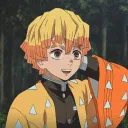
// Prepare and send the WhatsApp message
$headers = [
"Content-Type" => "application/json",
"Accept" => "application/json",
];
$data = [
"from" => $_ENV["VONAGE_WHATSAPP_NUMBER"],
"to" => $body["from"],
"message_type" => "text",
"text" => 'Hi there! You sent me: ' . $body["text"],
"channel" => "whatsapp",
];
$client = new Client();
$url = "https://messages-sandbox.nexmo.com/v1/messages";
try {
$response = $client->post($url, [
"headers" => $headers,
"json" => $data,
"auth" => [
$_ENV["VONAGE_API_KEY"],
$_ENV["VONAGE_API_SECRET"]
],
]);
if ($response->getStatusCode() === 200) {
$result = json_decode($response->getBody(), true);
return $context->res->json(["ok" => true]);
} else {
return $context->error("Error " . $response->getBody());
}
} catch (Exception $e) {
return $context->res->json(["ok" => false], 500);
}
};
?>
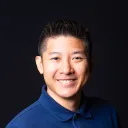
btw, it's helpful to include the language after the 1st 3 backticks to enable syntax highlighting
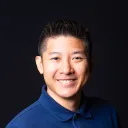
any errors or logs on that execution tab?
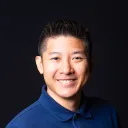
you can't return an error like:
return $context->error("Error " . $response->getBody());
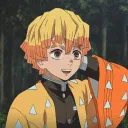
yeah, I'm getting
An internal curl error has occurred within the executor! Error Msg: Operation timed out\nError Code: 500
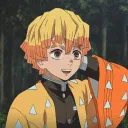
I've updated the code:
<?php
require(__DIR__ . '/../vendor/autoload.php');
require(__DIR__ . '/utils.php');
use \Firebase\JWT\JWT;
use GuzzleHttp\Client;
return function ($context) {
throw_if_missing($_ENV, [
'VONAGE_API_KEY',
'VONAGE_API_SECRET',
'VONAGE_API_SIGNATURE_SECRET',
'VONAGE_WHATSAPP_NUMBER',
]);
if ($context->req->method === 'GET') {
return $context->res->send(get_static_file('index.html'), 200, [
'Content-Type' => 'text/html; charset=utf-8',
]);
}
$body = $context->req->body;
$headers = $context->req->headers;
$token = (isset($headers["Authorization"])) ? explode(" ", $headers["Authorization"])[1] : "";
try {
$decoded = JWT::decode($token, $_ENV['VONAGE_API_SIGNATURE_SECRET'], ['HS256']);
$context->log($decoded);
} catch (Exception $e) {
$context->error("JWT decoding error: " . $e->getMessage());
}
if (hash('sha256', $context->req->bodyRaw) !== $decoded['payload_hash']) {
return $context->res->json([
'ok' => false,
'error' => 'Payload hash mismatch'
]);
}
try {
throwIfMissing($context->req->body, ['from', 'text']);
} catch ( Exception $e) {
return $context->res->json([ "ok"=> false, "error"=> $e->getMessage()], 400);
}
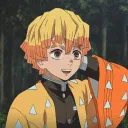
$vonageApiKey = $_ENV['VONAGE_API_KEY'];
$vonageAccountSecret = $_ENV['VONAGE_API_SECRET'];
$basicAuthToken = base64_encode("$:$vonageAccountSecret");
$headers = [
'Content-Type:application/json',
'Accept:application/json'
];
$data = [
'from' => $_ENV['VONAGE_WHATSAPP_NUMBER'],
'to' => $context->req->body['from'],
'message-type' => 'text',
'text' => 'Hi there! You sent me: ' . $context->req->body['text'],
'channel' => 'whatsapp'
];
$client = new Client();
$url = "https://messages-sandbox.nexmo.com/v1/messages";
try {
$response = $client->post($url, [
"headers" => $headers,
"json" => $data,
"auth" => [
$_ENV["VONAGE_API_KEY"],
$_ENV["VONAGE_API_SECRET"]
],
]);
if ($response->getStatusCode() === 200) {
$result = json_decode($response->getBody(), true);
return $context->res->json(["ok" => true]);
} else {
return $context->error("Error " . $response->getBody());
}
} catch (Exception $e) {
return $context->res->json(["ok" => false], 500);
}
}
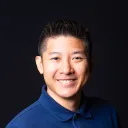
I still see return $context->error("Error " . $response->getBody());
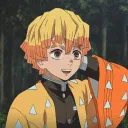
I did fix it but still getting the same error. Is it related to syntax?
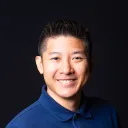
maybe. or maybe it's a network problem.
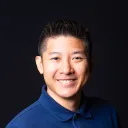
you should probably return json after $context->error("JWT decoding error: " . $e->getMessage());
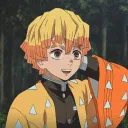
i'm a bit confused because if it was a network problem the get request should not be fulfilled right? but only the post request is failing
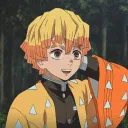
oh, i'll check
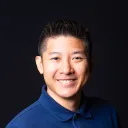
I mean network problem between the runtime container and nexmo causing a timeout
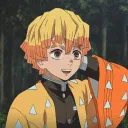
hm 🤔
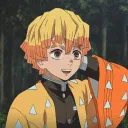
there should be a way to resolve this if that's the case
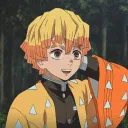
still on the same error
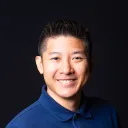
Maybe increase the timeout? Maybe remove code until it works and then incrementally add so you can narrow down where the problem is?
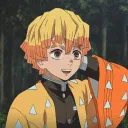
Increased timeout results in
Operation timed out after 30001 milliseconds with 0 bytes received with status code 0\nError Code: 0
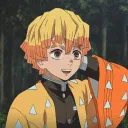
I think i should probably track down the code as you suggested
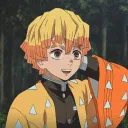
that'd be better
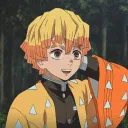
Update:
Issue Resolved ✅
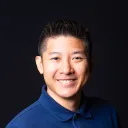
what was the problem?
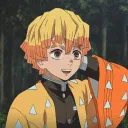
there were a bunch of errors that were preventing the post request from executing, Implementing try and catch blocks instead resolved the issue
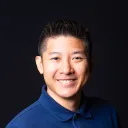
[SOLVED] POST Requests are failing
Recommended threads
- ❗[Help] Function stuck in "waiting" stat...
Hi Appwrite team 👋 I'm trying to contribute to Appwrite and followed the official setup instructions from the CONTRIBUTING.md guide to run the platform locall...
- deno 2 Cloud random errors
we have big problems with the functions. although we do not change anything in the function, we have the following random behaviour: - no scope permissions erro...
- Appwrite functions can't connect to data...
I'm trying to create a function that queries my database, but all database operations timeout from within the function, even though CLI access works perfectly. ...
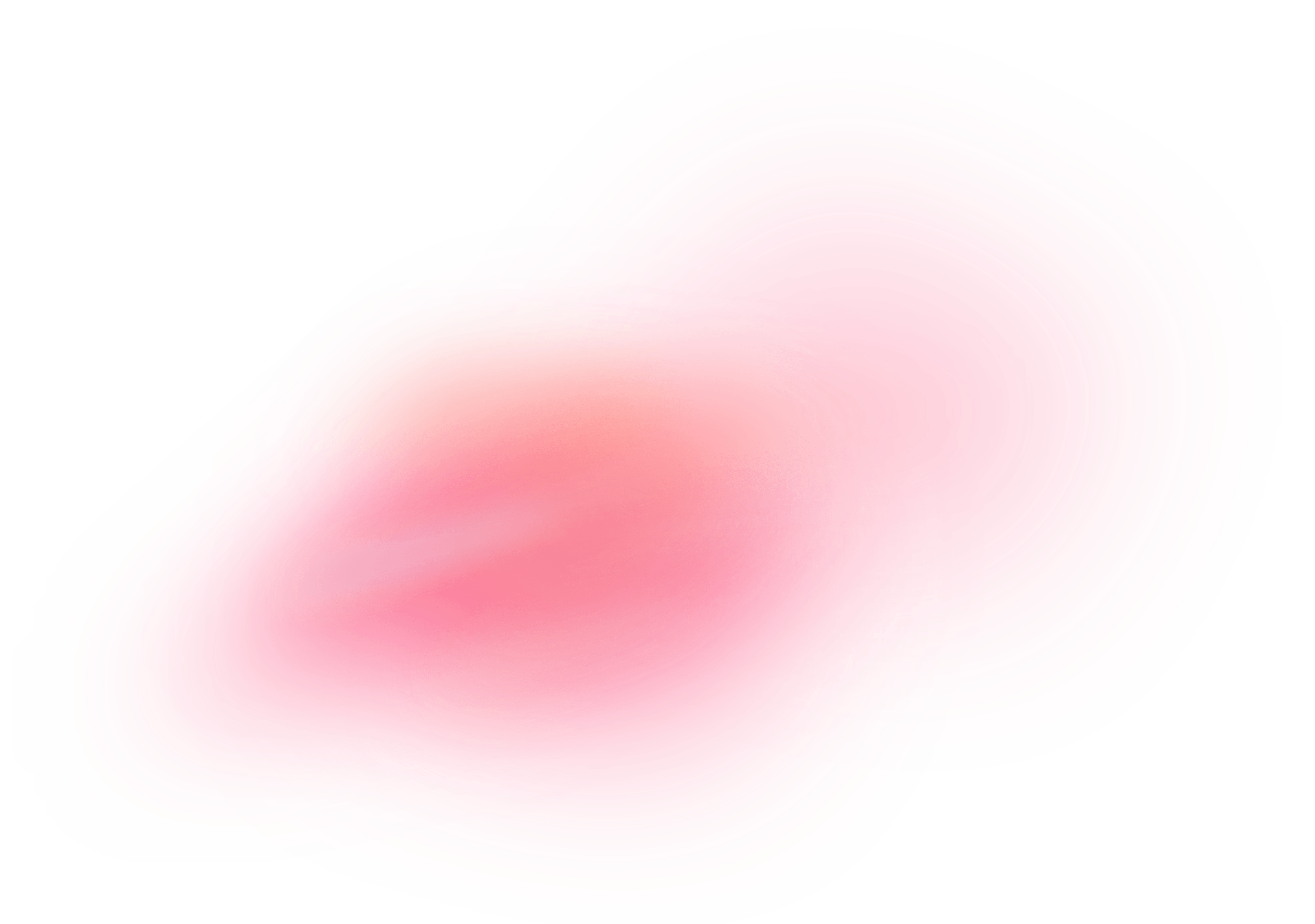