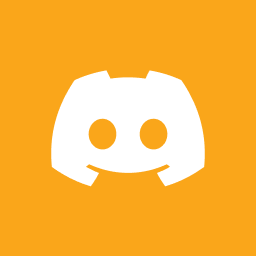
Hello everyone,
I have a question about an example I copied from here: https://github.com/saimon24/flutter-appwrite-auth.
I would like to access the same AuthAPI class in other classes, for example to get the UserId. What is the easiest way to do this without saving data?
Just started with Flutter and can't really find anything about it (maybe I'm just searching wrong).
Thanks! Greeting, Manuel
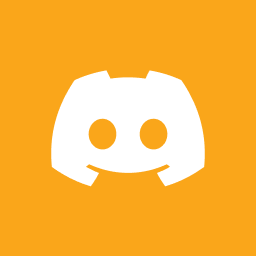
Question about class access for authentication (Flutter)
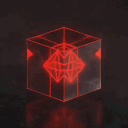
Hello !
From the code, I'd say you can retrieve the existing AuthAPI instance with this line final AuthAPI appwrite = context.read<AuthAPI>();
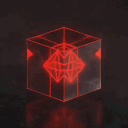
You can retrieve the user id with this code:
final AuthAPI appwrite = context.read<AuthAPI>();
appwrite.userid // <-- retrieve user id from AuthAPI instance
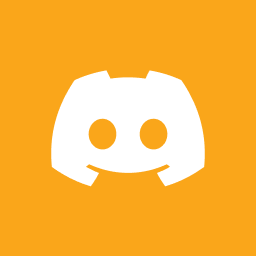
Hi, first of all, thank you for your quick reply. But if I try your solution in this code:
class EditProfilePage extends StatefulWidget {
const EditProfilePage({Key? key}) : super(key: key);
@override
State<EditProfilePage> createState() => _EditProfilePageState();
}
class _EditProfilePageState extends State<EditProfilePage> {
bool _isLoading = true;
final AuthService authService = context.read<AuthService>();
String userId = authService.currentUser.$id;
UserProfile? user;
UserService userService = UserService();
@override
void initState() {
super.initState();
_getData();
}
_getData() async {
user = await userService.fetchCurrentUser(userId);
_isLoading = false;
}
@override
Widget build(BuildContext context) {
.....```
The error message:
``The instance member 'context' can't be accessed in an initializer.
Try replacing the reference to the instance member with a different``
is displayed
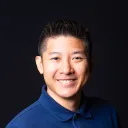
It needs to go in initState
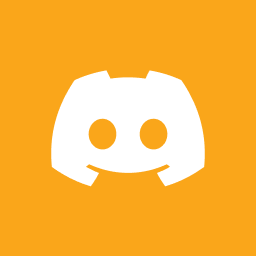
Ok so it has to look something like this?
class _EditProfilePageState extends State<EditProfilePage> {
bool _isLoading = true;
late AuthService authService;
late String userId;
late UserProfile user;
UserService userService = UserService();
@override
void initState() {
super.initState();
authService = context.read<AuthService>();
userId = authService.currentUser.$id;
}
_getData() async {
user = await userService.fetchCurrentUser(userId);
_isLoading = false;
}```
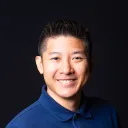
btw it's best to use 3 backticks for multiline code
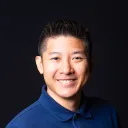
and you can also add dart
after the first 3 backticks to get syntax highlighting 😉
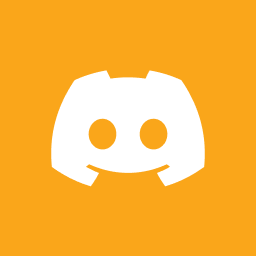
@Steven thank you for your help. But I see the CircularProgressIndicator (only if _isLoading == true) the hole time although the data is arrived in the Service class 😮
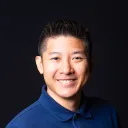
what's the rest of yoru code? what's the result of fetchCurrentUser()
?
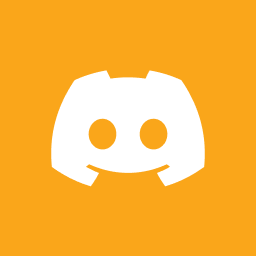
This is the Widget build:
@override
Widget build(BuildContext context) {
_getData();
return Scaffold(
drawer: SideNavDrawer(),
appBar: buildAppBar(context, "Profil bearbeiten"),
body: _isLoading
? const Center(
child: CircularProgressIndicator(),
)
: ListView(
....```
The answer from ``fetchCurrentUser`` is the UserProfile entity.
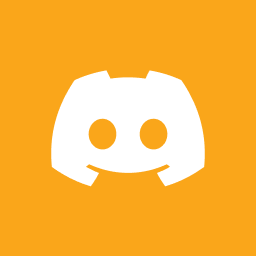
The fetchCurrentUser function:
Future<UserProfile> fetchCurrentUser(String id) async {
final docmentList = await db.listDocuments(
databaseId: APPWRITE_DATABASE_ID,
collectionId: COLLECTION_USER,
queries: [Query.equal("user_id", id)]);
print("Done");
return docmentList.documents
.map((e) => UserProfile.fromMap(e.data))
.toList()[0];
}```
And the ``print("Done")`` is very fast in the debug console.
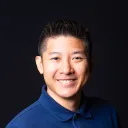
so the problem with this is there is nothing telling Flutter that _isLoading
has been updated and that it needs to re-render.
I recommend finding some additional tutorials on flutter and state. both of the problems you raised are flutter topics and not appwrite specific
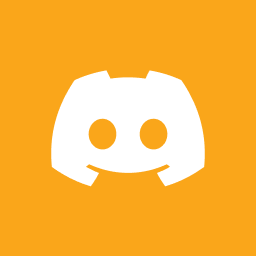
Thanks for your help 😉
Recommended threads
- I am facing this error: type 'Null' is ...
When attempting to fetch areas from the area collection, the application throws an error: "type 'Null' is not a subtype of type 'int.'" This issue originates in...
- Adding Domain to Sites [Self Hosted]
I am struggling to get this working. I stood-up a new server and deployed appwrite 1.7.4. I added update .env file _APP_DOMAIN=appwrite.mydomain.com _APP_DOMAI...
- Cloud DB
Hey guys, my entire database has gone missing. Project id 665ae18f002aac268b73
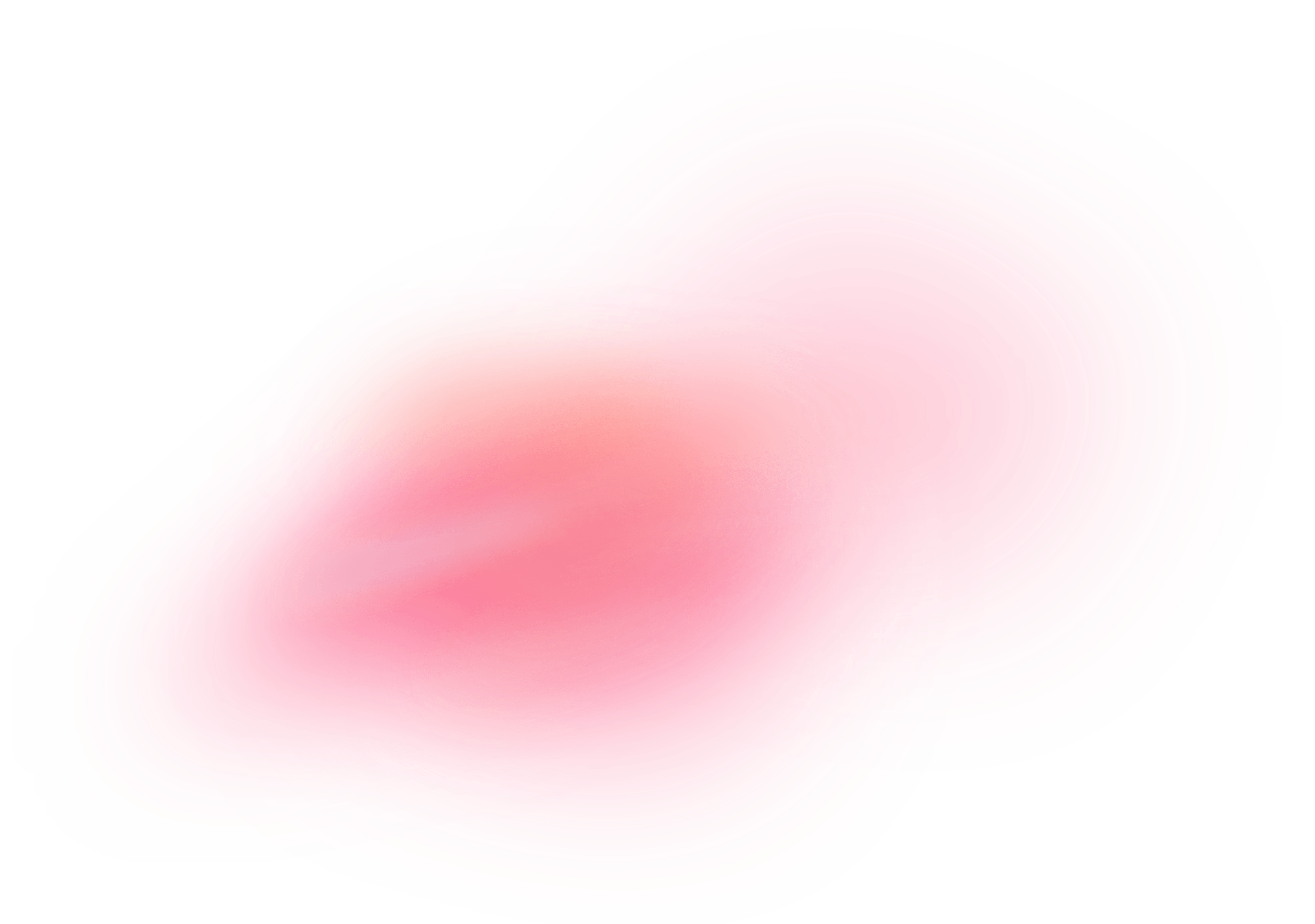