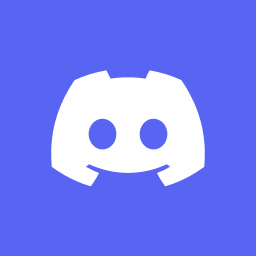
Hello, how can I check if a user is logged in?
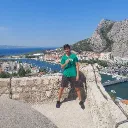
I think that only way is to fetch accou, accout.get()
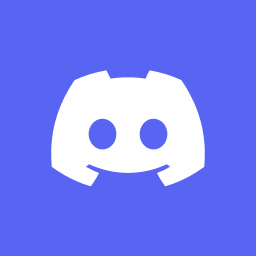
I did it like that, but it always shows the logged in screen, and throws an error
code:
class App extends StatelessWidget {
const App({super.key});
@override
Widget build(BuildContext context) {
bool isUserLoggedIn() {
try {
account().get();
return true;
} catch (error) {
return false;
}
}
Widget homeScreen = isUserLoggedIn() ? LoggedInHomeScreen() : NotLoggedInScreen();
return MaterialApp(
title: 'Outfytr',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: const Color.fromRGBO(113, 100, 231, 1)),
useMaterial3: true,
),
home: homeScreen,
);
}
}
class LoggedInHomeScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Logged In'),
),
body: Center(
child: Text('Logged In'),
),
);
}
}
class NotLoggedInScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Not Logged In'),
),
body: Center(
child: Text('Not Logged In'),
),
);
}
}```
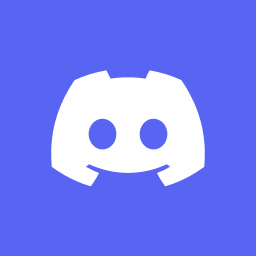
error:
Error: AppwriteException: general_unauthorized_scope, User (role: guests) missing scope (account) (401)
dart-sdk/lib/_internal/js_dev_runtime/private/ddc_runtime/errors.dart 288:49 throw_
packages/appwrite/src/client_mixin.dart 73:9 prepareResponse
packages/appwrite/src/client_browser.dart 214:14 call
dart-sdk/lib/_internal/js_dev_runtime/patch/async_patch.dart 45:50 <fn>
dart-sdk/lib/async/zone.dart 1661:54 runUnary
dart-sdk/lib/async/future_impl.dart 147:18 handleValue
dart-sdk/lib/async/future_impl.dart 784:44 handleValueCallback
dart-sdk/lib/async/future_impl.dart 813:13 _propagateToListeners
dart-sdk/lib/async/future_impl.dart 584:5 [_completeWithValue]
dart-sdk/lib/async/future_impl.dart 657:7 callback
dart-sdk/lib/async/schedule_microtask.dart 40:11 _microtaskLoop
dart-sdk/lib/async/schedule_microtask.dart 49:5 _startMicrotaskLoop
dart-sdk/lib/_internal/js_dev_runtime/patch/async_patch.dart 177:15 <fn>```
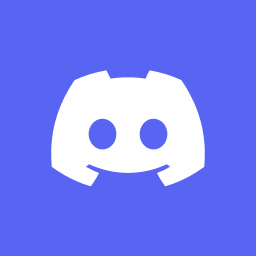
pls help

Yes, it's normal for it to throw an error if there is no logged-in user. You should handle the exception
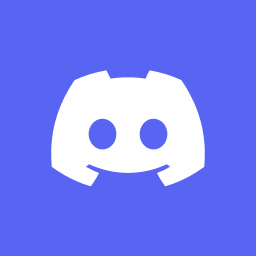
and how is that possible?
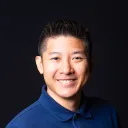
- you need to
await account.get()
- you shouldn't be calling that in the build step. perhaps you need to go through some more Flutter tutorials to learn how to structure an app
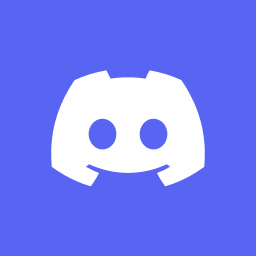
in the second step you mean my bool function right?
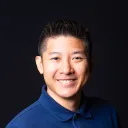
sort of. you can't/shouldn't be calling async methods in build

Oh, I forgot about await. Anyway, I agree with Steven. Here is a brief sample, but you should use StatefulWidget instead and call your function within initState. (This is not a good practice. I recommend using a proper state management.)
Future<bool> isUserLoggedIn() async {
//---------------------------------
try {
await appApi.getAccount.get();
return true;
} on AppwriteException catch (_) {
return false;
}
}
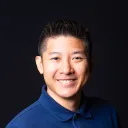
ya, and you'd need local state to update the UI accordingly with the result of isUserLoggedIn
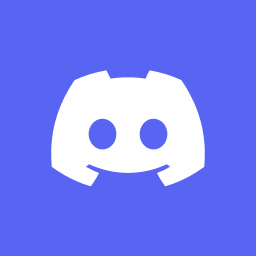
works thx!
Recommended threads
- Changing password when logged using a OT...
I'm developping in flutter/dart, I'm using email token to login to an existing user: _sessionToken = await account.createEmailToken(userId: app.ID.unique(), ema...
- Simplest Appwrite + Riverpod + Go_router...
Hey, I managed to get native Google Sign-In working with Appwrite custom tokens and Riverpod/GoRouter, and I’m stuck on three things: --- ## 1) SDK user ident...
- Invalid URI. Register your new client on...
Hello everyone, I've had recovery password flow implemented for about over a year and I've tested it on both test and production projects. Recently one of my us...
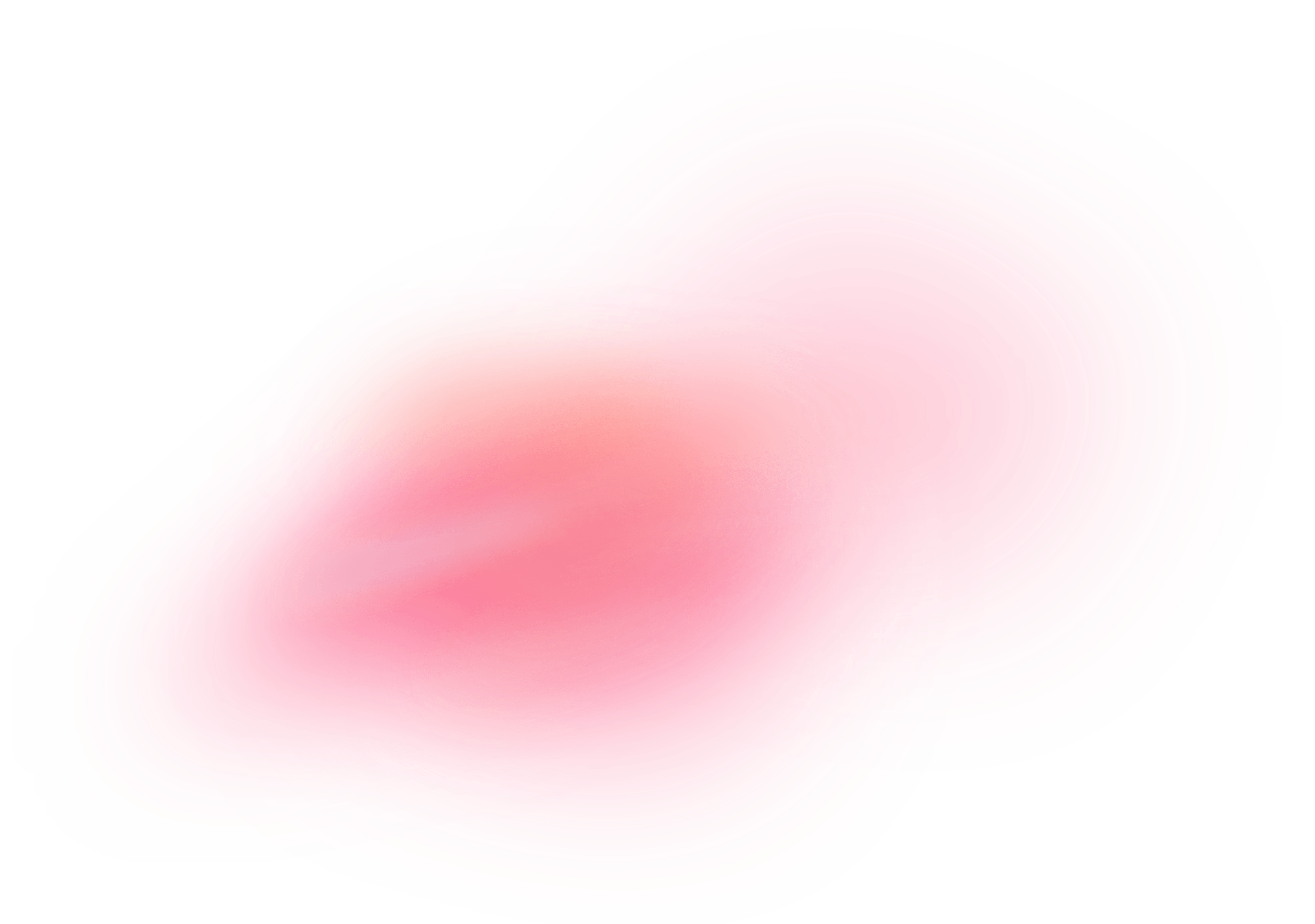