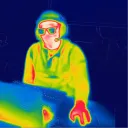
Hi, everyone. Anyone that could lend me a hand with Airtable automations here? I'm programming an Airtable automation to push newly created records on a table to a Cloud collection. This is an extract of the code:
...
const about_us = inputConfig.about_us
var data = {
"company_name": company,
...
"about_us": about_us
}
let createDocResponse = {}
let request = await fetch(ENDPOINT + CREATE_PATH, {
"method": 'POST',
"documentId": "ID.unique()",
"data": JSON.stringify(data),
"headers": {
'Content-Type': 'application/json',
'X-Appwrite-Response-Format': '1.4.0',
'X-Appwrite-Project': PROJECT_ID
}
});
createDocResponse = await request.json();
console.log(createDocResponse)
And this is the response for the request:
{message: "Param "documentId" is not optional.", code: 400, type: "general_argument_invalid", version: "0.11.8"}
Fetch is supported out of the box on Airtable automations. Not sure how to adapt the client REST call on https://appwrite.io/docs/references/cloud/client-rest/databases. Your guidance, help, insights, will be highly appreciated.
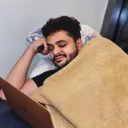
Can you try to use "unique()"
instead of "ID.unique()"
and see if it helps?
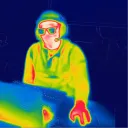
Thank you, @safwan . I already tried it, and also reusing the airtable record id, as "documentId": airtable_record_id. Not working. unique() is detected as a warning. When testing the script, it throws the following error:
ReferenceError: unique is not defined
at main on line 49
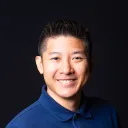
"documentId": "ID.unique()",
is not a valid fetch option. that parameter should be included in the request body.
One easy way to figure out what the fetch request should look like is to open the browser dev tools and switch to the network tab. Then, create a document using the Appwrite Console. The network tab will log the network reqeust for the create document. You can, then, right click and save the request as fetch:
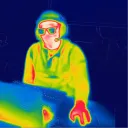
@Steven, the devtools technique is Level God in Steroids, Thank you very much. I'll move the documents id to the request body. 'll be right back.
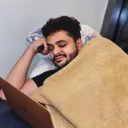
oof damn good catch lol
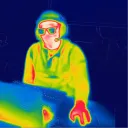
I followed the Steven technique, startinng from a new document on the Appwrite Console. So documentID is the first itemin body, followed by data, as in
"body": "{\"documentId\":\"unique()\",\"data\": {...
I tried ID.unique(), single quotes, no backslash, backslash as escape character, but still
message: "Param "documentId" is not optional." ...
So passing the documentID hasa become the quest for the Holly REST.
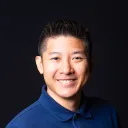
what's the full code?
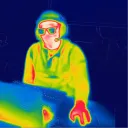
The full code is a bit large:
const record_id = inputConfig.record_id
const contact_owner = inputConfig.contact_owner
const industry_type = inputConfig.industry_type
const show = inputConfig.show
const primary_email = inputConfig.primary_email
const primary_phone = inputConfig.primary_phone
const public_email = inputConfig.public_email
const public_phone = inputConfig.public_phone
const logo = inputConfig.logo
const website = inputConfig.website
const sl_facebook = inputConfig.social_link_facebook
const sl_instagram = inputConfig.social_link_instagram
const sl_linkedin = inputConfig.social_link_linkedin
const sl_twitter = inputConfig.social_link_twitter
const about_us = inputConfig.about_us
var data = {
"company_name": company,
"airtable_record_id": record_id,
"contact_owner": contact_owner,
"industry_type": industry_type,
"primary_email": primary_email,
"primary_phone": primary_phone,
"public_email": public_email,
"public_phone": public_phone,
"logo": logo,
"website": website,
"social_link_facebook": sl_facebook,
"social_link_instagram": sl_instagram,
"social_link_linkedin": sl_linkedin,
"social_link_twitter": sl_twitter,
"about_us": about_us
}
let createDocResponse = {}
let request = await fetch(ENDPOINT + CREATE_PATH, {
"method": "POST",
"headers": {
"Content-Type": "application/json",
"x-appwrite-response-format": '1.4.0',
"x-appwrite-project": PROJECT_ID,
},
"body": {"documentId": "unique()",
"data": data,
"permissions": []},
});
createDocResponse = await request.json();
console.log(createDocResponse)````
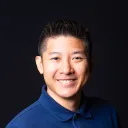
body needs to be a string, not a JSON object. so you can wrap it with JSON.stringify()
to turn it into a string
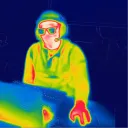
That's what I was adding right now. After stringifying body, the response changed to 401:
"data": data,
"permissions": []}), ```
Now the response is:
``` {message: "The current user is not authorized to perform the requested action.", code: 401, type: "user_unauthorized", version: "0.11.8"} ```
Consider this an advance!
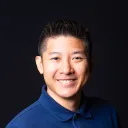
that's a permission problem
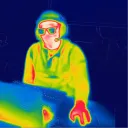
Yes, but at least it's not stuck in the documentId anymore. I'll post the progress, so others trying the same can configure automations between Airtable and Appwrite.
Recommended threads
- JSON and Object Support in Collection do...
I am working with Next.Js and Appwrite Cloud, I am relatively New to Appwrite but i have noticed there is no direct support of JSON and Object support in attrib...
- list() is very slow; eventually shows no...
When I use the web browser to view the collections in my database, the documents they contain are normally displayed within a few seconds. For a few days now, h...
- CSV Not Importing
We don’t seem to having any luck importing a simple .csv file. The import function acts like it’s working but no data imports or is shown in the collection The...
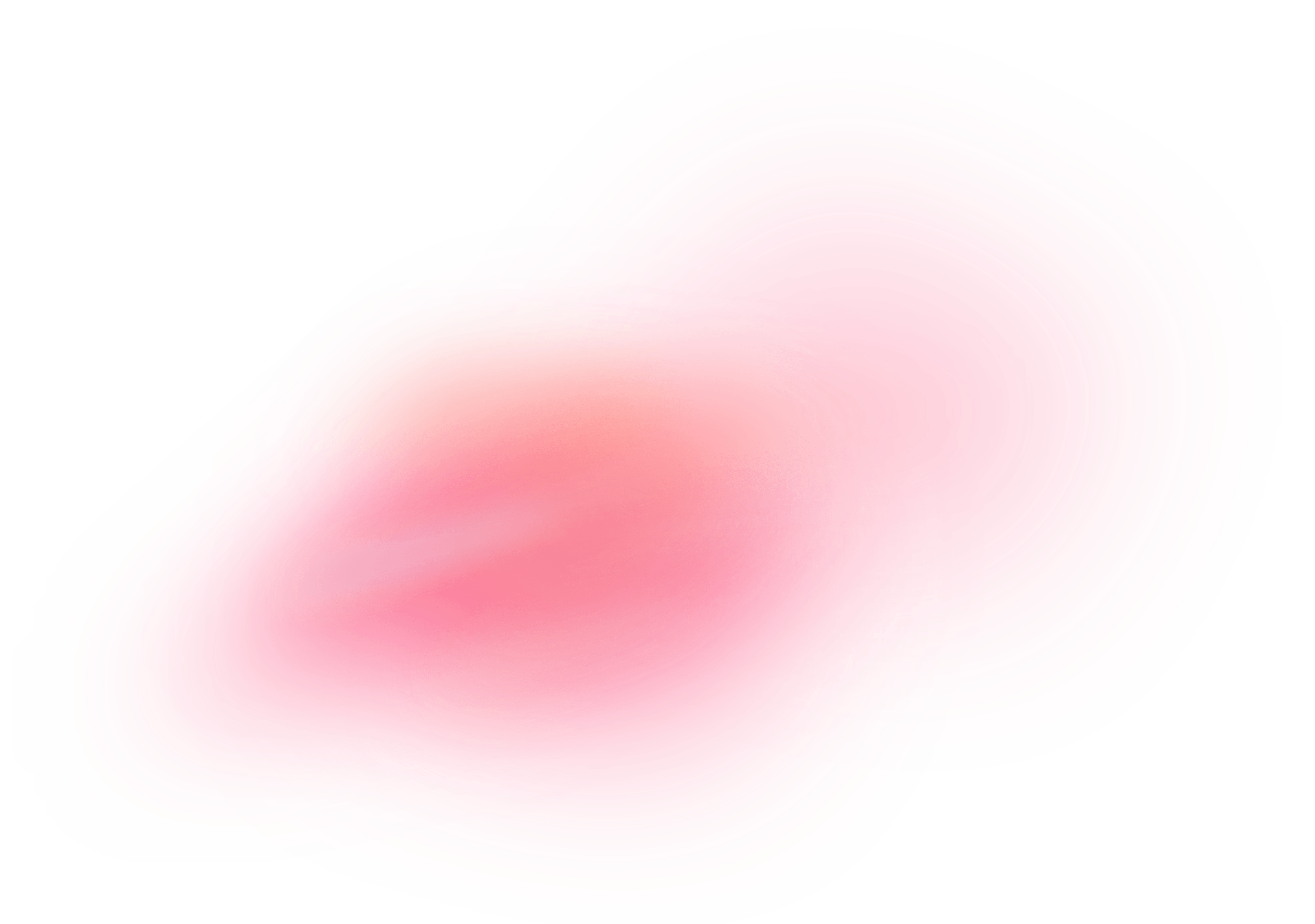