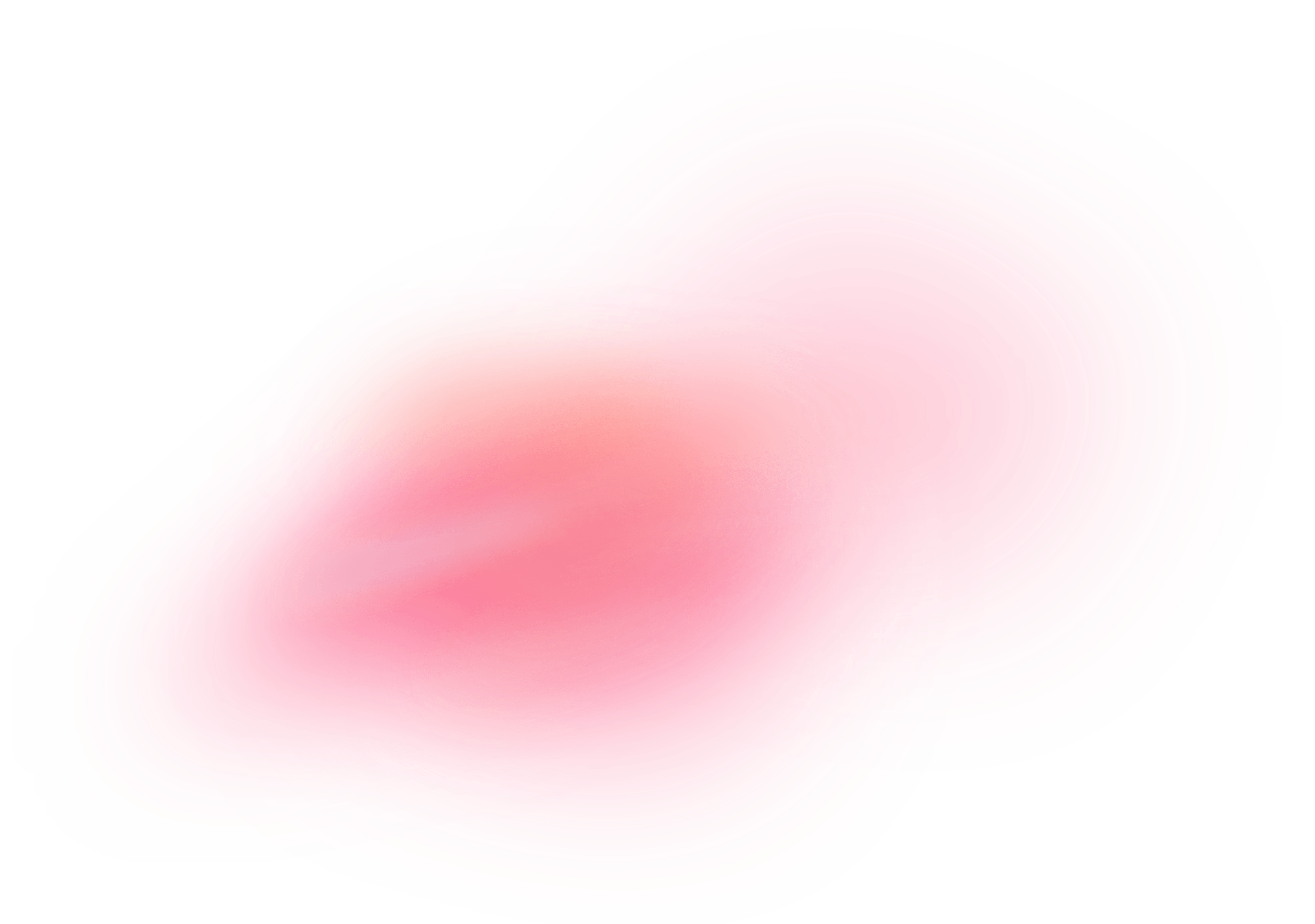