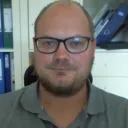
I have rewritten a working node cloud function to the new syntax. The function is triggered on a create or update event in a bucket.
When I try to access the file that triggered the function I have the following Error: "An internal curl error has occurred within the executor! Error Msg: Http invalid protocol\nError Code: 500"
I'm testing on a local Appwrite instance. All other functions I have rewritten are working. Only storage access seems to be broken.
Do you have an idea what could cause this problem?
openruntimes-executor | [Error] Message: An internal curl error has occurred within the executor! Error Msg: Http invalid protocol
openruntimes-executor | [Error] File: /usr/local/app/http.php
openruntimes-executor | [Error] Line: 1027
openruntimes-executor | Skipping runtimes stats loop due to error: Docker Error: EOF
[...]
const promise = storage.getFileView(bucketID, fileID);
promise.then(
function (response) {
log(response);
},
function (error) {
log(error);
}
);
[...]
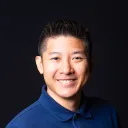
Did you create a new function or did you update a pre-1.4 function?
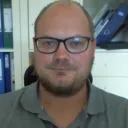
I created a new one
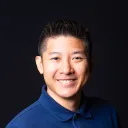
Where are you returning the response?
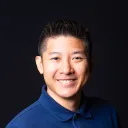
Also, it can be problematic to log the response if the response is so big. And passing error to log can be problematic too
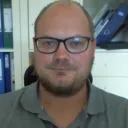
Thanks, I will check this tomorrow.
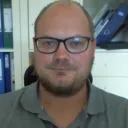
I made a new cloud Funktion on Appwrite 1.4.11 to try to get something running:
/*
appwrite functions createDeployment --functionId=test --entrypoint='src/main.js' --code='functions/test/' --activate=true
*/
import { Client, Storage } from 'node-appwrite';
export default async (context) => {
const client = new Client();
client
.setEndpoint(process.env.APPWRITE_ENDPOINT)
.setProject(process.env.APPWRITE_FUNCTION_PROJECT_ID)
.setKey(process.env.APPWRITE_API_KEY);
const storage = new Storage(client);
const promise = storage.getFileView(
'62a8722a6d1f2f03c06d',
'6553637de685181ec36a'
);
promise.then(
function (response) {
context.log(response);
},
function (error) {
context.error(error);
}
);
// `res.json()` is a handy helper for sending JSON
return context.res.json({
motto: 'Build like a team of hundreds_',
learn: 'https://appwrite.io/docs',
connect: 'https://appwrite.io/discord',
getInspired: 'https://builtwith.appwrite.io',
});
};
I do not get any logs or error messasges in the backend.
In the openruntimes-executor log I hav the following error:
openruntimes-executor | [Error] Type: Exception
openruntimes-executor | [Error] Message: Multiple internal curl errors has occurred within the executor! Error Number: 111. Error Msg: Connection refused
openruntimes-executor | [Error] File: /usr/local/app/http.php
openruntimes-executor | [Error] Line: 1031
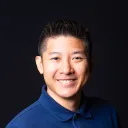
I highly recommend using appwrite init function
and appwrite deploy function
instead of manually deploying with appwrite functions createDeployment
Do you have the build command set to npm install
?
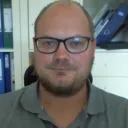
I did some more testing and deployed using "appwrite deploy function", npm install is set.
This is working:
import { Client, Storage } from 'node-appwrite';
export default async (context) => {
const client = new Client()
.setEndpoint(process.env.APPWRITE_ENDPOINT)
.setProject(process.env.APPWRITE_FUNCTION_PROJECT_ID)
.setKey(process.env.APPWRITE_API_KEY);
const storage = new Storage(client);
//const bucketID = req.body.bucketId;
//const fileID = req.body.$id;
const file = await storage.getFile(
'62a8722a6d1f2f03c06d',
'6554756f9b5ca53ac199'
);
context.log(file);
return context.res.send('ok');
};
This Version (using storage.getFileView) throws the error "An internal curl error has occurred within the executor! Error Msg: Http invalid protocol\nError Code: 500"
import { Client, Storage } from 'node-appwrite';
export default async (context) => {
const client = new Client()
.setEndpoint(process.env.APPWRITE_ENDPOINT)
.setProject(process.env.APPWRITE_FUNCTION_PROJECT_ID)
.setKey(process.env.APPWRITE_API_KEY);
const storage = new Storage(client);
//const bucketID = req.body.bucketId;
//const fileID = req.body.$id;
const file = await storage.getFileView(
'62a8722a6d1f2f03c06d',
'6554756f9b5ca53ac199'
);
context.log(file);
return context.res.send('ok');
};
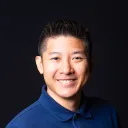
What if you remove the context.log()
line?
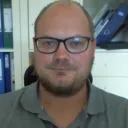
Then it works. Tried it a few hours ago and removed the logs in all the functions to get everything running again.
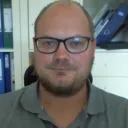
Is there a "proper" way of debugging when the log and error functions cant be used?
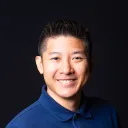
Can you create a GitHub issue with this detail? Ideally, the log works and you don't end up with a 500 error with no info at all
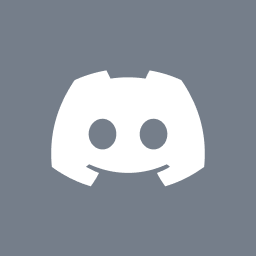
I have a similar problem in 1.3.7 where the function results in no output other than "An internal curl error has occurred within the executor! Error Msg: Operation timed out". What is wrong with "appwrite functions createDeployment"? (This part works just fine.)
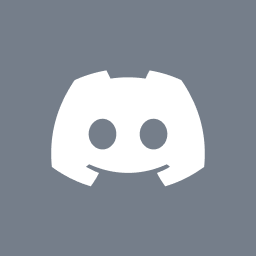
Oh, i am passing json to an env variable like so API_KEY=password GOOGLE_CREDENTIALS_JSON='{ json content }' is that OK?
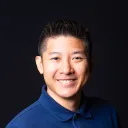
Please create a separate post instead of posting in someone else's
Recommended threads
- Is Quick Start for function creation wor...
I am trying to create a Node.js function using the Quick Start feature. It fails and tells me that it could not locate the package.json file. Isn't Quick Start ...
- Connecting server functions to GitHub re...
The project I am working in has recently moved organizations on Appwrite. The same is true for the repo on GitHub, which as moved from a private user to a organ...
- Missing C++ libstdc library in Python fu...
I have a function running Python 3.12 which suddenly started dumping errors (as of today; it worked yesterday). I hadn't changed any code so I found this odd, b...
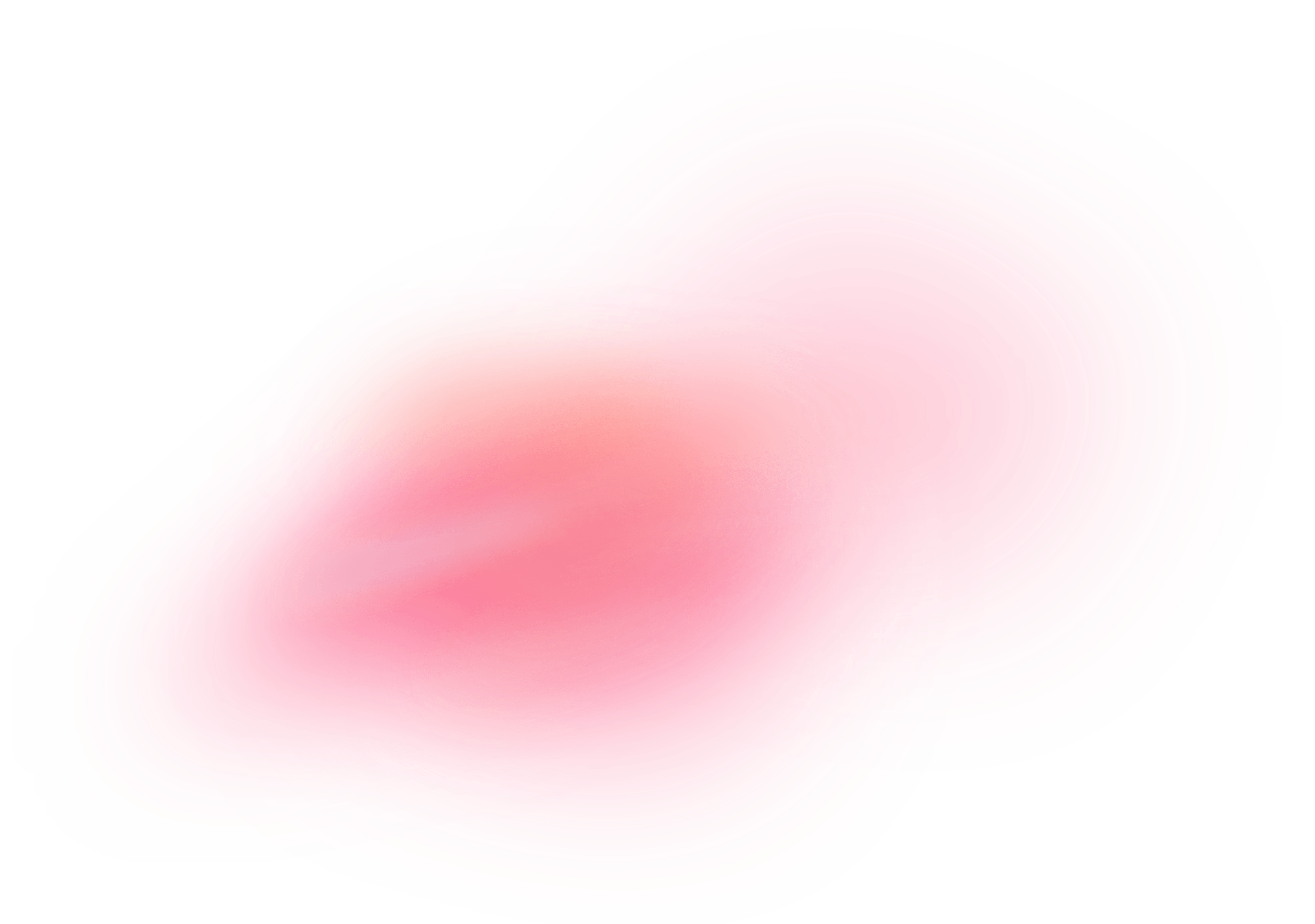