
Hey everyone,
At the moment on my app, users can create an account with any email they wish without any type of verification. I want to implement a way to verify emails on account creation. My idea was to have it so that when a user registers an account, it will send them a "login email" with a link that logs them in to their account (which means the email is verified). It shouldn't let the user access the account if they've not used that link.
This is my current code for my login and register functions:
const handleUserLogin = async (e, credentials) => {
e.preventDefault()
try {
const response = await account.createEmailSession(credentials.email, credentials.password)
const accountDetails = await account.get()
setUser(accountDetails)
navigate('/')
} catch(error) {
console.warn(error)
}
}
const handleUserRegister = async (credentials) => {
try {
let response = await account.create(
ID.unique(),
credentials.email,
credentials.password1,
credentials.name
)
await account.createEmailSession(credentials.email, credentials.password1)
const accountDetails = await account.get()
setUser(accountDetails)
navigate('/')
} catch(error) {
console.warn(error)
}
}
Does this functionality already exist within Appwrite?
Thank you in advance! 😄

Oh btw, setUser()
is just a React state that holds the returned account data to be accessed later for getting email, names etc.
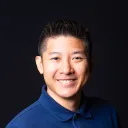
In appwrite, you would allow users to log in even if their account isn't verified. You would restrict access to resources to only verified users using permissions. To verify their email, you would call https://appwrite.io/docs/references/cloud/client-web/account#createVerification and then https://appwrite.io/docs/references/cloud/client-web/account#updateVerification after they're redirected to your app from the email

Would I just deny the read permission and add a handler for that so that it asks them to verify their email? If so, how would I differentiate the verification issue from any other reasons why the user may be denied read access.
Is there no way to just check the verification status of an account before I setUser
credentials and redirect?
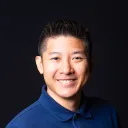
you can grant access to verified users instead of all users. The way I do it is i still setUser(), but then on whatever page they're on after login, I look at the user object to see if they're verified. If not, show a banner with a button that allows them to send verification email

Ah, okay.

So would you still recommend allowing them access to use the app even without verification?
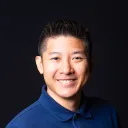
Sort of... essentially, they wouldn't be able to do anything because your UI can hide things because they aren't verified. And server-side, things are restricted to verified users.

Okay, for robustness, even if the UI is hidden, should I still add verification checks for various database interactions from the user?
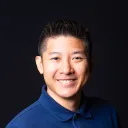
That's where the server side permissions should suffice
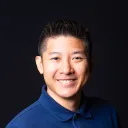
As long as you've restricted it server-side, you're fine

wym?
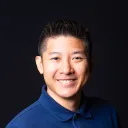
ie. restricting access to documents to only verified users
Recommended threads
- setup custom domain to appwrite but stil...
i just installed a Appwrite instance in my vps and i did all default otions exept the domain i have setuop its dns as A records pointing to my vps ip altho...
- 1.7.x Style issue in the migration page
I am not able to select what to migrate because of the styling... the checkboxes are not showing on any of the appwrite versions 1.7.x. I tried other browsers, ...
- Appwrite Sites: Max File Size
Hello, I am facing an issue on my self-hosted appwrite instance with Appwrite Sites. I already have a small vite web app built out that I want to deploy, and ...
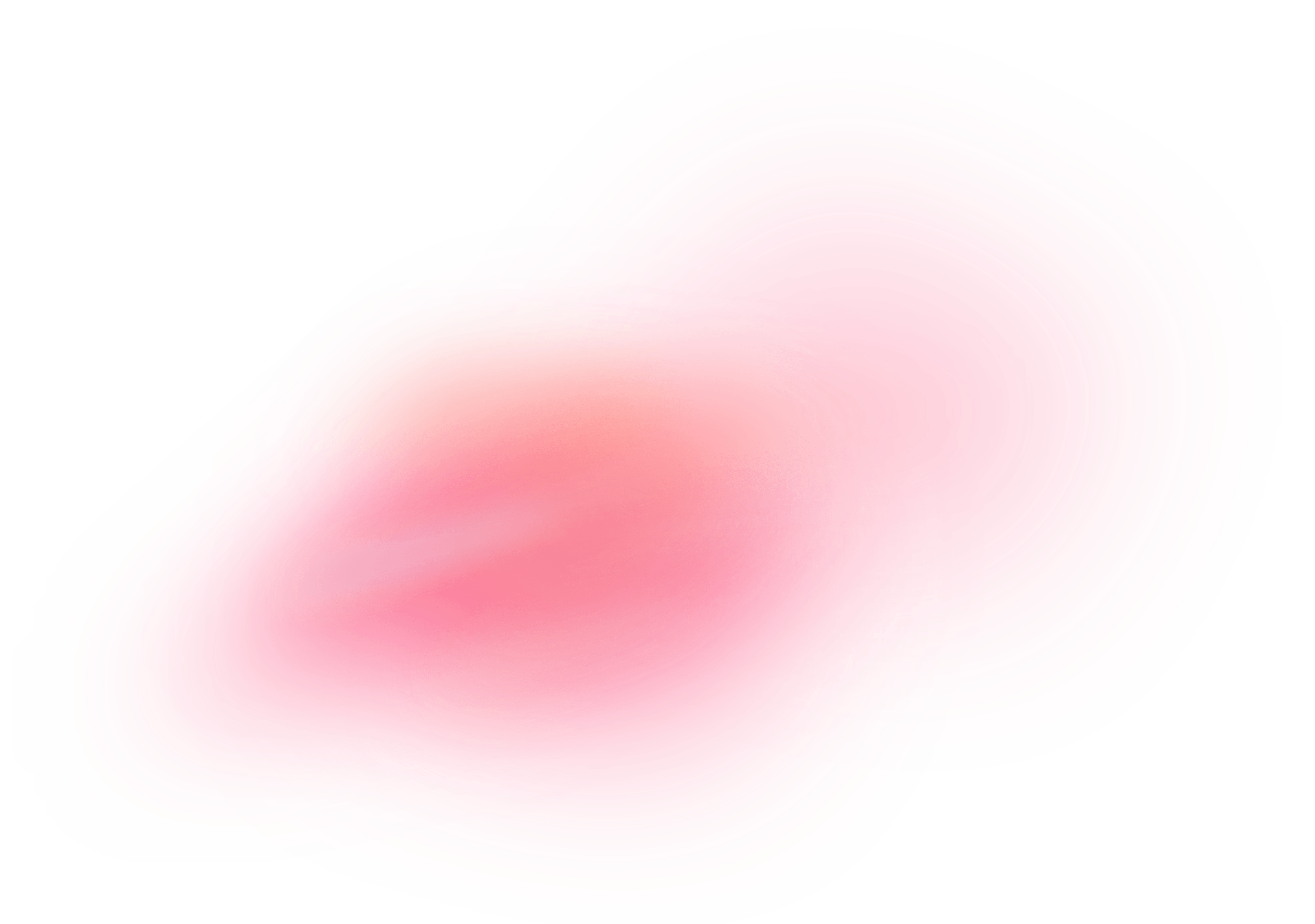