
I'm using Appwrite to create an email session with users. At the moment, I do very basic checks within the login form component that check if the password is longer than 8 characters. I then call a function in a separate utility file that handles the login. How can I check for errors such as no user found? Is there perhaps a boilerplate for an appwrite login and register function that I can look at? I'm fairly new to React, is code within a component secure, i.e: can I call the createEmailSession
from the component file?
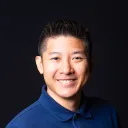
You can put it in the component. It's up to you how you want to organize your code.
To handle errors, wrap in try/catch, and then handle the exception
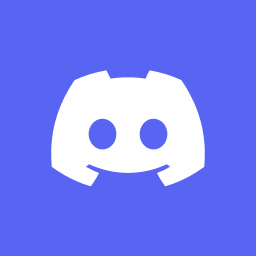
Hi @larkx , here is a pseudo example using try catch:
import { sdk } from 'appwrite';
const loginUser = async (email, password) => {
try {
await sdk.account.createEmailSession(email, password);
} catch (error) {
if (error.code === 'user-not-found' // any other error you are checking for) {
console.log('User not found. Please check your credentials.');
} else {
console.log('An error occurred:', error.message);
}
}
};```
Recommended threads
- self-hosted auth: /v1/account 404 on saf...
Project created in React/Next.js, Appwrite version 1.6.0. Authentication works in all browsers except Safari (ios), where an attempt to connect to {endpoint}/v1...
- delete document problems
i don't know what's going on but i get an attribute "tournamentid" not found in the collection when i try to delet the document... but this is just the document...
- Update User Error
```ts const { users, databases } = await createAdminClient(); const session = await getLoggedInUser(); const user = await users.get(session.$id); if (!use...
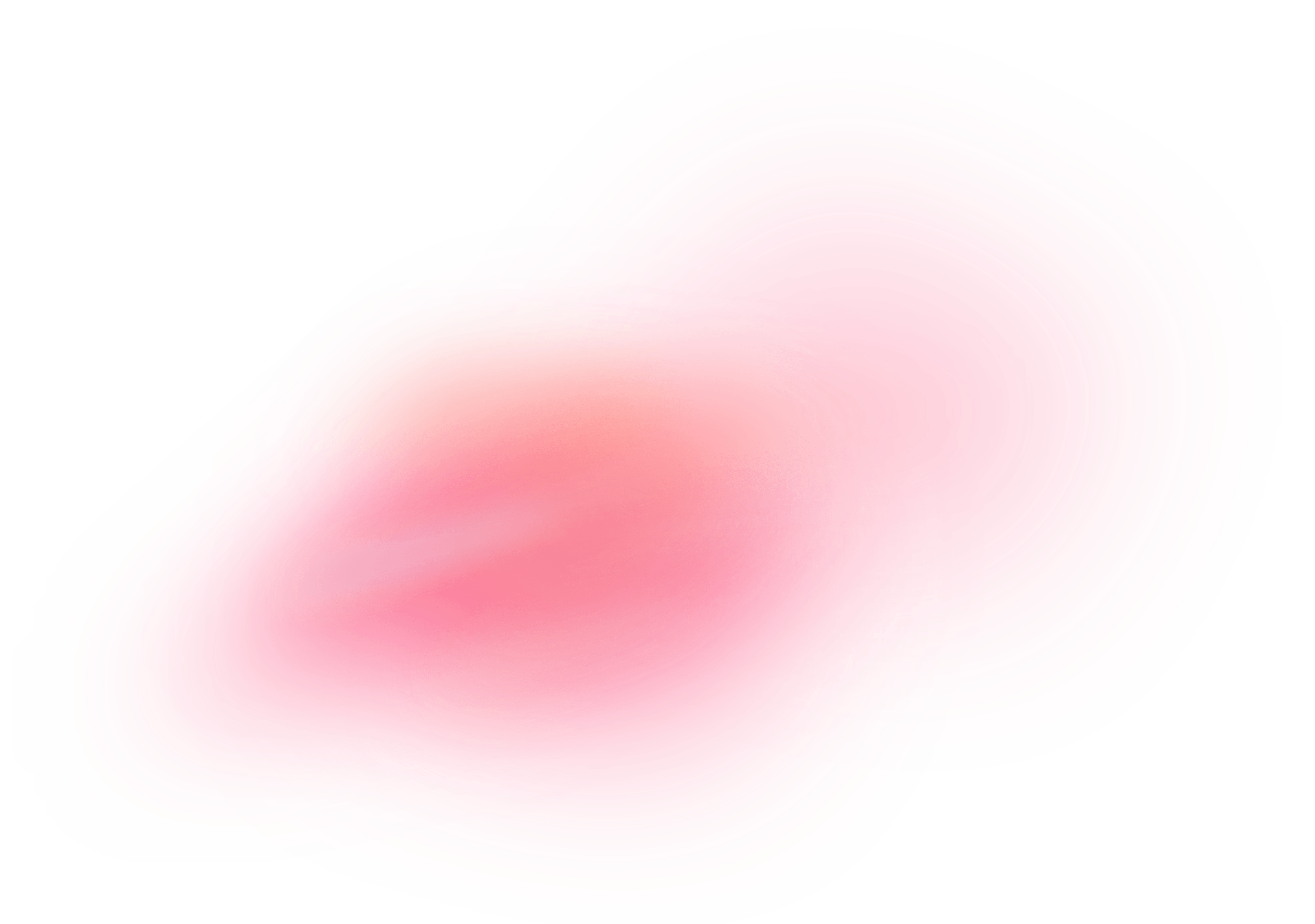