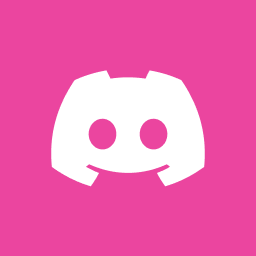
I have a function that checks for authentication in the following way
- Initialize the client using
jwt
passed by the user
const client = new Client()
.setEndpoint(process.env.APPWRITE_API_ENDPOINT)
.setProject(process.env.APPWRITE_FUNCTION_PROJECT_ID)
.setJWT(req.headers['x-appwrite-user-jwt'])
- Authenticate function to check if a user is a valid one
import { Account } from "node-appwrite"
export async function authenticate(client, log, error) {
log("Initializing Account")
const account = new Account(client)
let user = null
try {
log("Getting account")
user = await account.get()
log(user)
if (!user.email)
throw Error("User does not have email")
log(user.$id, 'authenticated')
} catch (e) {
user = null
error(e.toString())
}
return user
}
However I get the following logs,
Initializing Account
Getting account
And the following error logs,
Error: connect ECONNREFUSED 127.0.0.1:80
It probably means the Accounts API is not working in node-appwrite
, Can someone please help me out here?
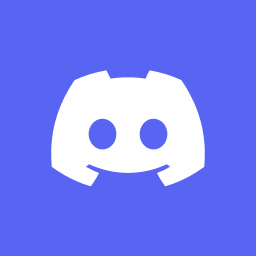
Is this cloud or self-hosted?
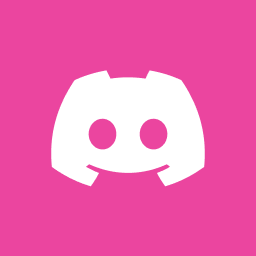
In cloud
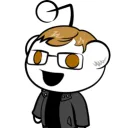
What have you set APPWRITE_API_ENDPOINT
to?
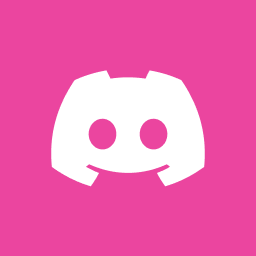
Isn't that passed during execution in cloud?
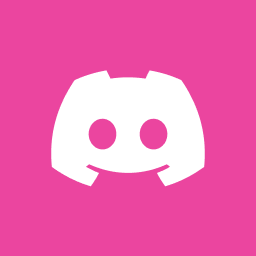
Has that changed in the recent update ?
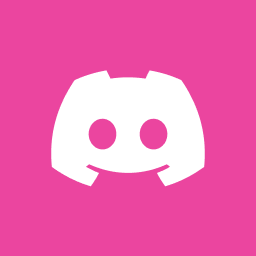
Ok now I have set APPWRITE_API_ENDPOINT='https://cloud.appwrite.io/v1'
Still the error is the same
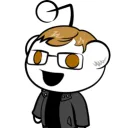
Not to my knowledge - at least, it’s not in the list of variables in the docs
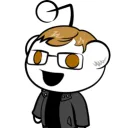
Interesting. Have you tried dumping out the vars to check that they’re actually being set correctly?
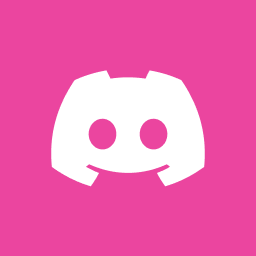
Yes I did that too, it prints whatever I have set, in fact I am using the same instantiation of client
in another function (except the JWT part), it works fine
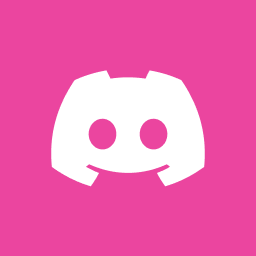
I have also found that the error is at user = await account.get()
in the authenticate function, as I am catching and error logging it.
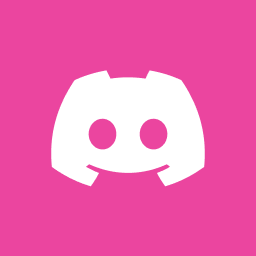
Everything before it prints in the log perfectly
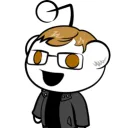
Yeah, that’s what I assumed
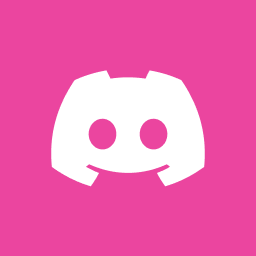
Ok
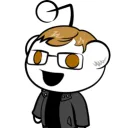
What if you just put https://cloud.appwrite.io/v1 in .setEndpoint()
, instead of using the envvar?
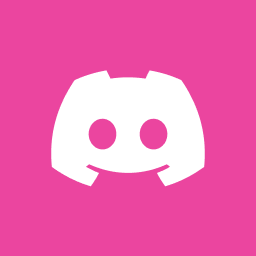
Ok trying now
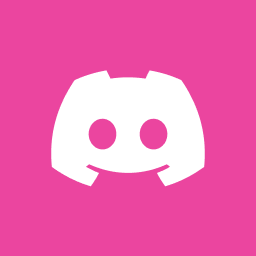
If you don't mind me asking, why do you think that the client is the problem ?
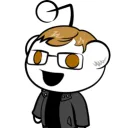
It looks like it’s trying to connect to the wrong thing. It shouldn’t be attempting a connection to 127.0.0.1 - it should be trying to connect to cloud.appwrite.io
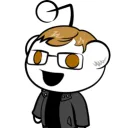
And I don’t see anything else it could be (in the code)
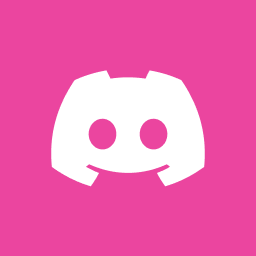
Yea it seems to be working now
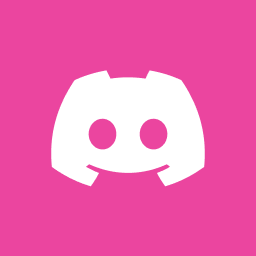
Thank you so much I did not notice the quotes here in the log for the environement variable
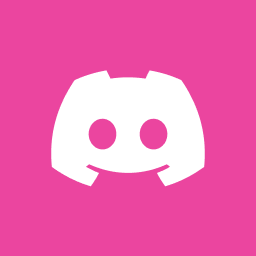
APPWRITE_API_ENDPOINT=https://cloud.appwrite.io/v1
Setting it to this works (without quotes)
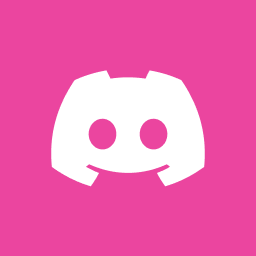
Thanks again @ideclon
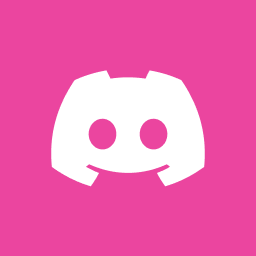
[SOLVED] Account not working in appwrite functions
Recommended threads
- How to Avoid Double Requests in function...
I'm currently using Appwrite's `functions.createExecution` in my project. I want to avoid double requests when multiple actions (like searching or pagination) a...
- Project in AppWrite Cloud doesn't allow ...
I have a collection where the data can't be opened. When I check the functions, there are three instances of a function still running that can't be deleted. The...
- Get team fail in appwrite function
I try to get team of a user inside appwrite function, but i get this error: `AppwriteException: User (role: guests) missing scope (teams.read)` If i try on cl...
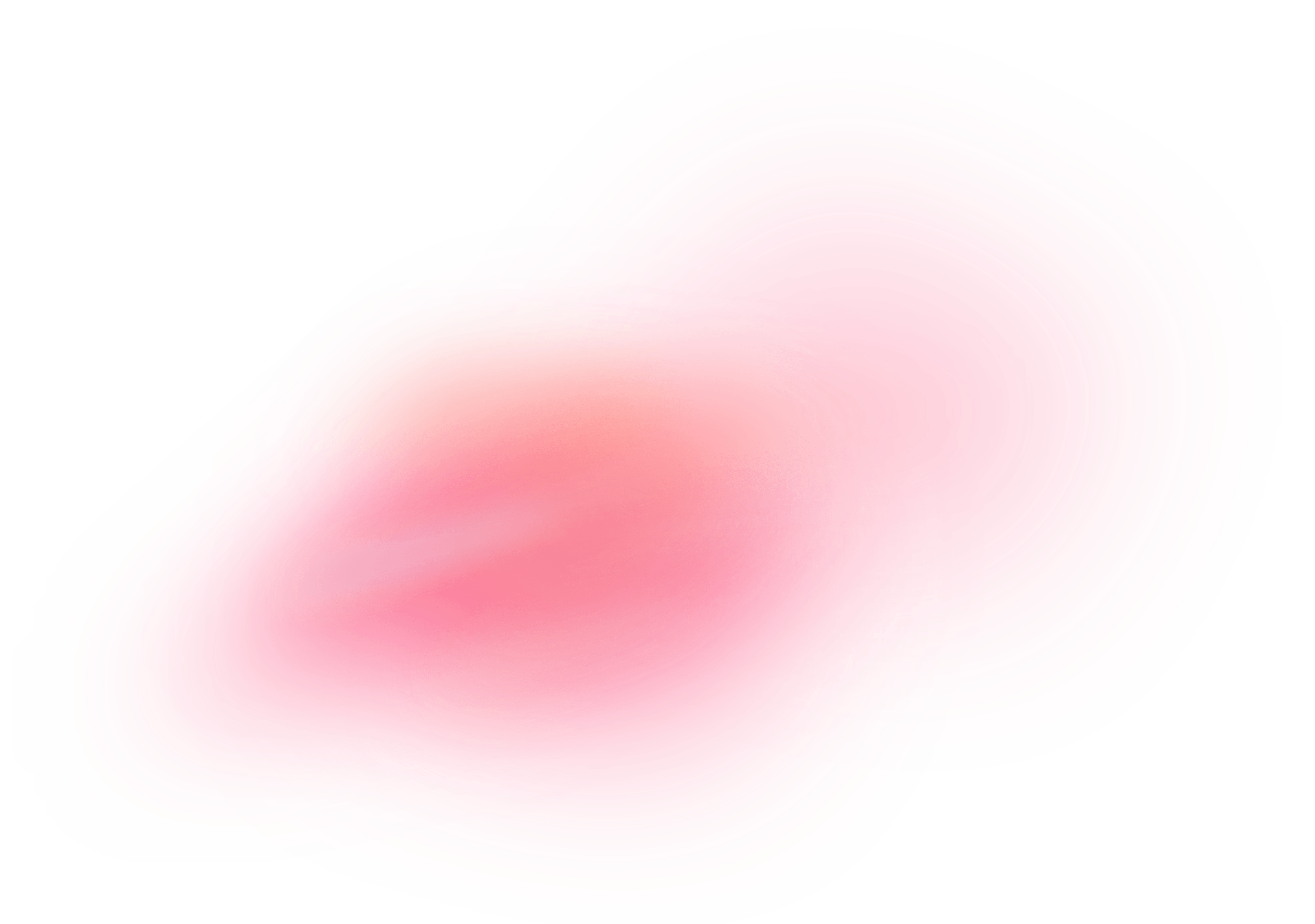