
Hey Appwriters :),
I am new to WebDev, majorily worked in Mobile Industry. So, I have zero knowledge of CORS Errors. I made public cloud function in appwrite using python, wanted to use that in React Project, but for some reasons I am constantly facing CORS error, whereas i can use the function in curl
, browser
and postman
.
This is my source code of appwrite function,
from appwrite.client import Client
import os
from .notion_api import get_top_news
NOTION_API_KEY = os.environ["NOTION_API_KEY"]
def main(context):
return context.res.json(
{
"notion_top_news": get_top_news(NOTION_API_KEY),
}
)
Whereas I am calling it like this,
export async function fetchTopNews(): Promise<{ notion_top_news: { document_id: string; headline: string; image_url: string; summary: string } }> {
const url = 'https://<function-id>.appwrite.global/';
try {
const response = await fetch(url);
if (!response.ok) {
throw new Error(`Request failed with status: ${response.status}`);
}
const data = await response.json();
if (!data.notion_top_news || typeof data.notion_top_news !== 'object') {
throw new Error('Invalid JSON format in response');
}
return data as {
notion_top_news: {
document_id: string;
headline: string;
image_url: string;
summary: string;
};
};
} catch (error) {
throw new Error(`Failed to fetch data: ${error}`);
}
}

So, When i run react on locahost:3000, it is throwing error.
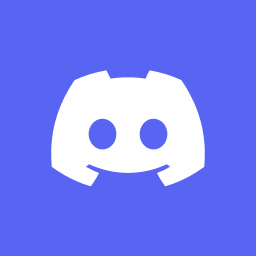
You will need to add a platform in the appwrite dashboard and add localhost as hostname

hey @D5 , i have already added a client...


is there any tutorial or any other sample that explain how to use cloud function only from React/etc framework?

hey i just check console,
localhost/:1 Access to fetch at 'https://<function-id>.appwrite.global/' from origin 'http://localhost:3000' has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource. If an opaque response serves your needs, set the request's mode to 'no-cors' to fetch the resource with CORS disabled.

so how to add header in response?
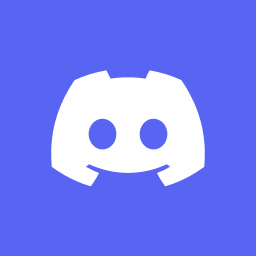
If the function is not hosted in cloud, why you're calling it like that? I mean, by using appwrite.global domain

function is hosted to cloud.(I removed the actual function host name, because it is proxy for notion api, i don't want to it ping it unwantedly)

it surely not giving header, can set custom response headers?

Access-Control-Allow-Origin

oh yeah, i got the part of CORS, in python sample, https://github.com/appwrite/templates/blob/954c0c09cfc748ee7427d8972a6f0e6d9b70be74/python/email_contact_form/src/main.py#L77C13-L77C29
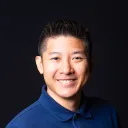
do you need to use the function domain? Why not use the Appwrite SDK?
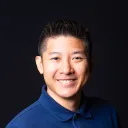
i think the problem is the user doesn't have access to make that fetch api call
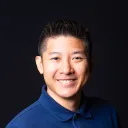
Getting CORS Error during Appwrite Function from localhost?

Hey @Steven , I am using function as proxy b/w notion API and caching markdown in appwrite storage bucket. thinking that it will be much better that I just remove appwrite specific code from website. So, that if I need to host my own flask server in future, then I don't have to remove appwrite SDK. Currently my whole project only depends on functions only. and In functions I am accessing storage and db.
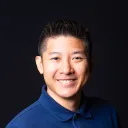
i see okay. so what are the permissions set on this function?

for now it is any, and api allow localhost only. for now. later on deployment i will also deployement url+localhost only.
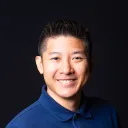
and api allow localhost only
What do you mean?
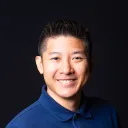
you may need to return Access-Control-Allow-Origin header to allow CORS then

yes, btw it isn't localhost but wildcard(*).

btw thanks Steven.
Recommended threads
- Adding custom domain to Appwrite project
My app is hosted on Vercel on domain todo.velleb.com. On PC, the OAuth logins like GitHub, Discord and Google work and create the account and log in. On mobile ...
- Appwrite CLI
I try to deploy a function using Appwrite CLI but it says: `appwrite functions createDeployment ^ --functionId=xyz^ --code="." ^ --activate error: u...
- #support
<#1072905050399191082> any help on that?
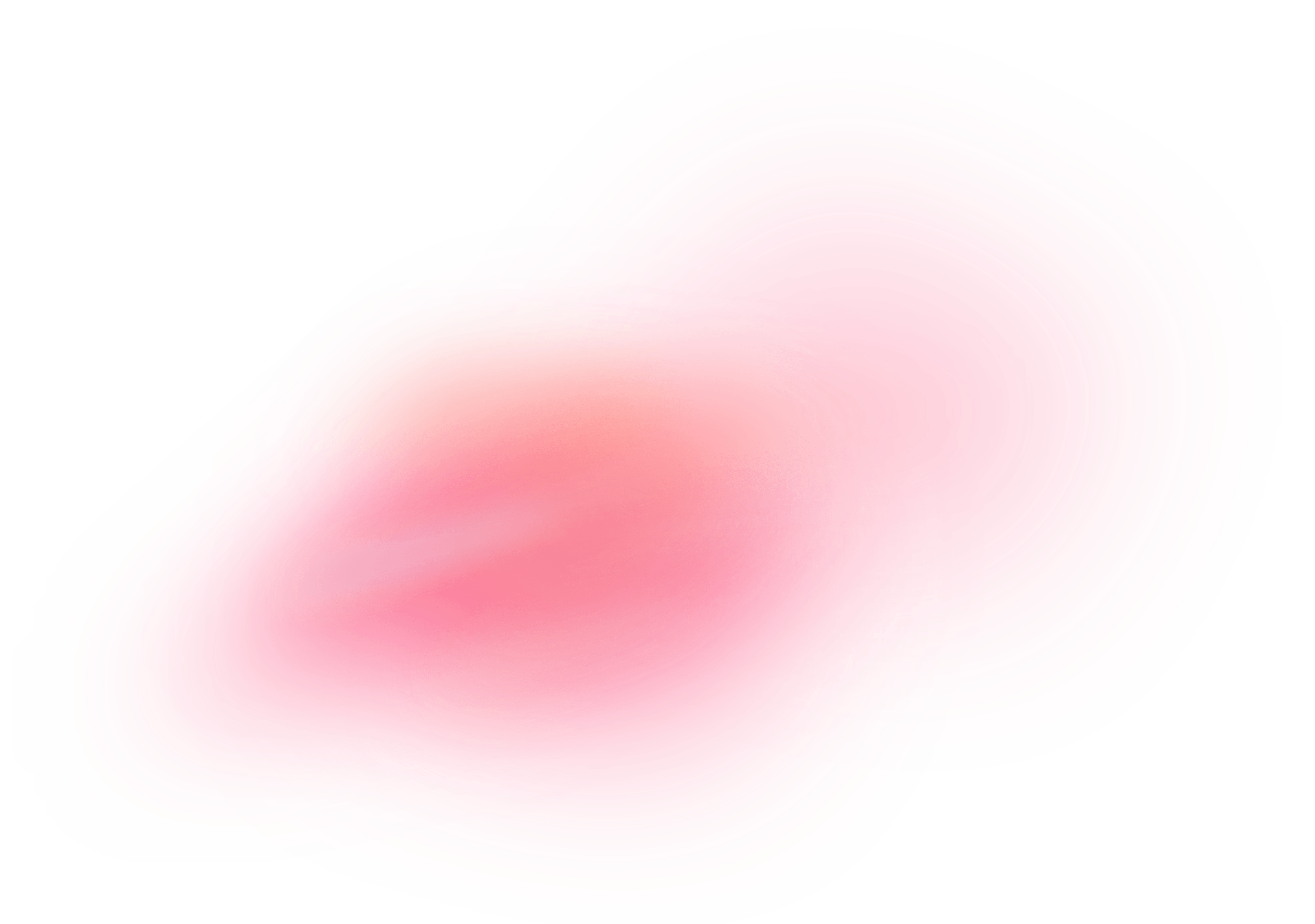