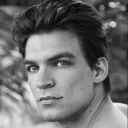
so this is my main.py
from appwrite.client import Client
from appwrite.services.databases import Databases
from appwrite.services.users import Users
from appwrite.query import Query
from .claude_functions import ClaudeFunctions
import os
# This is your Appwrite function
# It's executed each time we get a request
def main(context):
# Why not try the Appwrite SDK?
client = (
Client()
.set_endpoint("https://zappwrite.com/v1")
.set_project(os.environ["APPWRITE_FUNCTION_PROJECT_ID"])
.set_key(os.environ["APPWRITE_API_KEY"])
)
appwriteusers = Users(client)
appwritedb = Databases(client)
personalityAssessments = appwritedb.list_documents(
"maindb", "personality_assessments", [Query.limit(1000)]
)
context.log(
f"Found {len(personalityAssessments['documents'])} personality assessments"
)
claude_functions = ClaudeFunctions.get_instance(context)
# Go through our personality assessments and index any that need to be indexed
for assessment in personalityAssessments:
if assessment.get("indexed") == False:
claude_functions.index_personality_assessment(
assessment.get("$id"), assessment.get("description")
)
# Get the user ID
user_id = context.req.body.get("userId")
chat_id = context.req.body.get("chatId")
user_message = context.req.body.get("userMessage")
context.log(f"User ID in request: {user_id}\nChat ID in request: {chat_id}")
# Get the user
user = appwriteusers.get(user_id)
# If we have a user we're good to go
if user:
user_messages = appwritedb.list_documents(
"maindb",
"chat_messages",
[Query.limit(1000), Query.equal("chatId", chat_id)],
).get("documents")
response = claude_functions.chat(user, user_message, user_messages)
return context.res.json(response)
else:
context.error("User not found")
return context.res.json({"error": "User not found"})
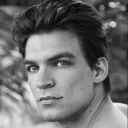
still the same error
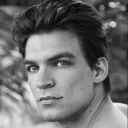
Traceback (most recent call last):
File "/usr/local/server/src/server.py", line 165, in handler
output = await asyncio.wait_for(execute(context), timeout=safeTimeout)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "/usr/local/lib/python3.11/asyncio/tasks.py", line 479, in wait_for
return fut.result()
^^^^^^^^^^^^
File "/usr/local/server/src/server.py", line 150, in execute
userModule = importlib.import_module("function." + userPath)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "/usr/local/lib/python3.11/importlib/__init__.py", line 126, in import_module
return _bootstrap._gcd_import(name[level:], package, level)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "<frozen importlib._bootstrap>", line 1206, in _gcd_import
File "<frozen importlib._bootstrap>", line 1178, in _find_and_load
File "<frozen importlib._bootstrap>", line 1149, in _find_and_load_unlocked
File "<frozen importlib._bootstrap>", line 690, in _load_unlocked
File "<frozen importlib._bootstrap_external>", line 940, in exec_module
File "<frozen importlib._bootstrap>", line 241, in _call_with_frames_removed
File "/usr/local/server/src/function/src/main.py", line 5, in <module>
from .claude_functions import ClaudeFunctions
File "/usr/local/server/src/function/src/claude_functions.py", line 15, in <module>
OPENAI_API_KEY = os.environ("OPENAI_API_KEY")
^^^^^^^^^^^^^^^^^^^^^^^^^^^^
TypeError: '_Environ' object is not callable
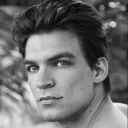
only difference is i'm using 3.11 runtime
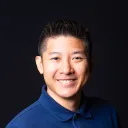
what's the code in claude_functions.py
?
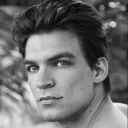
uh
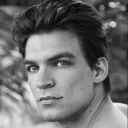
AI code
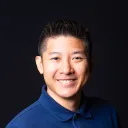
line 15...
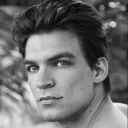
oh
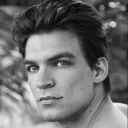
# AI Imports
from llama_index.llms import Anthropic, OpenAI, ChatMessage, MessageRole
from llama_index.chat_engine.types import ChatMode
from llama_index.vector_stores import WeaviateVectorStore
from llama_index import VectorStoreIndex, ServiceContext, Document
from langchain.embeddings.huggingface import HuggingFaceEmbeddings
import anthropic
import openai
from pydantic import BaseModel, Field
# Standard Imports
import os
import weaviate
OPENAI_API_KEY = os.environ("OPENAI_API_KEY")
CLAUDE_API_KEY = os.environ("CLAUDE_API_KEY")
anthropic.Anthropic.api_key = CLAUDE_API_KEY
openai.api_key = OPENAI_API_KEY
weaviate_auth = weaviate.AuthApiKey(api_key=os.environ("WEAVIATE_KEY"))
weaviate_url = os.environ("WEAVIATE_URL")
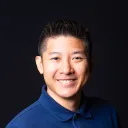
there's hte problem...
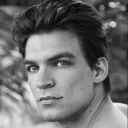
why>
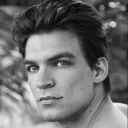
you do realize in the default Python code
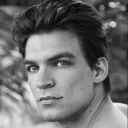
you guys use os.environ
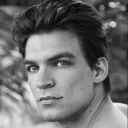
to access the Appwrite vars
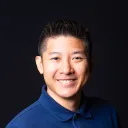
its with brackets, not parenthesis
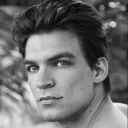
oh
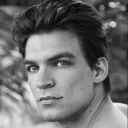
does os.getenv work?
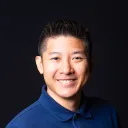
yes
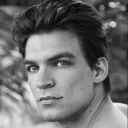
okay, huh, is there a list of tricks to this?
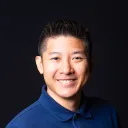
no this is default python behavior
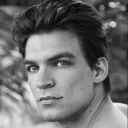
like the .module
trick to import
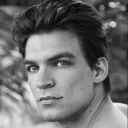
oh I guess I normally use getenv then
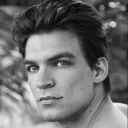
my bad.
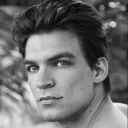
[SOLVED] Appwrite Function "No Module Found"
Recommended threads
- Properly contained appwrite main app can...
Hello! We tried to reinstall our main self-hosted appwrite with a new method but the main app 2 mins after launch throw this error: ```2025/06/22 16:16:14 s...
- Broken message
https://github.com/appwrite/appwrite/issues/10081 I just realized that I can just build appwrite myself, was this bug fixed in latest dev release?
- 404 errors after 7 Days
Local hosted Appwrite via docker. Last version and current version. After exactly 7 days Appwrite stops working. I get 404 route not found, cannot access anyth...
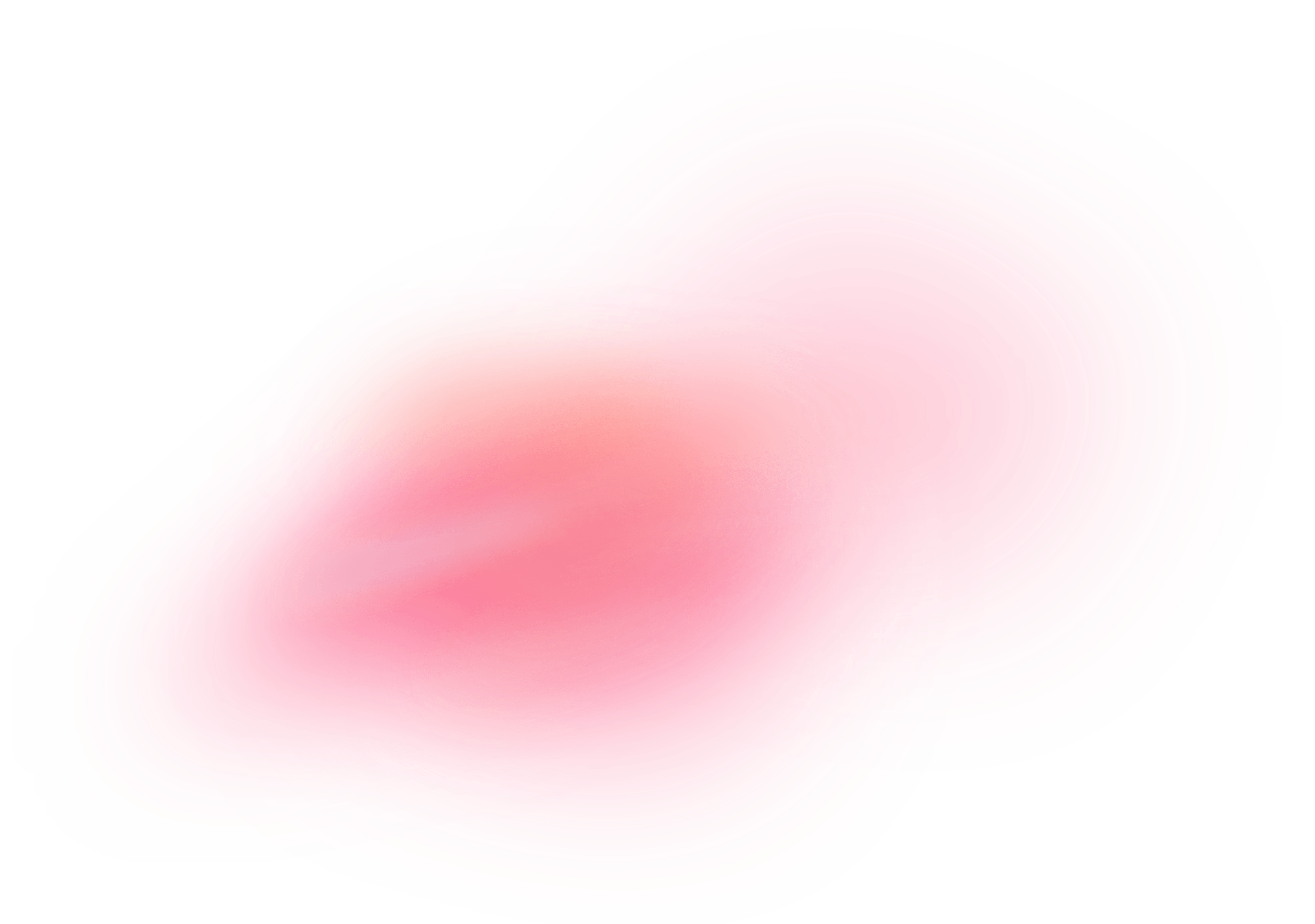