
addMessage() {
const data = {
message: '!!! 2nd message !!!',
sender: this.currentUserId,
};
const promise = this.chat3Service.getRoom(this.roomId).then(room => {
room.messages.push(data);
return this.chat3Service.updateRoom(this.roomId, room);
});
promise.then(
function (response) {
console.log(response); // Success
},
function (error) {
console.log(error); // Failure
}
);
}
Copilot suggesting me to get all data first then push and update the relation again.
Is there any better way to do that ? I was trying to do
addMessage() {
const data = {
message: '!!! 2nd message !!!',
sender: this.currentUserId,
};
const promise = this.chat3Service.updateRoom(this.roomId, {
messages: [...data], // error warning this line
});
promise.then(
function (response) {
console.log(response); // Success
},
function (error) {
console.log(error); // Failure
}
);
}
but i am getting following error
Type '{ message: string; sender: string; }' must have a '[Symbol.iterator]()' method that returns an iterator.ts(2488)
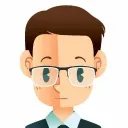
What is the messages
attribute?
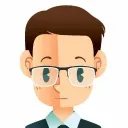
This line
messages: [...data],
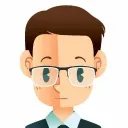
Won't work as []
is array and data
is an object
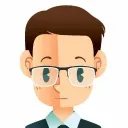
Then you need to go a different way on it.

messages: One-to-Many Relationship to another collection which named messages
{
message: string,
seen: boolean
}
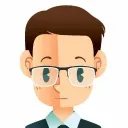
First you'll need to fetch the current messages Then, you need to stringify the new Data and append it Something like so
const promise = this.chat3Service.updateRoom(this.roomId, {
messages: [...prev.message, JSON.stringify(data)], // error warning this line
});

Everytime pushing new messages, should i fetch all prev.messages ? Without fetching, can i do it ? any idea
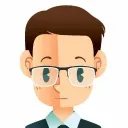
As of now, there is no way to update just re-fetch 😦 Maybe changing the logic to message collection with the chat (or user) id for the relationship

actually, as you see, its pointing to an id from messages collection, but in docs, if i want to update relational documents, i understand to update parent documents with new obj which is
{
message: string,
seen: boolean
}
doc ref: https://appwrite.io/docs/databases-relationships#create-nested
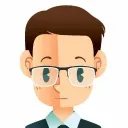
Got you
But messages: [...data],
won't work in, javascript, what you can try to do is something like this
messages:[
...current.messages
{...data}
]
Recommended threads
- Query Appwrite
Hello, I have a question regarding Queries in Appwrite. If I have a string "YYYY-MM", how can I query the $createdAt column to match this filter?
- Different appwrite IDs are getting expos...
File_URL_FORMAT= https://cloud.appwrite.io/v1/storage/buckets/[BUCKET_ID]/files/[FILE_ID]/preview?project=[PROJECT_ID] I'm trying to access files in my web app...
- Invalid document structure: missing requ...
I just pick up my code that's working a week ago, and now I got this error: ``` code: 400, type: 'document_invalid_structure', response: { message: 'Inv...
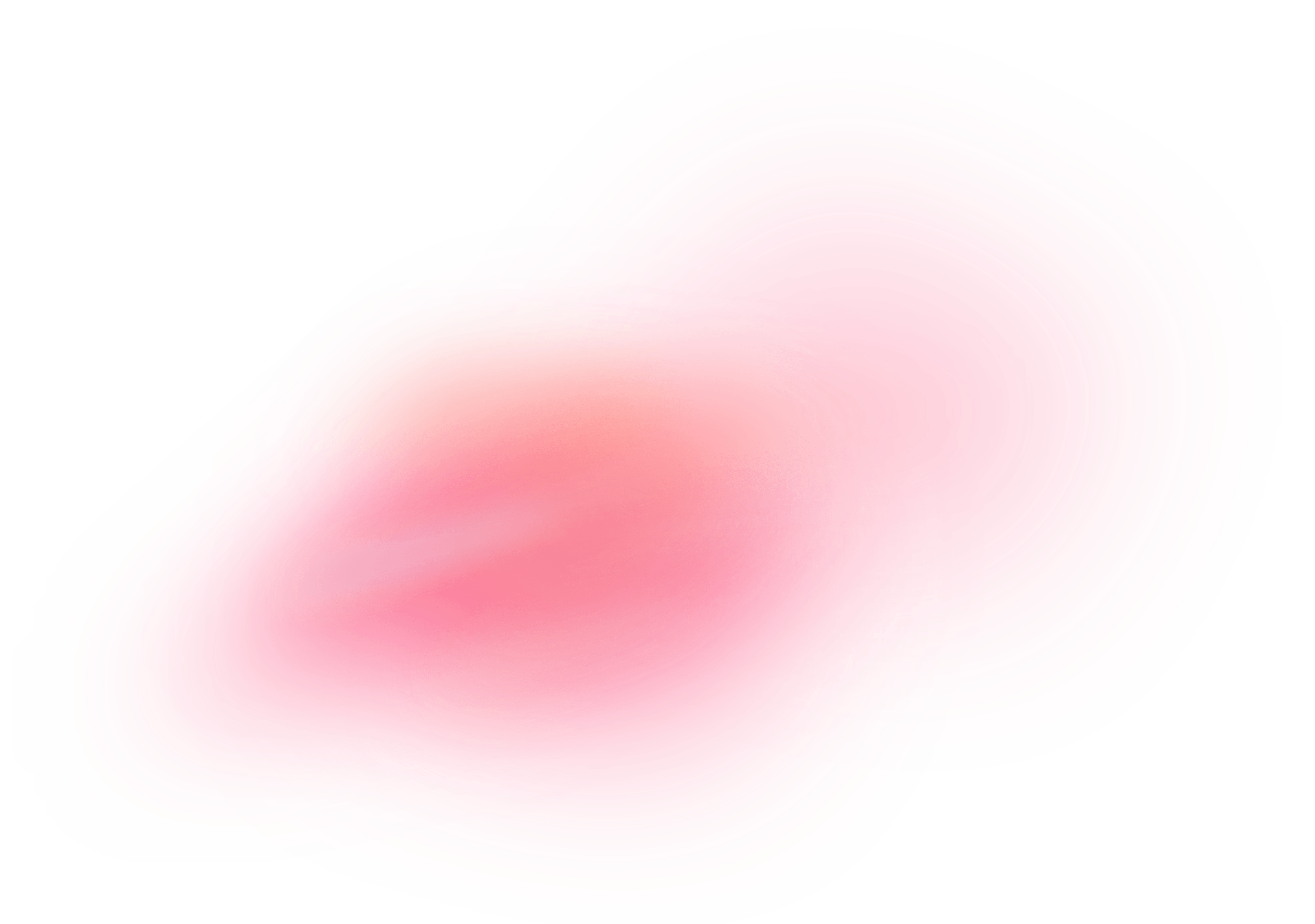