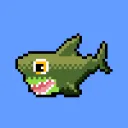
Hello, I want to update password for the logged in user in my android app(Kotlin). I'm using 2.0.x version of appwrite sdk and maybe I can't find the proper documentation for this version. When I downgrade the dependency version to 1.2.x or 1.3.x, I got an exception of "Invalid credentials. Please check email and password".
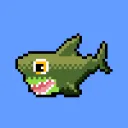
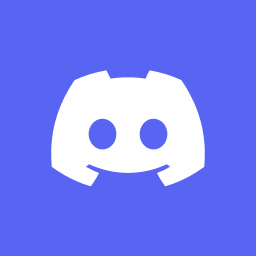
Could you send the code you're using to update the password?
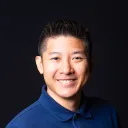
Version 2.0.0 of the Android SDK is for Appwrite version 1.3.x (notice the 1st line of the SDK Readme).
Docs are here: https://appwrite.io/docs/client/account?sdk=android-kotlin&v=1.3.x#accountUpdatePassword
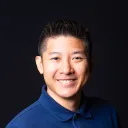
You said
the update doesn't seem to be happening
Can you provide more detail? Perhaps you can print either the response
or e
to verify which part is being executed.
I would actually assume you're getting an error because you're not passing the old password.
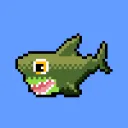
I have the updated code like the following:
class UpdatePasswordActivity : AppCompatActivity() {
private lateinit var binding: ActivityUpdatePasswordBinding
@OptIn(DelicateCoroutinesApi::class)
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
binding = ActivityUpdatePasswordBinding.inflate(layoutInflater)
setContentView(binding.root)
AppwriteManager.initialize(this)
binding.updatePasswordBtn.setOnClickListener {
GlobalScope.launch {
try {
val response = account.updatePassword(binding.password.text.toString(),null)
Toast.makeText(
this@UpdatePasswordActivity,
"Password updated successfully",
Toast.LENGTH_SHORT
).show()
Log.d("UpdatePassword",response.toString())
//startActivity(Intent(this@UpdatePasswordActivity, LoginActivity::class.java))
//finish()
} catch (e: AppwriteException) {
Log.d("UpdatePasswordException",e.message.toString())
}
}
}
}
}
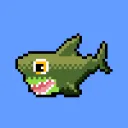
Then got the exception like this
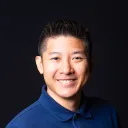
Btw, it's best to use 3 back ticks with multi-line code. See https://www.markdownguide.org/extended-syntax/#syntax-highlighting
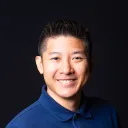
Right... You didn't pass the correct old password...

I'm recommend you to catch this error and make Toast to your users if new/old password is not correct.
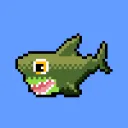
Thanks. Will do it from now onwards 👍
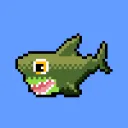
How can I fetch the old password of the user? Like the following?:
account.get().password
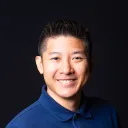
no...the user needs to input their old password...
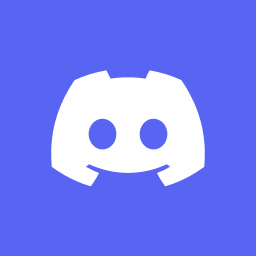
Also it should not be possible nor recommended, since that means not using the default hashed system (it's insecure being able to get the password)
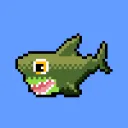
Understood. But if a user forgets his old password, what should he do?
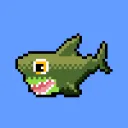
I have also passed old password in parameter as below but still getting the same exception as UpdatePasswordException com.example.appwrite_userauth D Invalid credentials. Please check the email and password.
:
binding.updatePasswordBtn.setOnClickListener {
GlobalScope.launch {
try {
val response = account.updatePassword(binding.password.text.toString(),"12345678")
Toast.makeText(
this@UpdatePasswordActivity,
"Password updated successfully",
Toast.LENGTH_SHORT
).show()
Log.d("UpdatePassword",response.toString())
//startActivity(Intent(this@UpdatePasswordActivity, LoginActivity::class.java))
//finish()
} catch (e: AppwriteException) {
Log.d("UpdatePasswordException",e.message.toString())
}
}
}
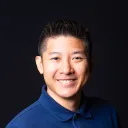
This API is not for forgetting password...for that we have this: https://appwrite.io/docs/client/account?sdk=android-kotlin#accountCreateRecovery
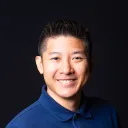
is that the correct old password?
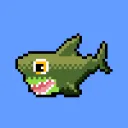
Yes it was correct.
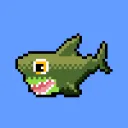
Okay, trying this way.
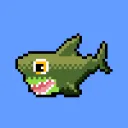
Can you tell me what will be the value for url
? I mean how can I redirect user to my app through url?
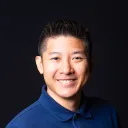
according to the error, the old password was incorrect 🤷🏼♂️
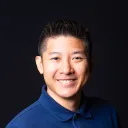
maybe this will help: https://stackoverflow.com/questions/47887464/launching-an-app-from-a-url
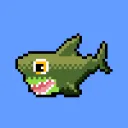
I am sorry for the inconvenience. I updated the password as the right password had been passed.
Recommended threads
- OAUTH2 doesn't works
https://github.com/202420505/APPWRITE-GOOGLE-AUTH Is my code wrong? I think this is also related to deeplinks
- Auth administration members are not show...
Auth administration members are not showing in authentication so plz help
- White Screen of Death
I am building an android application which is using React native(EXPO) and Appwrite. But While using the App in the Expo Go or Orbit it works perfectly wihout e...
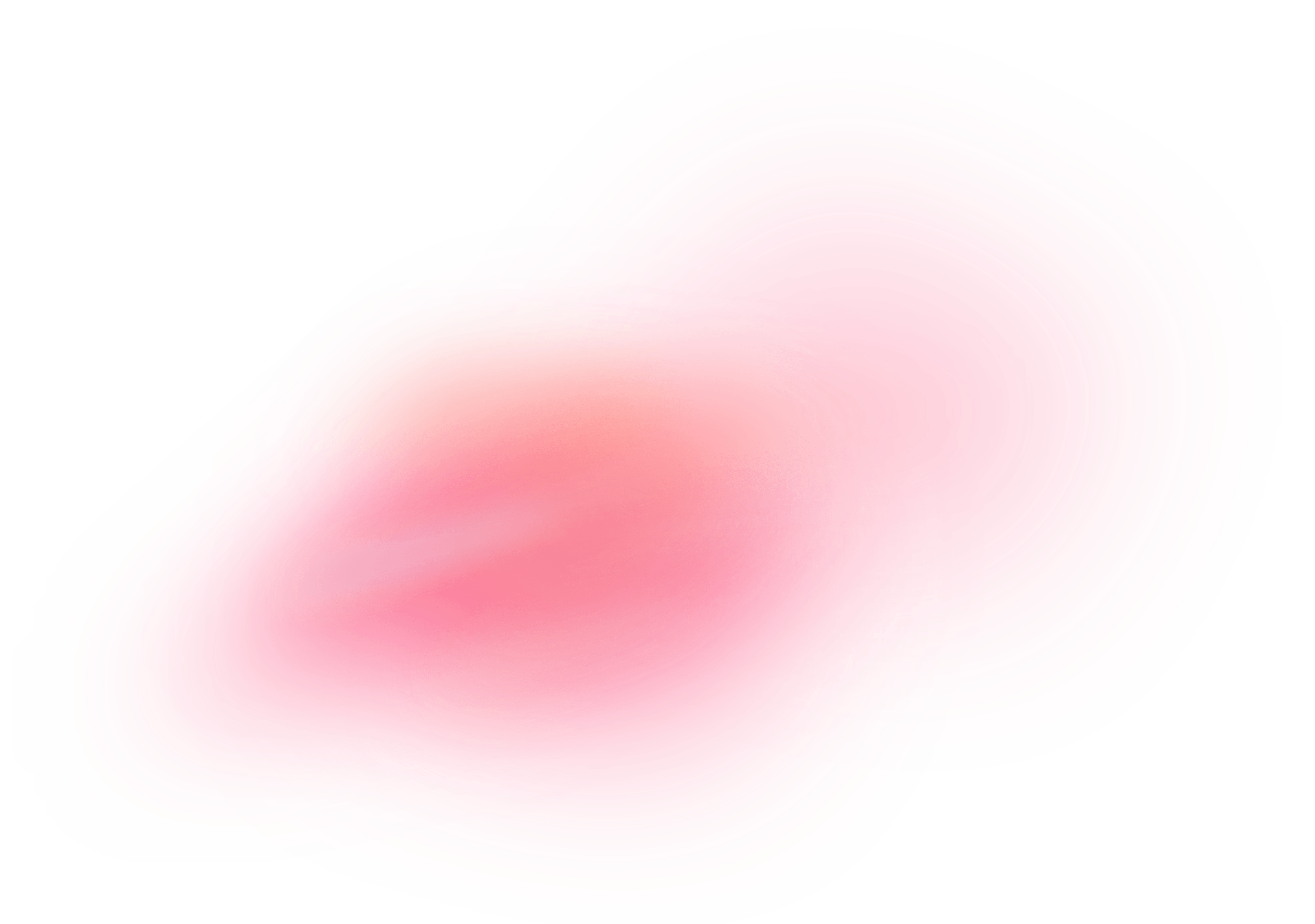