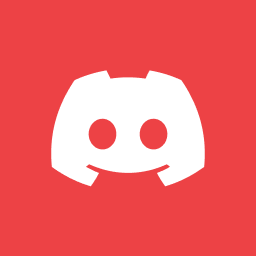
How do I fix internal runtime error causing my function to failed
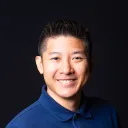
How long does the function run for and what's the timeout on the function?
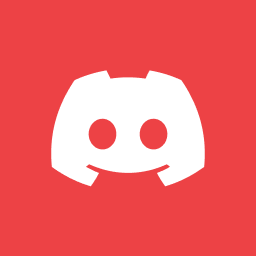
It run for 23ms and the max timeout is 900 basically the default appwrite timeout
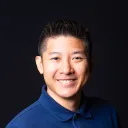
So this typically happens when an exception is thrown by your code. Try to add try/catch to prevent your code from throwing an exception
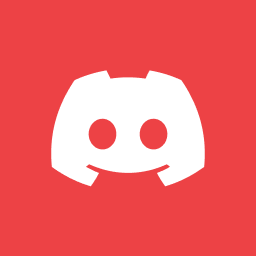
i do have that in my code from appwrite.client import Client from appwrite.services.databases import Databases
def main(req, res): try:
client = Client()
if not req.variables.get('APPWRITE_FUNCTION_ENDPOINT') or not req.variables.get('APPWRITE_FUNCTION_API_KEY'):
print('Environment variables are not set. Function cannot use Appwrite SDK.')
else:
client.set_endpoint(req.variables.get('APPWRITE_FUNCTION_ENDPOINT', None))
client.set_project(req.variables.get('APPWRITE_FUNCTION_PROJECT_ID', None))
client.set_key(req.variables.get('APPWRITE_FUNCTION_API_KEY', None))
client.set_self_signed(True)
# Create the database instance
database = Databases(client)
# Retrieve the collection and database IDs from environment variables
collection_id = req.variables.get('APPWRITE_FUNCTION_COLLECTION_ID', None)
database_id = req.variables.get('APPWRITE_FUNCTION_DATABASES_ID', None)
if collection_id and database_id:
Extraction(req.payload, database, database_id, collection_id)
else:
print('Collection or Database environment variables not set.')
return res.json({
"areDevelopersAwesome": True
})
def Extraction(document, database, database_id, collection_id): id = document['$id'] name = document['name'] email = document['email']
# Use the database and collection IDs to create a document with the same ID as the 'id' field
usersDocument = database.create_document(
database_id,
collection_id,
document_id=id, # Set the document ID to the value of the 'id' field
data={
'id': id,
'name': name,
'email': email
}
)
except Exception as e: print(e) return res.json({...})
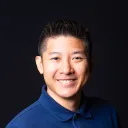
Btw, it's best to use 3 back ticks with multi-line code. See https://www.markdownguide.org/extended-syntax/#syntax-highlighting
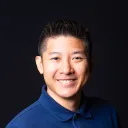
Instead of printing e, try to print str(e)
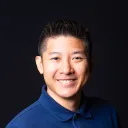
Is the indentation at the end wrong from copying and pasting? What's in the res.json() at the end?
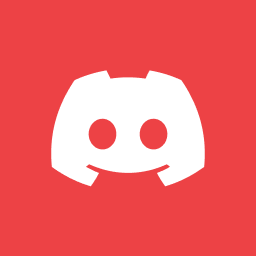
Yes it did copy from a previous support ticket with similar problem, I remove it and except Exception as e: print (βAn error occurred:β, str(e)) still getting the same error
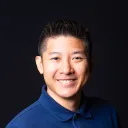
What's the full updated code now? Please make sure to format it using back ticks.
How long is it running for?
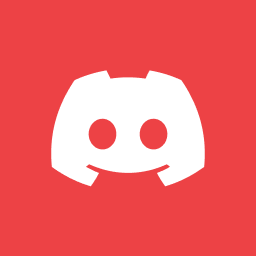
here is the updated code btw am not really sure about using back ticks and it running 3ms
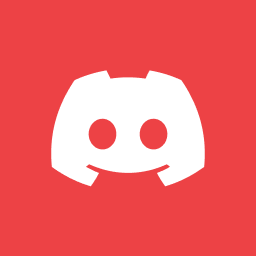
from appwrite.client import Client from appwrite.services.databases import Databases
def main(req, res): try:
client = Client()
if not req.variables.get('APPWRITE_FUNCTION_ENDPOINT') or not req.variables.get('APPWRITE_FUNCTION_API_KEY'):
print('Environment variables are not set. Function cannot use Appwrite SDK.')
else:
client.set_endpoint(req.variables.get('APPWRITE_FUNCTION_ENDPOINT', None))
client.set_project(req.variables.get('APPWRITE_FUNCTION_PROJECT_ID', None))
client.set_key(req.variables.get('APPWRITE_FUNCTION_API_KEY', None))
client.set_self_signed(True)
# Create the database instance
database = Databases(client)
# Retrieve the collection and database IDs from environment variables
collection_id = req.variables.get('APPWRITE_FUNCTION_COLLECTION_ID', None)
database_id = req.variables.get('APPWRITE_FUNCTION_DATABASES_ID', None)
if collection_id and database_id:
Extraction(req.payload, database, database_id, collection_id)
else:
print('Collection or Database environment variables not set.')
return res.json({
"areDevelopersAwesome": True
})
def Extraction(document, database, database_id, collection_id): id = document['$id'] name = document['name'] email = document['email']
# Use the database and collection IDs to create a document with the same ID as the 'id' field
usersDocument = database.create_document(
database_id,
collection_id,
document_id=id, # Set the document ID to the value of the 'id' field
data={
'id': id,
'name': name,
'email': email
}
)
except Exception as e: print('An error occurred:', str(e))
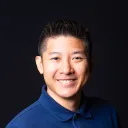
Please read what I linked and update your message: https://discord.com/channels/564160730845151244/1130068412010340433/1130170843637485658
Recommended threads
- How to detect user disconnection?
I'm creating a 1v1 challenge using realtime and i want to trigger a function when the user disconnect... how to make this using Appwrite Realtime? i searched i...
- How can I use appwrite function for stre...
I am building a course website where I want users can view the videos stored in appwrite storage in diff quality and also will do some processing before streami...
- Every time I deploy a function via CLI, ...
deploying appwrite function via cli breaks Git connection in function settings tab but when I push to git triggered deployment fail. usually multiple deployment...
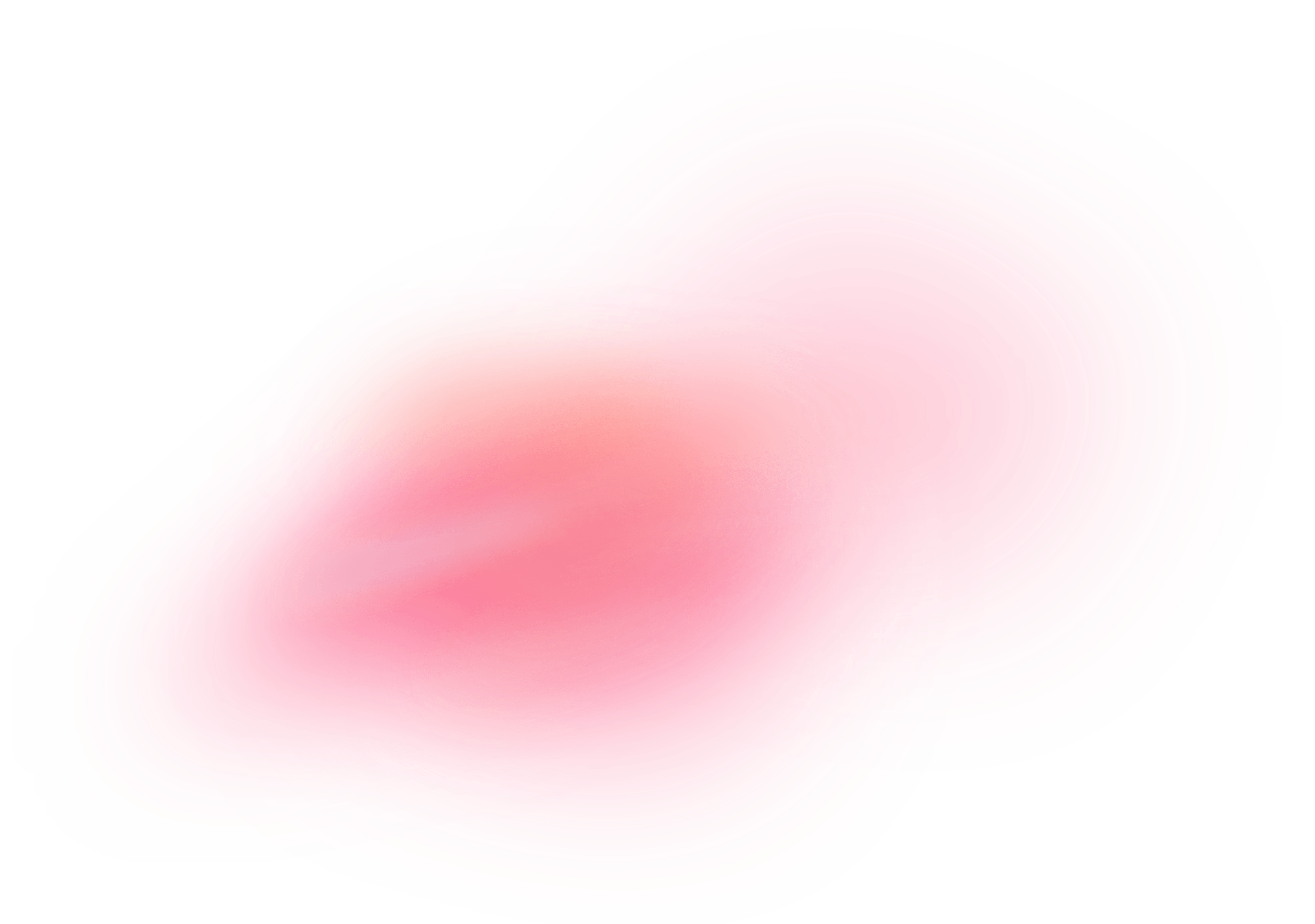