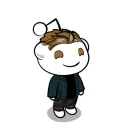
I'm trying to get data from the database but I've always a generic runtime error, I've tried with php code but I don't find where I'm wrong
The code is on the screenshot
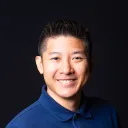
would you please try to wrap your code with a try/except to catch any exception?
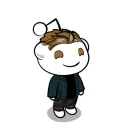
Tried but when I test I got only runtime error
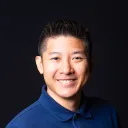
what's your updated code?
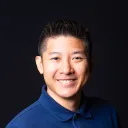
copying and pasting here would be easier
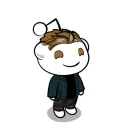
thank you for waiting
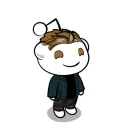
import os import json
from appwrite.client import Client from appwrite.services.databases import Databases from appwrite.services.users import Users from appwrite.id import ID from appwrite.query import Query
def main(req, res): payload = json.loads(req.variables.get('APPWRITE_FUNCTION_EVENT_DATA')) client = Client() client.set_endpoint(req.variables.get("APPWRITE_ENDPOINT")) client.set_project(req.variables.get("APPWRITE_PROJECT_ID")) client.set_key(req.variables.get("APPWRITE_API_KEY"))
#Get Users collection id
database_id = req.variables.get('DATABASE_ID')
users_collection_id = req.variables.get('USERS_COLLECTION_ID')
database = Databases(client)
users = Users(client)
db_result = users.get(payload['userId'])
try:
result = database.get_document(database_id, users_collection_id, payload['userId'])
if result is None:
database.create_document(
database_id,
users_collection_id,
ID.custom(id=payload['userId']),
data={
'userId': payload['userId'],
'name': db_result['name'],
'email': db_result["email"],
})
except Exception as e:
print(e)
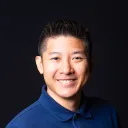
FYI, it's best to wrap code in backticks to format a bit nicer. You can use 1 backtick for inline code (https://www.markdownguide.org/basic-syntax/#code) and 3 backticks for multiline code (https://www.markdownguide.org/extended-syntax/#syntax-highlighting.
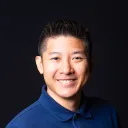
Would you please make sure res.json()
or res.send()
is called exactly once before exiting?
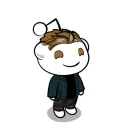
import os
import json
from appwrite.client import Client
from appwrite.services.databases import Databases
from appwrite.services.users import Users
from appwrite.id import ID
from appwrite.query import Query
def main(req, res):
payload = json.loads(req.variables.get('APPWRITE_FUNCTION_EVENT_DATA'))
client = Client()
client.set_endpoint(req.variables.get("APPWRITE_ENDPOINT"))
client.set_project(req.variables.get("APPWRITE_PROJECT_ID"))
client.set_key(req.variables.get("APPWRITE_API_KEY"))
#Get Users collection id
database_id = req.variables.get('DATABASE_ID')
users_collection_id = req.variables.get('USERS_COLLECTION_ID')
database = Databases(client)
users = Users(client)
db_result = users.get(payload['userId'])
try:
result = database.get_document(database_id, users_collection_id, payload['userId'])
if result is None:
database.create_document(
database_id,
users_collection_id,
ID.custom(id=payload['userId']),
data={
'userId': payload['userId'],
'name': db_result['name'],
'email': db_result["email"],
})
except Exception as e:
print(e)
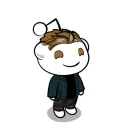
Thank you I didn't remember it
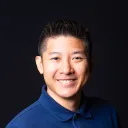
did that solve your problem?
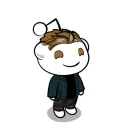
Nope, in that message I'm referring to markdownπ
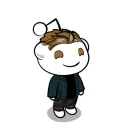
I tried again with same code but I hadn't any logs, nothing, I can't find where is the problem in the code
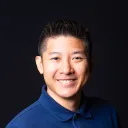
what's your updated code?
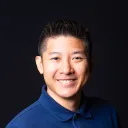
also, try to only print strings so: print(str(e))
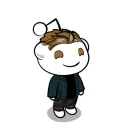
Nothing, now because the try catch it says completed but no response are recorded
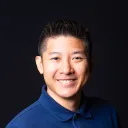
what's your updated code?
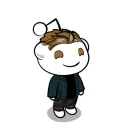
Same only with the change from print(e) to print(str(e))
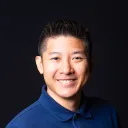
Would you please make sure res.json()
or res.send()
is called exactly once before exiting?
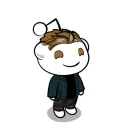
res.json
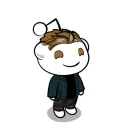
Now with the same exactly code, I got the result
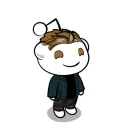
Maybe I miswrite a character, idk
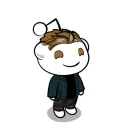
Thank you for help!
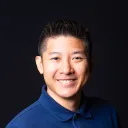
[SOLVED] Error with Python SDK database.get_document
Recommended threads
- How to Fetch Item and Include the User/A...
A typical workflow with ORMs is to, for example, fetch an item from the DB and include the user who created it. How would I do that with AppWrite? Is that easil...
- Unable to add permission when creating a...
I am creating a collection from a cloud function using the Appwrite Dart server SDK, and I want to add permissions so it can be accessed by users. I added code ...
- Resource limit on the free plan
I'm currently building a health-focused tech project as part of a student initiative, and Iβve been using Appwrite extensively for backend services. As part o...
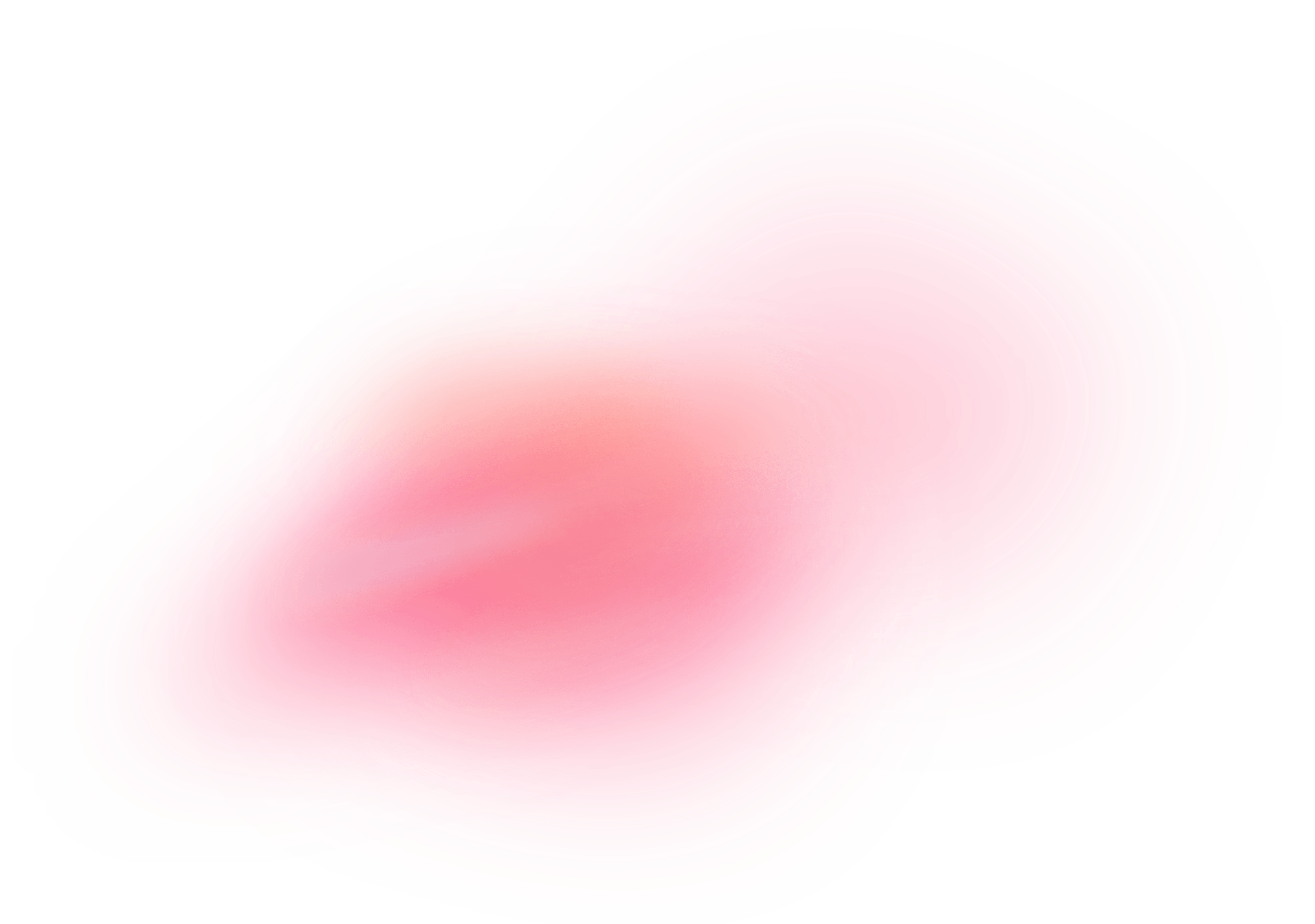