
databases.listDocuments(
environment.appwrite.APP_DATABASE,
environment.appwrite.ROOMS_COLLECTION,
[
Query.equal('members', [userID, currentUserID]),
Query.equal('members', [currentUserID, userID]),
]
);
my collection member attribute is: string[]
I want to either Query.equal('members', [userID, currentUserID])
, OR Query.equal('members', [currentUserID, userID])
,
not AND
Thanks!
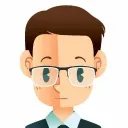
As of now you can't use logical or in queries , Make sure to check this feature request https://github.com/appwrite/appwrite/issues/2740

Yesterday i met with appwrite first time and came to the limits.. sad story. Do you have any ideas about how I can go around?
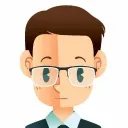
Yes You have two solutions
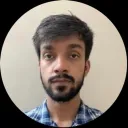
U can do this but the drawback is that you are making two request which might increase the latency
const result1 = await databases.listDocuments( environment.appwrite.APP_DATABASE, environment.appwrite.ROOMS_COLLECTION, [Query.equal('members', [userID, currentUserID])] );
const result2 = await databases.listDocuments( environment.appwrite.APP_DATABASE, environment.appwrite.ROOMS_COLLECTION, [Query.equal('members', [currentUserID, userID])] );
const combinedResults = [...result1.documents, ...result2.documents];
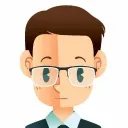
- Fetch the documents twice.
- Create another attribute name id
search
inside that attribute you can put all the userIDs values seprated by space. Then add fulltext index to that attribute and you'll be able to search that field using or logic like so 👇
databases.listDocuments(
environment.appwrite.APP_DATABASE,
environment.appwrite.ROOMS_COLLECTION,
[
Query.search('search', `${userID} ${currentUserID}`),
]
);

when i tried to examine this way, members has attribute of string[] (array) in docs, its written that if members has currentUserID OR userID then it will return the doc.
in my scenario, it is an array thats why it returns empty array when i tried everything manually.
const res1 = this.appwrite.listDocuments(environment.appwrite.ROOMS_COLLECTION,
[
Query.equal('members', ['Xvxuht5IIBPcV8bN1Q1UUhyYeil2', 'JNEB25J6hsdKjnHMOiZfuvQJ5dh2']),
]);
const res2 = this.appwrite.listDocuments(environment.appwrite.ROOMS_COLLECTION,
[
Query.equal('members', ['JNEB25J6hsdKjnHMOiZfuvQJ5dh2', 'Xvxuht5IIBPcV8bN1Q1UUhyYeil2']),
]);
return Promise.all([res1, res2]).then((values) => {
console.log(values);
return [...values[0].documents, ...values[1].documents];
});
console
[
{
"total": 0,
"documents": []
},
{
"total": 0,
"documents": []
}
]
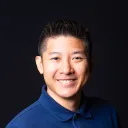
uhh why not have one query wiht all the member values 🧐 That's the only OR we support at the moment

[SOLVED] How can i write OR query to match an array?

The solution is not to use OR query like i tried first post.
I used AND query with Query.search
ofc after full-text index.
databases.listDocuments(
environment.appwrite.APP_DATABASE,
environment.appwrite.ROOMS_COLLECTION,
[
Query.search('users', cUserId),
Query.search('users', userId)],
]
);
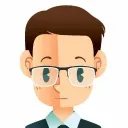
In this case you don't need to have two search
function.
You can have one with iser id's separate with a space

Really? in lib, search
function accepts only one attribute and value
(property) Query.search: (attribute: string, value: string) => string
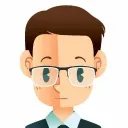
I know. But this string can have spaces in it like you can see here
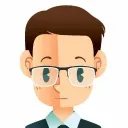

do you mean?
[Query.search('users', `${cUserId} ${userId}`)]
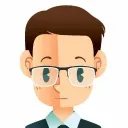
Yes

it works! Thanks a lot

small update: i found a bug in my app, that wanted to share it here, related topic
this implementation does not work, it returns when cUserId
found, not care about userId
.
i want both of the array contains cUserId
AND userId
[
Query.search('users', cUserId),
Query.search('users', userId)
]```
for now, this is the best and working option.
Recommended threads
- Collection Permission issue
I am facing issue in my Pro account. "Add" button is disabled while adding permission in DB collection settings.
- Opened my website after long time and Ba...
I built a website around a year back and and used appwrite for making the backend. At that time the website was working fine but now when i open it the images a...
- Is it possible to cancel an ongoing file...
When uploading a file to storage, is there a way to cancel the upload in progress so the file is not saved or partially stored?
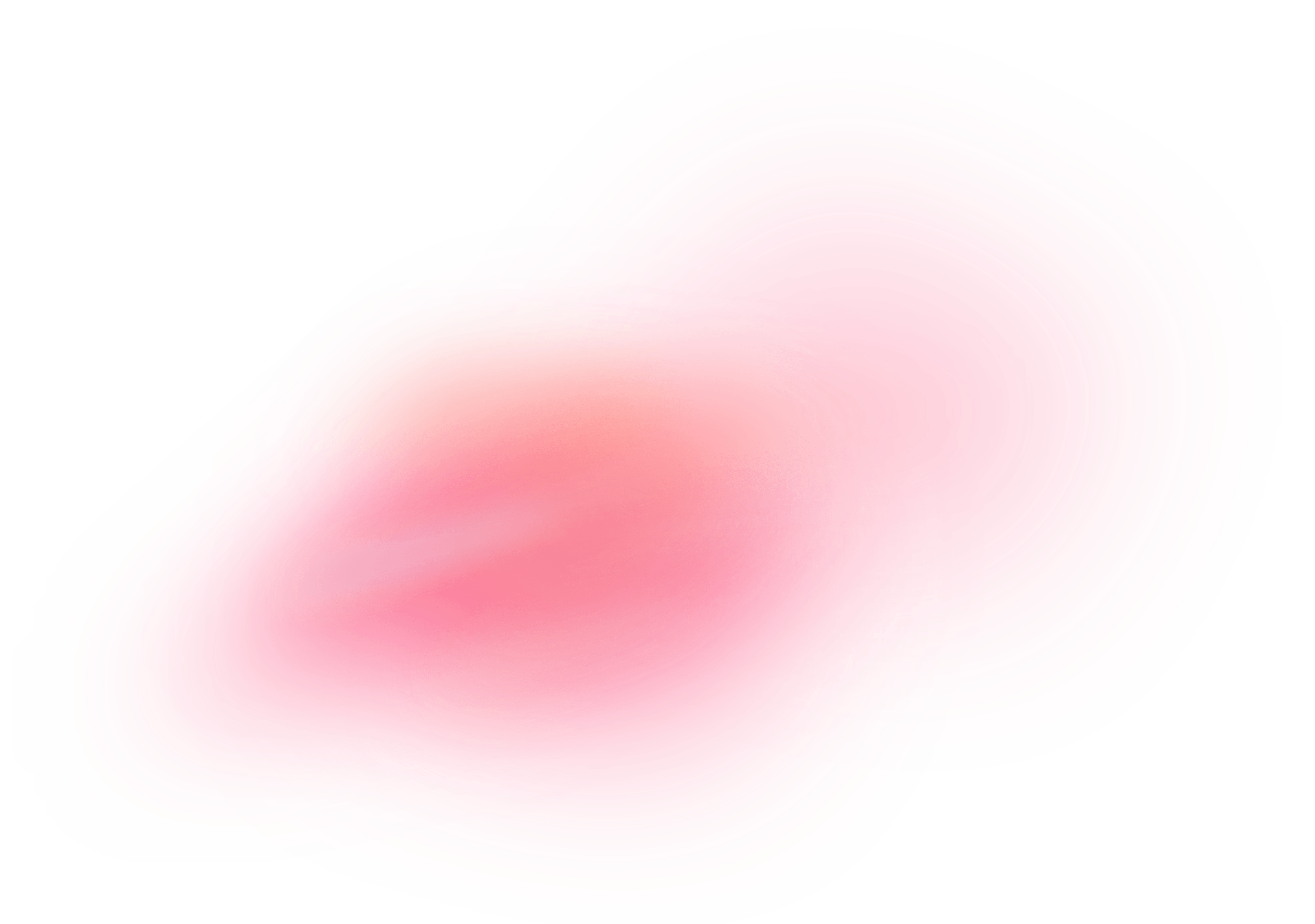