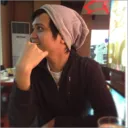
I am trying to send an email verification when a user registers on my project but I am getting the following error:
{
message: 'User (role: guests) missing scope (account)',
code: 401,
type: 'general_unauthorized_scope',
version: '1.3.8'
}
At this point the user isn't logged in yet as they have just submitted their registration form:
try {
const userAccount = await account.create(ID.unique(), email, password, `${firstname} ${lastname}`);
await account.createVerification(Constants.BASE_URL);
res.status(StatusCodes.OK).json(userAccount);
} catch (error: any) {
res.status(StatusCodes.INTERNAL_SERVER_ERROR).json(error);
}
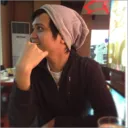
@Steven any idea why it's telling me I am not logged in when trying to send the verification email to a user who just registered to my project?
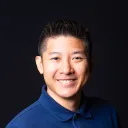
please don't tag people just because you need help as it can be disruptive. just post and wait
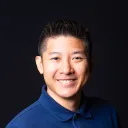
This error means there is no session (the user is not logged in).
Looking at the code you shared, you created an account, but you didn't create a session
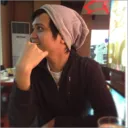
Looking at this page: https://gist.github.com/eldadfux/2eea9df7cc6dc18b63955dd8b10ad758
There's a quoted text that says
By default, unverified users are not restricted in any special way. It's up to you and your app logic to decide how these users are treated. You can prompt them with a verification message or limit their access to your application.
It does mention that users need to be logged in before calling createVerification
but what if I want to control access so that only verified accounts are able to log in?
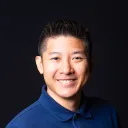
It does mention that users need to be logged in before calling createVerification but what if I want to control access so that only verified accounts are able to log in?
You would do that with permissions; limit access to resources to verified users. See https://appwrite.io/docs/permissions#permission-roles
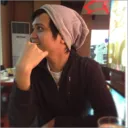
That's not really what I want to do as it pertains to resources like documents - what I want to do is after a user registers, they'll get an email to verify their account. Until they verify their account, they should be just be stuck on the login screen until they click on the link. Any attempt to login as an unverified account should be blocked. The permissions are for a different layer I think.
Anyway I tried:
const userAccount = await account.create(ID.unique(), email, password, `${firstname} ${lastname}`);
await account.createEmailSession(email, password);
await account.createVerification(Constants.BASE_URL);
await account.deleteSessions();
But I'm still getting the same error.
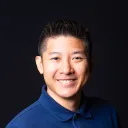
Until they verify their account, they should be just be stuck on the login screen until they click on the link.
Your UI can just show a page saying they must verify instead of showing data
The permissions are for a different layer I think.
No, i recommend this approach.
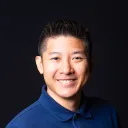
where is this code running? client side or server side?
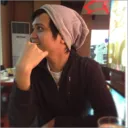
Currently on server side.
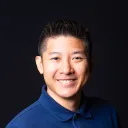
well ya that's not going to work because the web sdk uses cookies or local storage for session management. neither of those are available server side
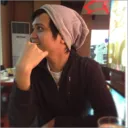
So what's the flow that I should implement here?
[CLIENT] - User submits the registration form
[SERVER] - Validate the registration form and return the result of account.create()
[CLIENT] - Call account.createEmailSession()
[CLIENT] - Call account.get()
[CLIENT/SERVER] - call account.createVerification()
This doesn't look right to me.
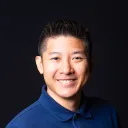
SSR is a huge pain. I would recommend avoiding it if possible. If you really want to use SSR, you'll need to figure out a way to have the session server-side when needed.
One way to do this is to create the session client side, create a jwt token, and then pass that server side.
Another way is to proxy the session creation through your backend where your backend will create the session manually, grab the cookie, and set it for the client. You can see an example of that here: https://next-js.ssr.almostapps.eu/
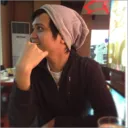
I think I am okay with avoiding SSR for most stuff - I really only need the backend API of NextJS to limit the registrations and login to certain emails - that's why I have some extra validation before I call account.create()
.
Recommended threads
- Need help setting up this error is showi...
You can't sign in to this app because it doesn't comply with Google's OAuth 2.0 policy. If you're the app developer, register the redirect URI in the Google Cl...
- Direct Upgrade from Appwrite v1.5.11 to ...
I'm on Appwrite v1.5.11. Can I upgrade directly to v1.6.2?
- Appwrite stopped working, I can't authen...
I'm having an issue with Appwrite. It was working fine just a while ago, but suddenly it stopped working for me and can't authenticate accounts. I even went bac...
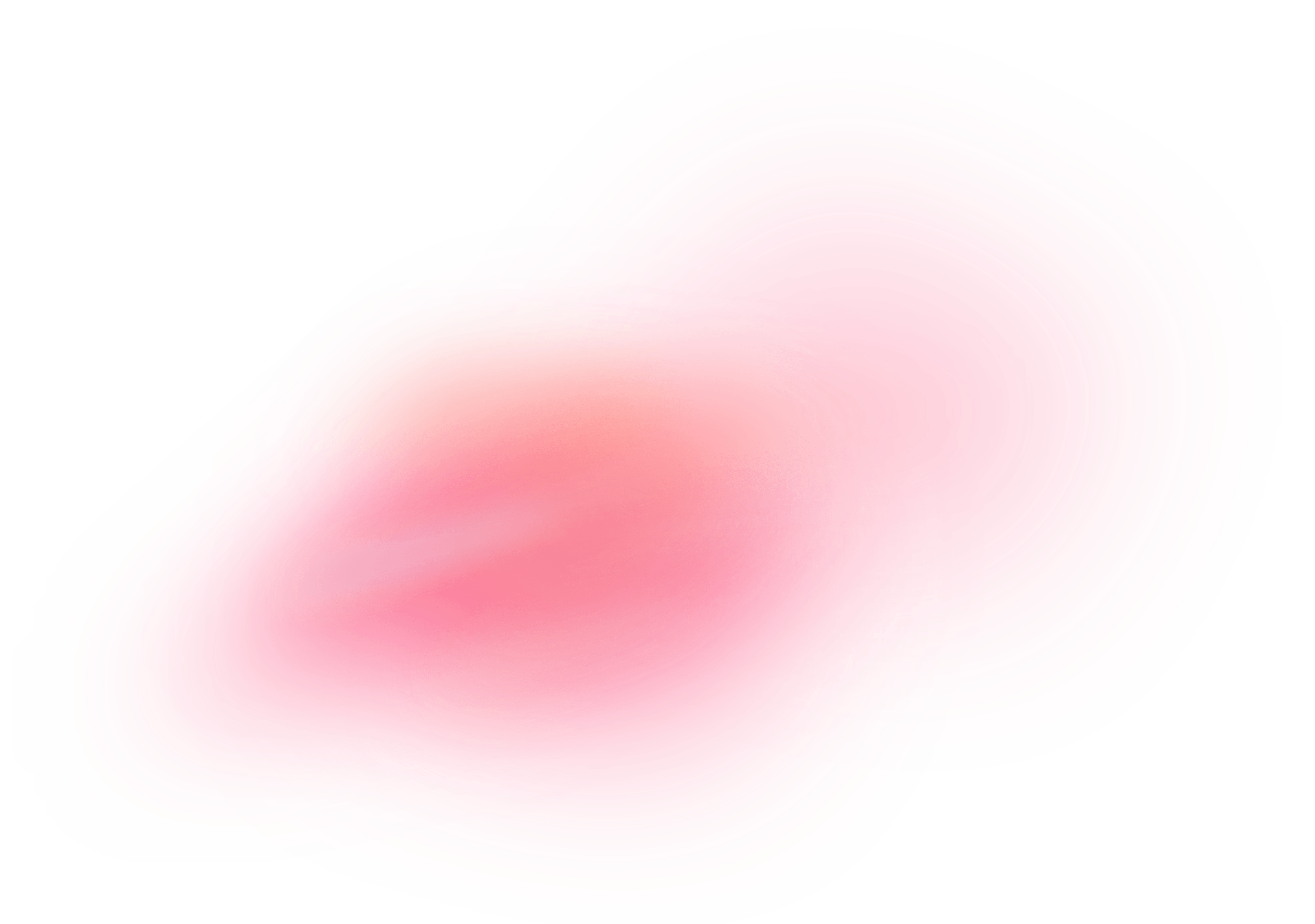