
// code for the appwrite config
import { Client, Account } from "appwrite";
import conf from "./config";
const client = new Client();
client.setEndpoint(conf.appwriteUrl).setProject(conf.appwriteProjectId);
const account = new Account(client);
export const sdk = {
register: async (success, failure) => {
// Register by creating an OAuth2 session
account.createOAuth2Session("github", success, failure);
},
getAccount: async () => {
//get account data
return await account.get();
},
getSession: async () => {
//get current session data
return await account.getSession("current");
},
getGithubData: async () => {
//get user's provider access token
const promise = await account.getSession("current");
const providerAccessToken = promise.providerAccessToken;
//make a request to github api
const response = await fetch("https://api.github.com/user", {
headers: { Authorization: `token ${providerAccessToken}` },
});
return await response.json();
},
logout: async () => {
// Delete the session
await account.deleteSession("current");
},
};

"use client";
import React from "react";
import Image from "@/components/Image";
import Button from "@/components/Button";
import { AiFillGithub } from "react-icons/ai";
import { BsGoogle } from "react-icons/bs";
import { sdk } from "@/conf/Appwrite";
export default function Login() {
const handleLoginGithub = () => {
sdk.register("http://localhos:3000/home", "http://localhost:3000/login");
};
return (
<div className="container h-screen w-full p-6 over">
<div className=" flex h-full justify-between items-center">
<div className="grid grid-cols-2 gap-10">
<div className="flex justify-center items-center">
<div className="flex flex-col gap-3">
<h2 className="text-5xl font-bold">Open-SpaceLink</h2>
<h2 className="text-2xl">
Open Source Marketplace - Empowering Innovation
</h2>
<div>
<Button
icon={<BsGoogle />}
text="Log in with Google"
color="secondary"
onClick={() => {
//login with google
}}
/>
<Button
icon={<AiFillGithub />}
text="Log in with Github"
color="primary"
onClick={handleLoginGithub}
/>
</div>
</div>
</div>
<Image image_url="/Login_graphic.png" alt="login" />
</div>
</div>
</div>
);
}

@Core can you please help me out?
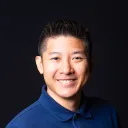
Please do not tag people or teams just because you need help as it can be very disruptive.

Sorry for that.
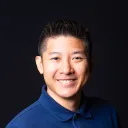
For now, you can install encoding as a dev dependency

Okay sure.

Still the same error.
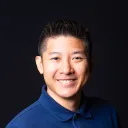
Or install as a regular dependency?

Solved thanks!
Recommended threads
- Is my approach for deleting registered u...
A few weeks ago, I was advised not to use the registered users' id in my web app. Instead, I store the publicly viewable information such as username and email ...
- Stuck in "deleting"
my parent element have relationship that doesnt exist and its stuck in "deleting", i cant delete it gives me error: Collection with the requested ID could not b...
- Help with 409 Error on Relationship Setu...
I ran into a 409 document_already_exists issue. with AppWrite so I tried to debug. Here's what I've set up: Collection A has 3 attributes and a two-way 1-to-m...
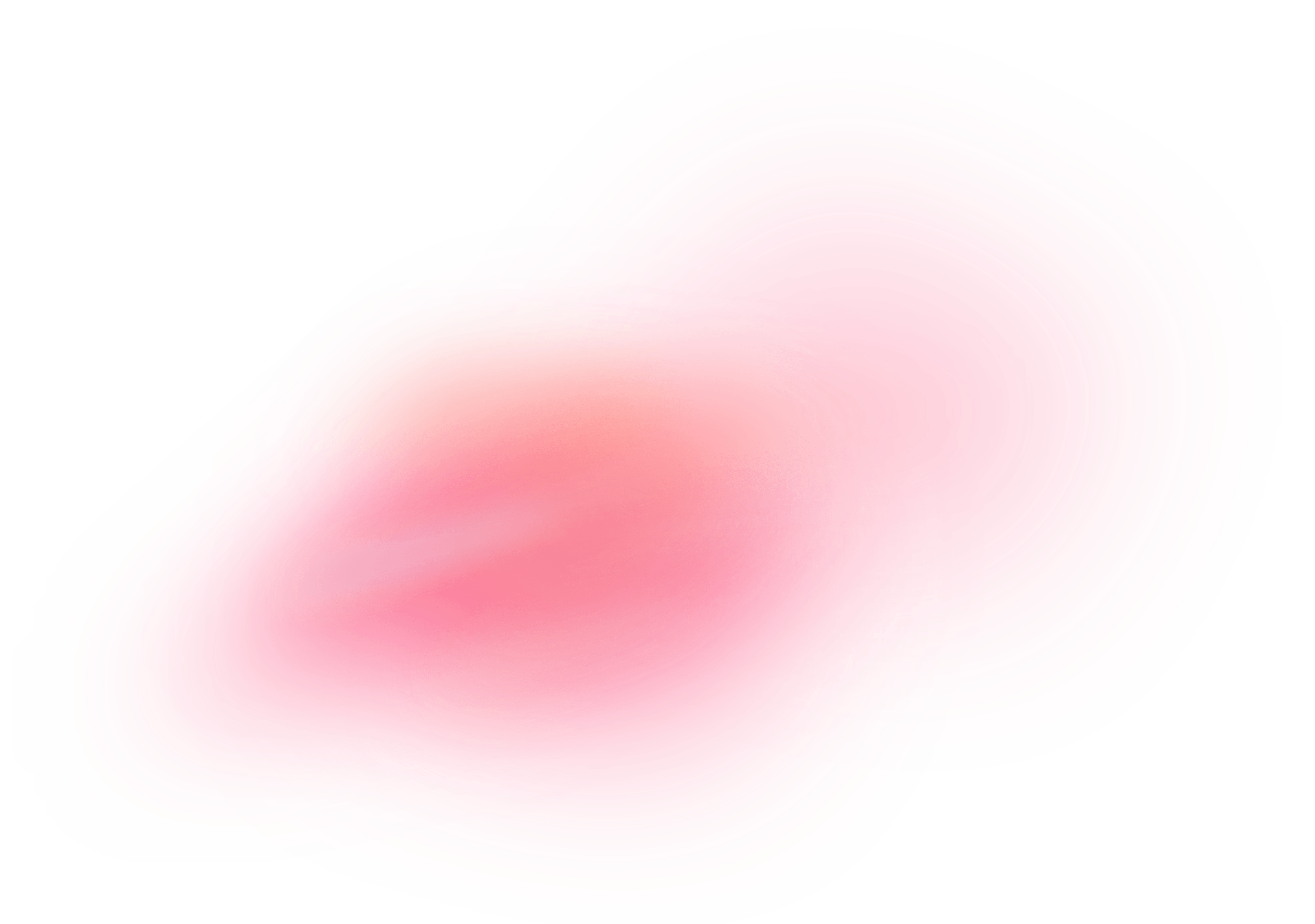