
When I run the below function with using Promise.all()
instead of await
ing each promise individually, one of the calls to createDocument
will fail almost every time.
Whereas, when I run the code as is, it seems to work every time.
Does anyone have any idea why?
Code:
export async function register(username: string, avatarFile: any) {
try {
const accountData = await account.get();
const {$id: auth_id} = accountData;
let avatar_id = null;
if (avatarFile) {
const file = await storage.createFile('profile_pictures', ID.unique(), avatarFile);
avatar_id = file.$id;
}
// const promiseUser = await db.createDocument(
await db.createDocument(
'chat',
'users',
ID.unique(),
{
auth_id
}
);
// const promiseProfile = await db.createDocument(
await db.createDocument(
'chat',
'profiles',
ID.unique(),
{
auth_id,
username,
avatar_id
}
);
// Not sure why, but this was causing either the users or the profiles doc creation fail, forcing user to register twice
// await Promise.all([
// promiseUser,
// promiseProfile
// ]);
}
catch (e) {
console.warn(e);
}
}
The only thing I can think of is perhaps ID.unique()
needs a few milliseconds to get a new unique ID? But even then I'm not sure that would cause one of the writes to fail.
I've ran about 6 tests against the working method though, and it seems to work consistently.
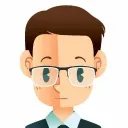
The ID.unique()
just returns a string, the logic is done in the server side.
What is the error you're getting?
Side note: Even if you don't use
Promise.all
the functions will run, thePromise.all
is just to make it easy for you to force sync execution of async logic.

Yeah that's why I'm not really understanding how this is a fix...
There is not error on creation, but the subsequent step (which expects both an entry in the users
and profiles
collection) will fail with a 500 error
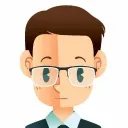
What do you see in the network tab? What are the results from Appwrite

I'll check next time I'm testing that process, but for now this is a solution, albeit a solution that I don't understand š
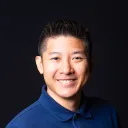
btw, if you want a promise, you can't put await. so it would need to be:
const promiseUser = db.createDocument();

Sorry those commented lines in my code were a typo, this is how I had them originally
Recommended threads
- Appwrite Fra Cloud Custom Domains Issue
Iām trying to configure my custom domain appwrite.qnarweb.com (CNAME pointing to fra.cloud.appwrite.io with Cloudflare proxy disabled) but encountering a TLS ce...
- Appwrite service :: getCurrentUser :: Us...
Getting this error while creating a react app can someone please help me solve the error
- Storage & Database is not allowing.
Storage & Database is not allowing to CRUD after i have logged in ? Using web SDK with next.js without any SSR or node-sdk.
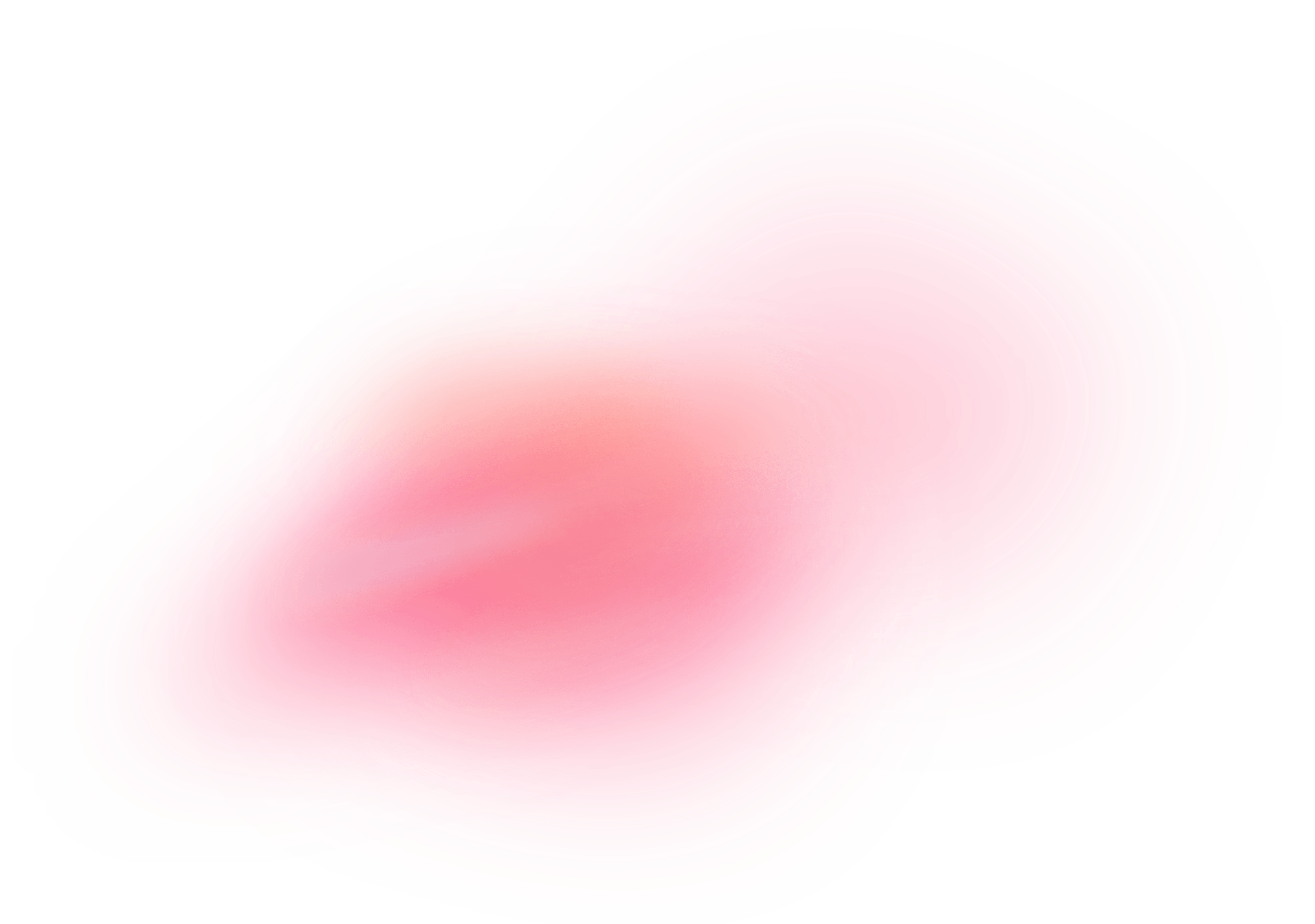