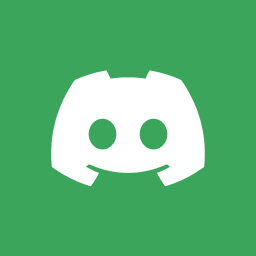
I am using appwrite 1.3.7 , when writing functions in dart , the function not executing "print" commands inside "if" statements or after".then" or in functions other than "start" function
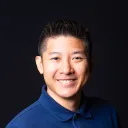
your function might be returning before those then callbacks are being executed. it would help if you shared your actual code
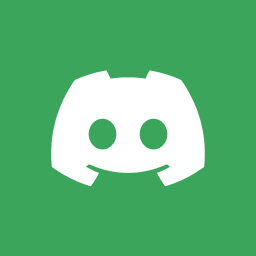
import 'dart:convert';
import 'package:dart_appwrite/dart_appwrite.dart';
import 'car_model.dart';
Future<String?> isAdvertiserExists({required String userId, required Databases db}) async {
String? res;
try {
db.listDocuments(
databaseId: "VipcarDatabaseV3",
collectionId: "Advertiser",
queries: [
Query.equal('user_id', [userId]),
]).then((adv){
if(adv.documents.isNotEmpty){
res= adv.documents[0].$id;
print("Document id : ${adv.documents[0].$id}");
}else{
print("Document not found");
res = null;
}
});
} catch (e) {
print(e.toString());
print("isAdvertiserExists function error");
}
return res;
}
Future<void> start(final req, final res) async {
final client = Client();
final database = Databases(client);
if (req.variables['APPWRITE_FUNCTION_ENDPOINT'] == null ||
req.variables['APPWRITE_FUNCTION_PROJECT_ID'] == null ||
req.variables['APPWRITE_FUNCTION_API_KEY'] == null) {
print(
"Environment variables are not set. Function cannot use Appwrite SDK.");
} else {
client
.setEndpoint(req.variables['APPWRITE_FUNCTION_ENDPOINT'])
.setProject(req.variables['APPWRITE_FUNCTION_PROJECT_ID'])
.setKey(req.variables['APPWRITE_FUNCTION_API_KEY'])
.setSelfSigned(status: true);
}
final String appUserId = req.variables["APPWRITE_FUNCTION_USER_ID"];
bool resultCode = true;
String message = "ok";
bool? adverOk;
late CarObject car;
try {
car = CarObject.fromJson(jsonDecode(req.payload));
print(car.carCategoryId);
} catch (e) {
print(e.toString());
}
car.advertiserId = appUserId;
isAdvertiserExists(userId: appUserId, db: database).then((res) {
if (res != null) {
adverOk = true;
} else {
adverOk = false;
}
});
res.json({
"code": resultCode,
"message": message,
"attr": adverOk,
});
}
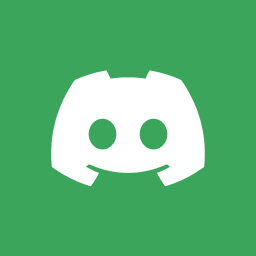
In this function i am receiving data from user then define model for that data, then trying to find the document that has user id as attribute.
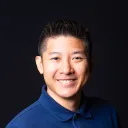
You have to await the list documents call
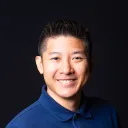
You should probably read into how futures work some more too to get a better understanding of how they work
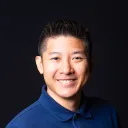
In short, the res.json() is called and your function returns before any of the thens get executed
Recommended threads
- Error 1.7.4 console team no found
In console when i go to auth, select user, select a membership the url not work. Only work searching the team. It is by the region. project-default- and i get ...
- Is Quick Start for function creation wor...
I am trying to create a Node.js function using the Quick Start feature. It fails and tells me that it could not locate the package.json file. Isn't Quick Start ...
- functions of 1.4 not work on 1.7
Hi, i updated of 1.4 to 1.7 but the function not work i get it error. Do I need to build and deploy the functions again?
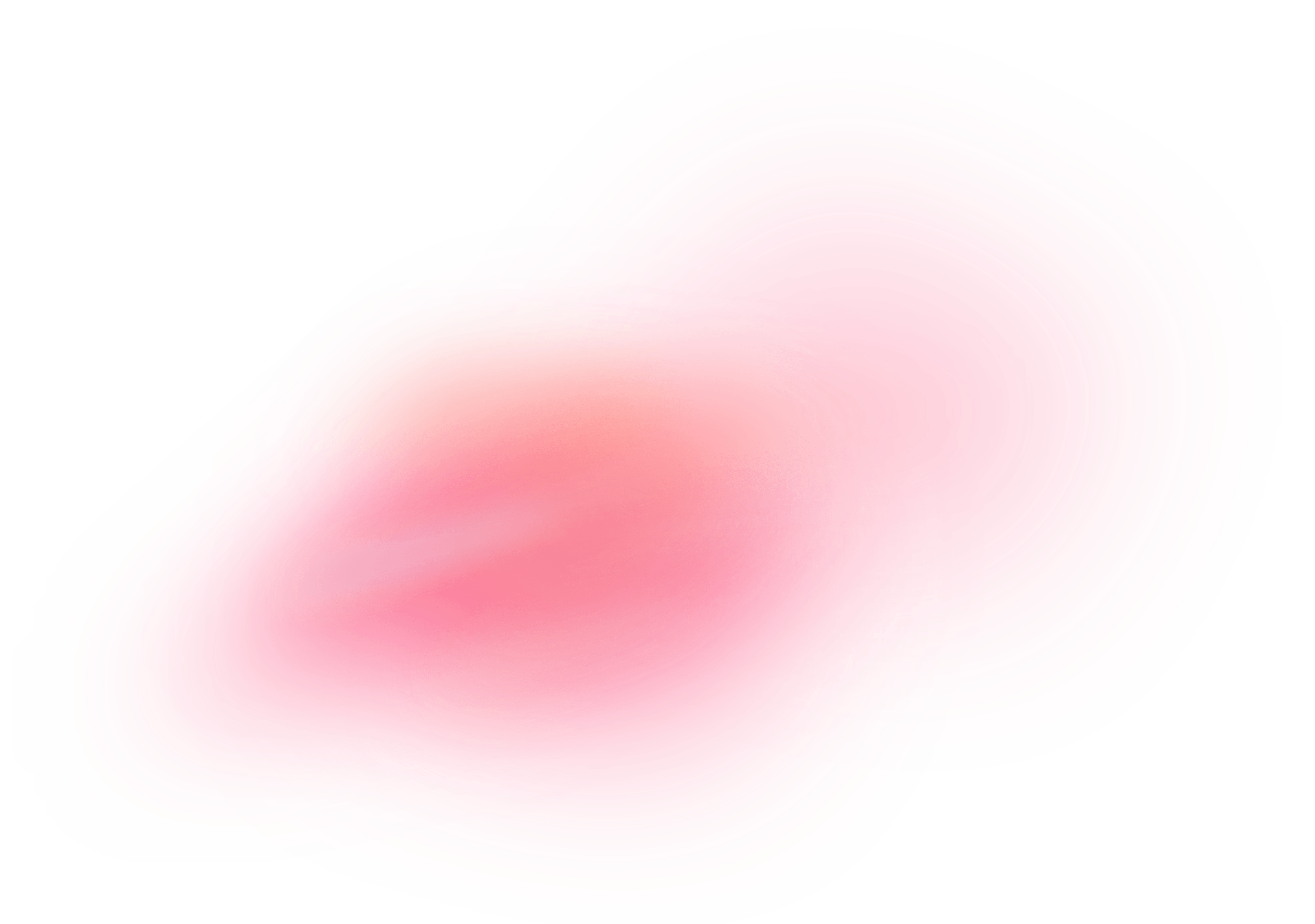