
Hi! I (ChatGPT xD) have created an appwrite function with node.js, which looks like this:
const sdk = require("node-appwrite");
const stripe = require("stripe");
module.exports = async function (req, res) {
const client = new sdk.Client();
const stripeClient = stripe(req.variables["STRIPE_SECRET_KEY"]);
if (
!req.variables["APPWRITE_FUNCTION_ENDPOINT"] ||
!req.variables["APPWRITE_FUNCTION_API_KEY"]
) {
console.warn(
"Environment variables are not set. Function cannot use Appwrite SDK."
);
} else {
client
.setEndpoint(req.variables["APPWRITE_FUNCTION_ENDPOINT"])
.setProject(req.variables["APPWRITE_FUNCTION_PROJECT_ID"])
.setKey(req.variables["APPWRITE_FUNCTION_API_KEY"])
.setSelfSigned(true);
}
let data = req.payload;
try {
let event = stripeClient.webhooks.constructEvent(
data,
req.headers["stripe-signature"],
"your-stripe-webhook-secret"
);
if (event.type === "checkout.session.completed") {
let session = event.data.object;
let user_id = session.metadata.user_id;
console.log(
`Payment for user ${user_id} was successful.`
);
//Here, a database update is going to be made to mark as successful
}
res.send("Webhook handled successfully.", 200);
} catch (err) {
console.error(`Failed to handle webhook: ${err}`);
res.send("Webhook handling failed.", 500);
}
};
And I created a webhook in stripe, that should create an execution on that function (Reference: Image). This webhook triggers on the checkout.session.complete event. So when I trigger the event of a successful payment, I get the following error: (Next message)

{
"message": "Invalid data: Value must be a valid string and at least 1 chars and no longer than 8192 chars",
"code": 400,
"type": "general_argument_invalid",
"version": "1.3.8"
}

The problem is:

The data that stripe sends is not at all 8192 characters long. In fact, it's around 2k. I don't have any idea how to fix this

According to stripe, this is what their request to the endpoint (my function) is:

Stripe webhook to appwrite function. Error general_argument_invalid

So I maybe found the problem: In this (https://dev.to/appwrite/start-selling-online-using-appwrite-and-stripe-3l04) older official article, it says: "Looking at Appwrite documentation, it expects a JSON body where all data is stringified under the data key. On the other hand, looking at Stripe documentation, it sends a webhook with all data in the root level as a JSON object.
Alongside this schema miss-match, Appwrite also expects some custom headers (such as API key), which Stripe cannot send. This problem can be solved by a simple proxy server that can properly map between these two schemas, and apply authentication headers. "
It also says to this: "You can expect official implementation from Appwrite itself, but as of right now, you can use Meldironβs Appwrite webhook proxy. "

But because this article is quite old, I am asking:

Is there an official implementation from Appwrite? The repository also seems like it's already a bit old (1 year ago there was the last update)

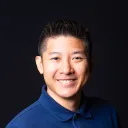
This one is probably better: https://github.com/BoolCode/appwrite-funcover
Also, this proxy won't be needed in the next version of Appwrite

Ah okay, thank you, will use that for now.
Looking forward to v 1.4 :)

So, I have set up funcover, but I cannot get around this error:
root@appwrite:~/appwrite# docker logs funcover -f
Request: POST /v1/functions/webhook/executions
content-type: application/json
x-appwrite-project: v1
Connection: keep-alive
User-Agent: Bun/0.6.13
Accept: */*
Host: appwrite
Accept-Encoding: gzip, deflate
Content-Length: 18
Response: < 404 Not Found
< X-Debug-Fallback: true
< Cache-Control: no-cache, no-store, must-revalidate
< Expires: 0
< Pragma: no-cache
< X-Debug-Speed: 0.006770133972168
< Content-Type: application/json; charset=UTF-8
< Server: swoole-http-server
< Connection: keep-alive
< Date: Sat, 12 Aug 2023 11:19:46 GMT
< Content-Length: 182
< Content-Encoding: gzip
When I try to access the function with: https://myAppwriteDomain.com/v1/webhook

I don't have any idea why it keeps thinking the project id is v1

My docker-compose.yml
:

And my .env
:

nvm, my docker-compose.yml was wrong

I had to add PATH_INSTEAD_OF_DOMAIN and PATH_PREFIX to the docker-compose.yml, not the .env
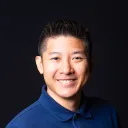
[SOLVED} Stripe webhook to appwrite function. Error general_argument_invalid
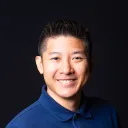
[SOLVED] Stripe webhook to appwrite function. Error general_argument_invalid
Recommended threads
- GitHub connection failed
I am running a self-hosted Appwrite with Coolify. Everything is running smoothly so far and I wanted to starte develop functions. Trying to connect github with ...
- create subdomain for function
I have deployed my react project on Appwrite using Sites feature. I have added custom DNS on namecheap. I want to create new sub domains for my functions on Ap...
- Appwrite CLI
I try to deploy a function using Appwrite CLI but it says: `appwrite functions createDeployment ^ --functionId=xyz^ --code="." ^ --activate error: u...
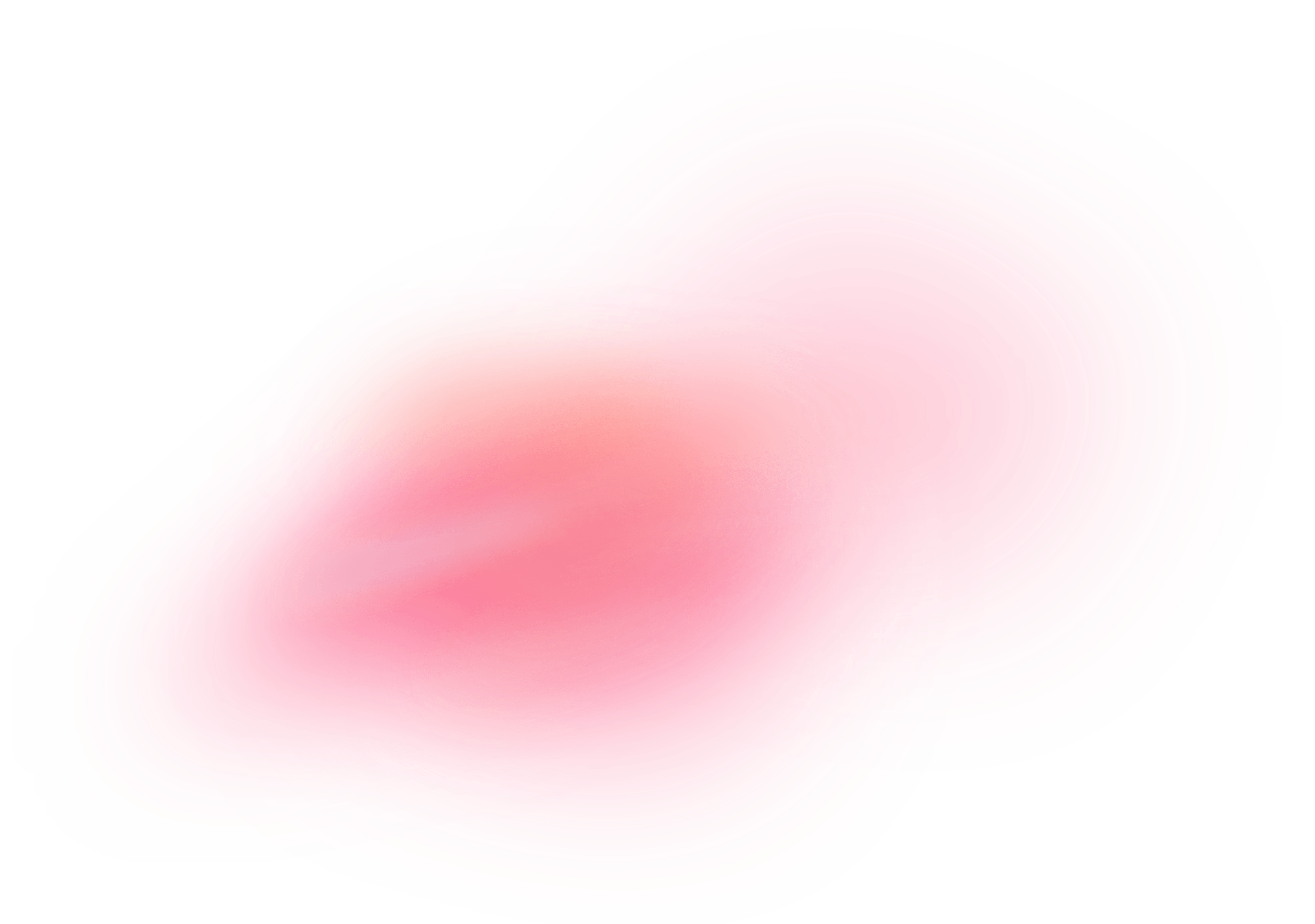