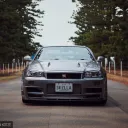
I keep getting the error A user with the same id, email, or phone already exists in this project.
, I have tried looking through previous posts and docs. None of which seems to help resolve the issue. I am selfhosting, and if i create an account from the dashboard it is fine. My code is below.
import { SafeAreaView, TouchableOpacity } from "react-native";
import { Card, Text, TextInput } from "react-native-paper";
import {Account, Client, ID } from "appwrite";
import PropTypes from "prop-types";
import { validateName, validateEmail, validatePassword } from "../../utils/validators";
import AuthLogo from "../../components/AuthLogo";
import AppStyle from "../../styling/AppStyling";
import AuthStyle from "../../styling/AuthStyling";
SignUpScreen.propTypes = {
navigation: PropTypes.shape({
navigate: PropTypes.func.isRequired,
}).isRequired,
};
export default function SignUpScreen({ navigation }) {
const [firstName, setFirstName] = useState("");
const [lastName, setLastName] = useState("");
const [email, setEmail] = useState("");
const [password, setPassword] = useState("");
const [confirmPassword, setConfirmPassword] = useState("");
const client = new Client();
client
.setEndpoint("Omitted")
.setProject("Omitted");
const handleSignUp = async () => {
const account = new Account(client);
const promise = await account.create(ID.unique(), email, password, `${firstName} ${lastName}`);
promise.then(function () {
navigation.navigate("Login");
}, function (error) {
console.log(error); // Failure
});
};```
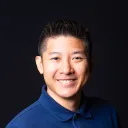
Check the network logs in the browser to see what's being sent to the server for the payload
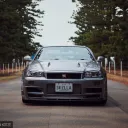
I'm using react native w/ expo. I know there is an option to open in the web, but it won't build a usable app. Do you know of another way to see the payload?
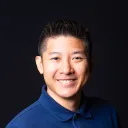
Then try logging the parameters before calling the create
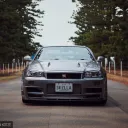
I did try this last night, but I did try it again for you
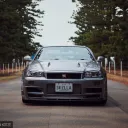
Is that what ID.unique( ) is supposed to return?
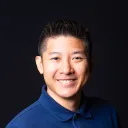
Yep that's expected
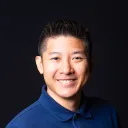
And no user with that email already exists?
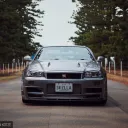
correct, I have tried with several different emails. All of which return that same error. I have even tried hardcoding a string into the create function as well. But to no avail, I keep getting that same error when trying to create the account.
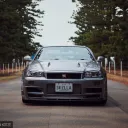
I checked the docs to make sure all params are in the correct order and valid
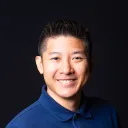
What happens if you call account.get()
before the create?
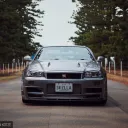
does it take any arguments?
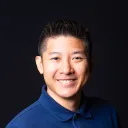
No
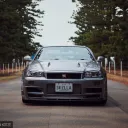
Okay, one moment
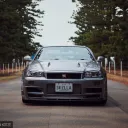
So that is showing that I am logged in using an account I created manually but never logged in with?
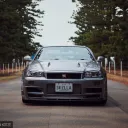
when i use the account.get( )
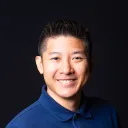
The SDK persists sessions automatically. So you're seeing the weird behavior because you're already logged in but trying to create an account again
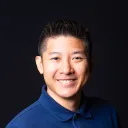
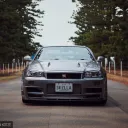
okay, thank you. So when you create an account, it automatically logs the user in? Or would I had to have logged in using the createEmailSession? Also do you happen to have the code for logging out of a session?
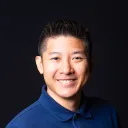
No, it doesn't automatically log the user in
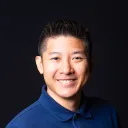
To log out, use the delete session API
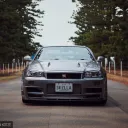
Okay, I am not sure exactly how that happened. This is for a group project, so maybe one of my group partners may have logged it in. My apologies, thank you for the help. But that fixed it. Thank you!
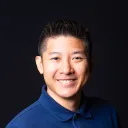
[SOLVED] Unable to create account
Recommended threads
- Google sign in wont allow you to choose ...
Hi, this is still unsolved, Appwrite's SDK won't let you specify prompt=select_account param, so a user cannot signin with a desired account, in chrome is just ...
- Console ErrorAppwriteException: User (ro...
AppwriteException: User (role: guests) missing scope (account) at Client.eval (webpack-internal:///(app-pages-browser)/./node_modules/appwrite/dist/esm/sdk....
- AppwriteException: Project ID not found
I'm getting this error: `Error signing up: AppwriteException: Project with the requested ID could not be found. Please check the value of the X-Appwrite-Project...
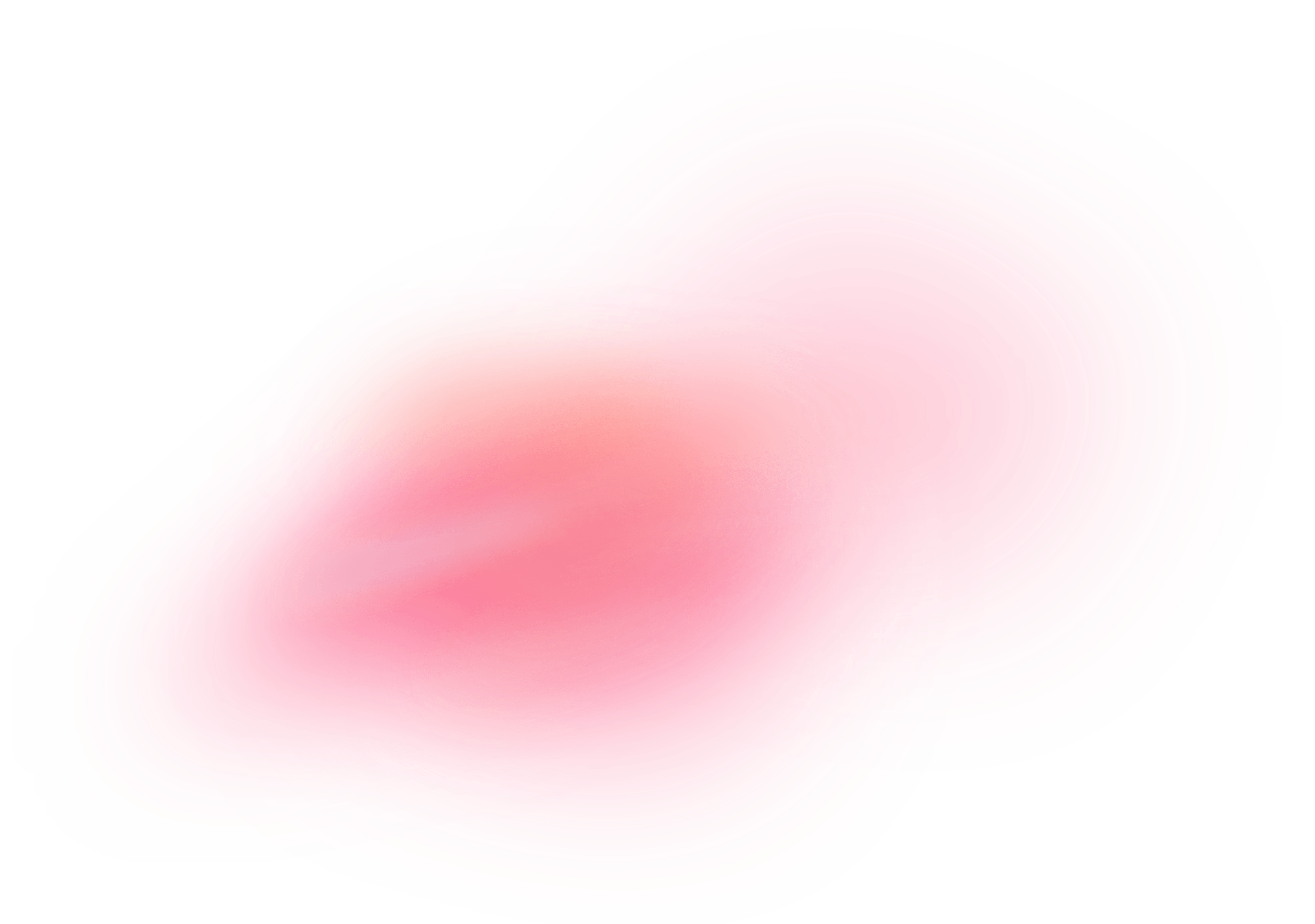