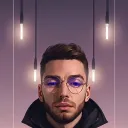
I have a list of documents that I want to query only for the respective "clients" collection that is in relationship with the "projects" collection.
Is that possible? Or should I query all the projects and then filter by clients?
const queryBuilder = () => {
const queryArray = []
if (search) {
queryArray.push(Query.search('name', search))
}
queryArray.push(Query.limit(limit))
queryArray.push(Query.offset(offset))
return queryArray
}
const getDocuments = async () => {
if (!database) return
await database.listDocuments(
Server.databaseId!,
Server.collections.projects!,
queryBuilder())
.then((res: any) => {
setprojects(res.documents)
setDocumentsCount(res.total)
})
.catch((err: Error) => {
showSnackbar(err.message, 'error')
})
}

Queries are not available for relationship at the moment
https://appwrite.io/docs/databases-relationships#query
So you will have to query all projects and filter by clients as you mention it
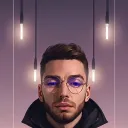
@Guille huh... but this works as expected thought... :
const queryArray = []
if (search) {
queryArray.push(Query.search('name', search))
}
if (clientId) {
queryArray.push(Query.equal('clients', clientId))
}
queryArray.push(Query.limit(limit))
queryArray.push(Query.offset(offset))
return queryArray
}

But seems like you are performing the query in the parent attributes not relational or I am wrong? 🤔

@Axentioi
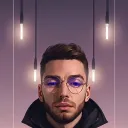
I am querying the documents from the projects collection, and I want to only take the projects only for the related client so I added the Query.equal('clients'', clientId) which is the related client for the project so I get back the correct response
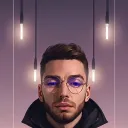
[Solved] How to query relationships in database.listDocuments()
Recommended threads
- A feature/Fix request
Whenever I use Appwrite then to see the items of document I've to click "columns" option and select those items that I want to see then if I refresh browser/pa...
- 401 - Project is not accessible in this ...
This is on the app write console https://screen.aryanwadhera.tech/7YTVhLTf
- functions page returns 500
I am running selfhosted appwrite version 1.6.0 and all of a sudden my functions page stopped working, returning a 500. I don't see anything in the logs that wo...
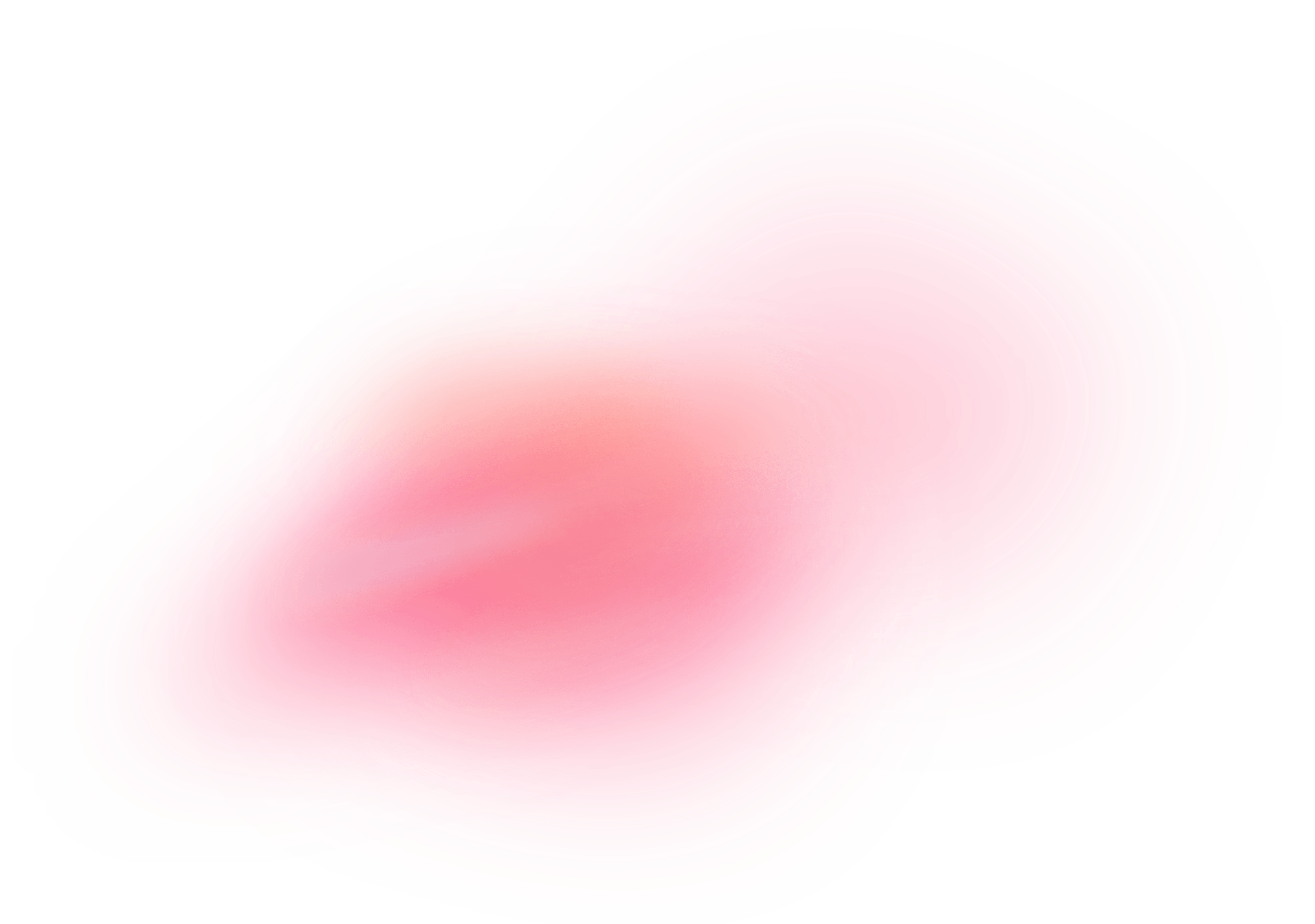