
I'm using the nodejs server sdk and awaiting for the attribute to be created and then I'm trying to add a document to the collection. But the await gets completed even with processing status and then when my server tries to add a document, unknown attribute error pops up.

newRoomDatabaseId,
"participants",
"isMicOn",
true
);
console.log(x.status);
await db.createDocument(
newRoomDatabaseId,
"participants",
roomData.admin_username,
{
isAdmin: true,
isModerator: true,
isSpeaker: true,
isMicOn: false,
}
);```

x.status shows processing even after the async call gets completed

And i have added three more create attribute calls to create other attributes in the data given to create the doc
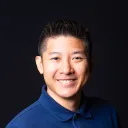
Yes, this is expected. The API call returns when the job is queued. You'll have to poll for when the status is available

I checked the status after 40 seconds and its still under processing.

Is there any other way to check if the attribute is available ?
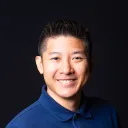
So right now it's still processing?

Yeahh

I just added a delay of 40 seconds and then checked the x.status . It showβs processing, but now the document gets added correctly.
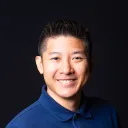
What do you mean you added a delay? And how are you checking the status?

I added a time delay as a promise for 40 seconds and then when it resolves, Im just console logging x.status
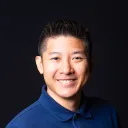
Can you share your code?

newRoomDatabaseId,
"participants",
"isMicOn",
true
);
console.log(x.status); //Prints processing
await new Promise(resolve => setTimeout(resolve, 5000));
console.log(x.status); //Prints processing
await db.createDocument(
newRoomDatabaseId,
"participants",
roomData.admin_username,
{
isMicOn: false,
}
);
console.log(x.status); //Prints processing```
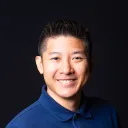
That doesn't work...you're just waiting and then looking at the same status string from when it was first created

Can you please help me with what has to be done here to achieve my requirement?
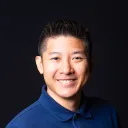
You need to fetch the attribute/collection again after waiting to see the new status

Okayy. Thanks @Steven . Will check that in sometime.

Hey @Steven This is the algorithm I came up with.
// Polling until all the required attributes are added to the collection
while (true) {
let participantCollection = await db.getCollection(
newRoomDatabaseId,
"participants"
);
let attributesAvailable = true;
participantCollection.attributes.forEach((attribute) => {
if (attribute.status != "available") {
attributesAvailable = false;
}
});
if (attributesAvailable === true) {
break;
}
}

It now works fine but I'm not sure if this is efficient. Need help in this regard.
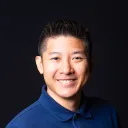
You should probably add some sort of delay between each iteration and set some sort of maximum so you don't get stuck in an infinite loop

Okayy. Will do that. Thanks @Steven
Recommended threads
- Realtime with multiple connections
I need the Realtime on multiple Collections for diffrent applicational logic. So my question is: Is there a way to have only 1 Websocket connection or do I need...
- Can't login or deploy functions in Appwr...
Hello, since i updatet to the appwrite cli 6.1.0 i can't login or deploy functions with the cli. When i call the command: "appwrite get account --verbose" i ge...
- Create admin user?
I'm not really sure how this is supposed to work, I installed Appwrite through docker-compose and set it up. When I launched the app and went into it, I created...
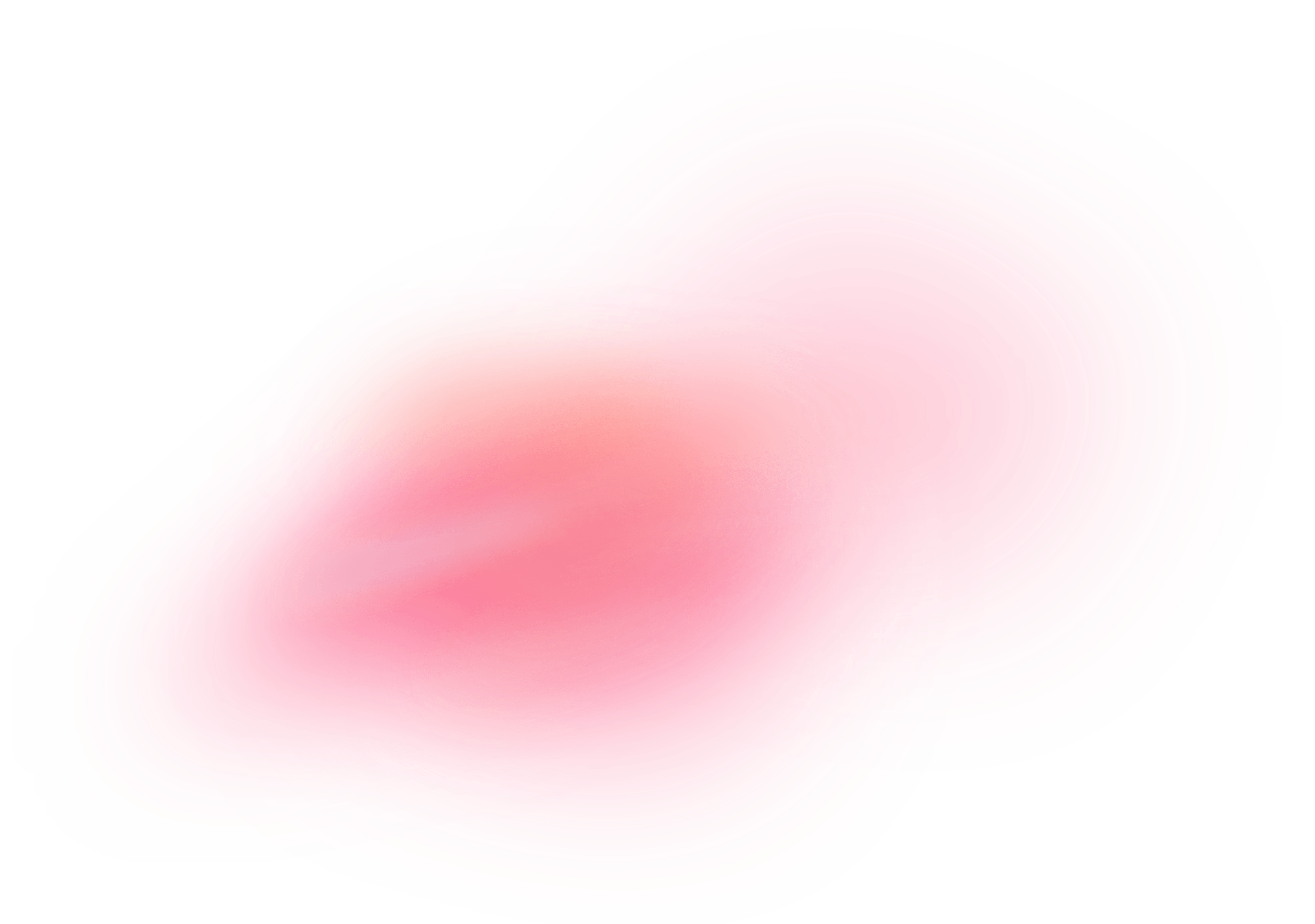