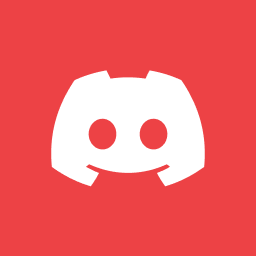
Hey team sorry for the unnecessary support req but this is the first time am write a cloud function as I start to learn python I just want to know if this function is correct if not can someone help on what I should change.
from appwrite.client import Client from appwrite.services.database import Database
//Initialize the Appwrite client client = Client() client.set_endpoint('https://YOUR_APPWRITE_ENDPOINT') client.set_project('YOUR_APPWRITE_PROJECT') client.set_key('YOUR_APPWRITE_API_KEY')
//Initialize the database service database = Database(client)
//Define the function to auto-populate profile collection def populate_profile(document): //Extract the necessary fields from the user's payload userId = document['$id'] email = document['email'] name = document['name']
# Create a new document in the profile collection
profileDocument = database.create_document(
collectionId='YOUR_PROFILE_COLLECTION_ID',
data={
'userId': userId,
'email': email,
'name': name,
# Add additional fields as needed
}
)
//Log the newly created profile document ID
print('Profile document created:', profileDocument['$id'])
//Call the function when a user is created populate_profile(event['payload'])
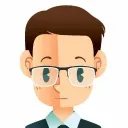
To easily create a function for Appwrite
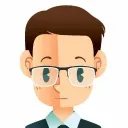
You can either use the Appwrite cli - which is the best way.
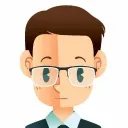
Or you can copy and start with one of the functions starters available here https://github.com/appwrite/functions-starter/tree/main/python-3.10
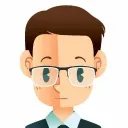
In your code, its looks like client
side code
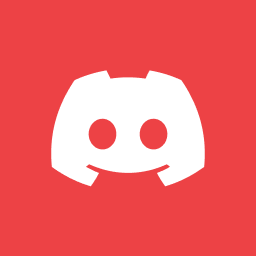
I sure will btw since am new to python what would you recommend to change in my function
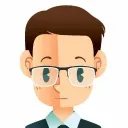
Are you referring to Appwrite cloud functions, Or, in general?
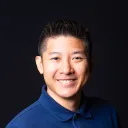
Btw, it's best to use 3 back ticks with multi-line code. See https://www.markdownguide.org/extended-syntax/#syntax-highlighting
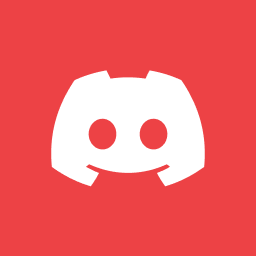
Appwrite cloud function
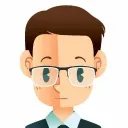
So for that you'll need to put your code in the starter function, like the link above. It will look something like this
from appwrite.client import Client
from appwrite.services.databases import Databases
def main(req, res):
client = Client()
client.set_endpoint('https://your_appwrite_endpoint/')
client.set_project('YOUR_APPWRITE_PROJECT')
client.set_key('YOUR_APPWRITE_API_KEY')
database = Databases(client)
populate_profile(req.payload)
return res.json({
"areDevelopersAwesome": True,
})
def populate_profile(document):
userId = document['$id']
email = document['email']
name = document['name']
# Create a new document in the profile collection
profileDocument = database.create_document(
collectionId='YOUR_PROFILE_COLLECTION_ID',
data={
'userId': userId,
'email': email,
'name': name,
# Add additional fields as needed
}
)
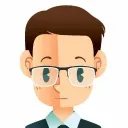
This is the basic code
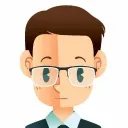
It won't work out of the box
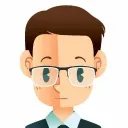
You'll need to set, the
- Appwrite project
- Appwrite key
- CollectionID
- DatabaseID
The code you have used seems to be outdated.
As it use an older version of the create_document
function without database.
What I recommend is to try this:
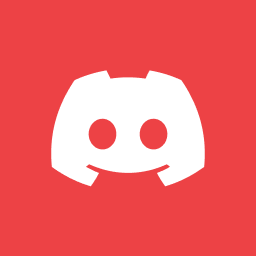
I sure will check them out, thank you for your help!
Recommended threads
- Where is tensorflow support? 3.11 ML doe...
and if i manually tried to add tensorflow i get Cannot access offset of type string on string no matter what
- OAuth2 Error: invalid success param url ...
Hi everyone! I'm trying to implement Google OAuth2 login in a React Native app (using the Android simulator) with Appwrite Cloud, and I'm getting the following ...
- Trying to set up a new appwrite project
we have used the folder structure as follow Functions /function-name /src/index,js This is our entrypoint and git confugre settings When I try to run ...
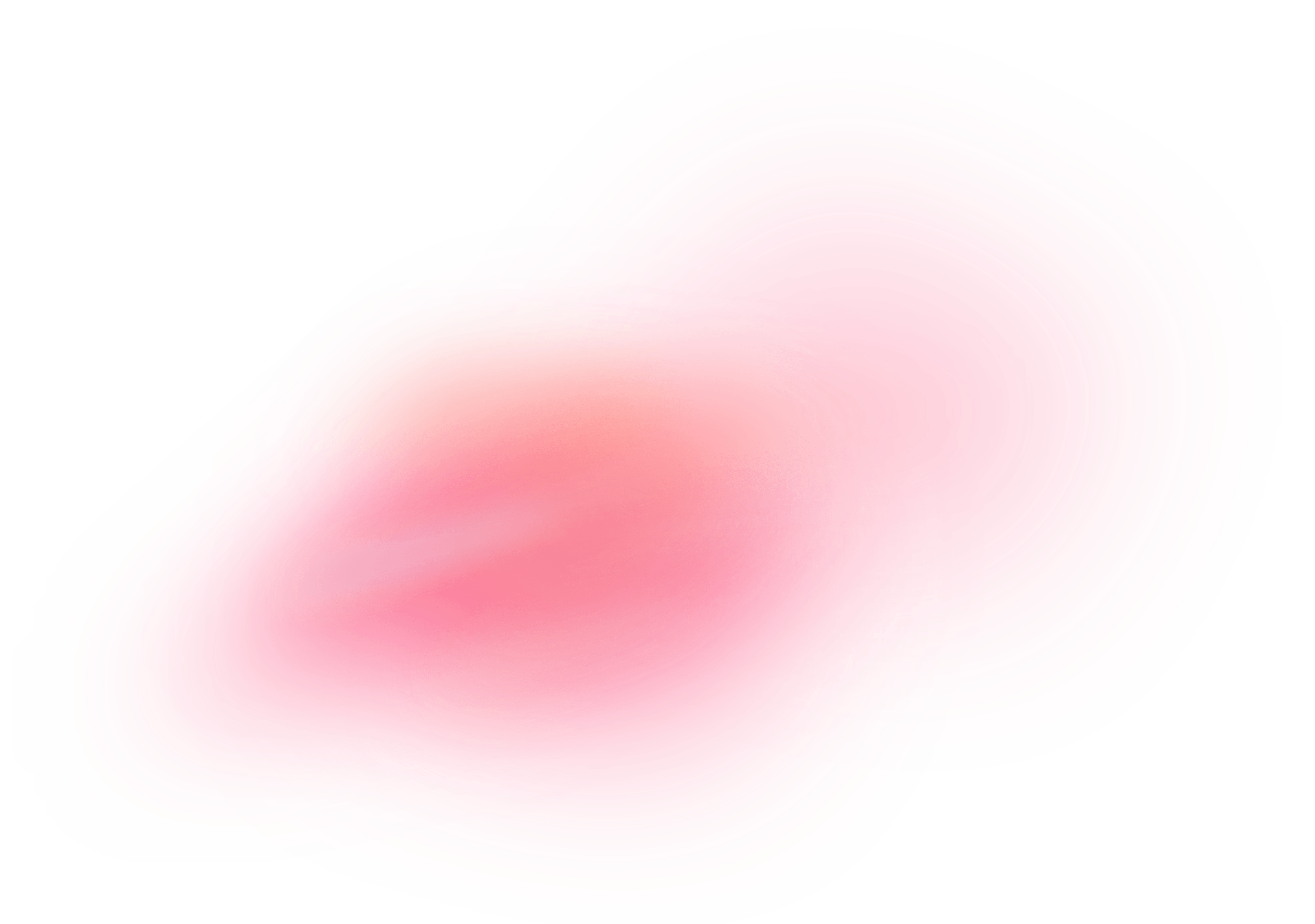