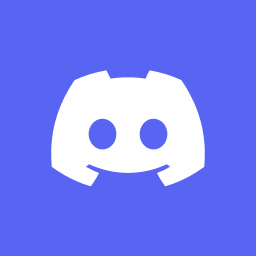
@srish What's the programming language you're using?

seems to be python
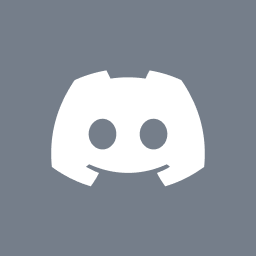
Yes python
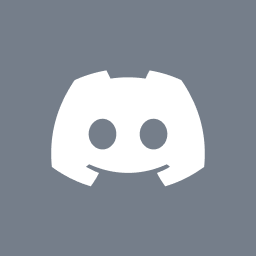
See is there any error?

You should do this:
result = users.create(ID.unique(), email=email, password=password, name=name)```

import ID
from appwrite SDK

Remove this code:
# Generate a unique user_id using the email address and a unique identifier
userid = re.sub(r'[^a-zA-Z0-9.-]', '', email) # Remove any special characters from the email
userid += '' + str(uuid.uuid4())
user_id = user_id[:36]
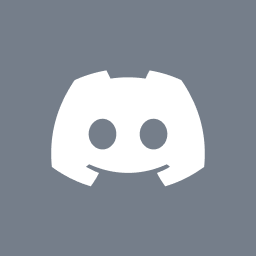
Here is the updated code
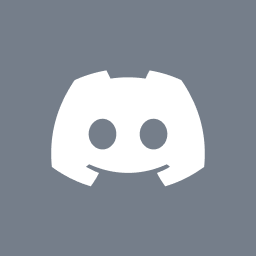
But still showing the same error
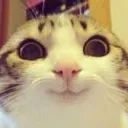
In the first image, remove your validation of user id. You are not supposed to validate user id yourself, appwrite does it on the backend and the returned error may be handled on the frontend.
FYI, ID.unique() returns the string unique()
and the backend generates an unique id internally
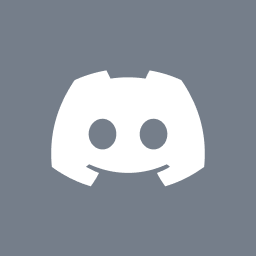
R u talking about user_id=ID.unique()?
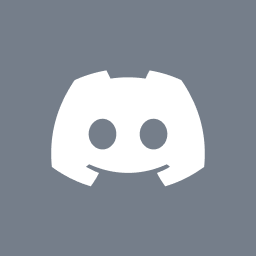
This line👆
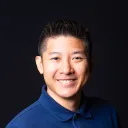
Btw, it's best to use 3 back ticks with multi-line code. See https://www.markdownguide.org/extended-syntax/#syntax-highlighting

Your code should be someting like this:
@app.route('/signup', methods=['GET', 'POST'])
def signup():
error_message = None
if request.method == 'POST':
# Retrieve form data
email = request.form['email']
password = request.form['password']
name = request.form['name'] if 'name' in request.form else ''
try:
result = users.create(ID.unique(), email=email, password=password, name=name)
response = account.create_session(email, password)
session_data = response.json()
# Store the session data in the user's session
session['userId'] = session_data['userId']
session['email'] = email
session['token'] = session_data['$id']
# Redirect to the login page after successful signup
return redirect(url_for('login'))
except Exception as e:
error_message = str(e)
# Render the signup template
return render_template('signup.html', error_message=error_message)
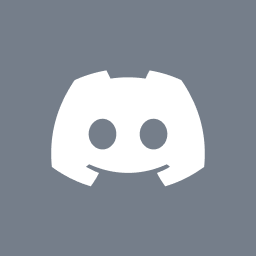
Okie let me try this one
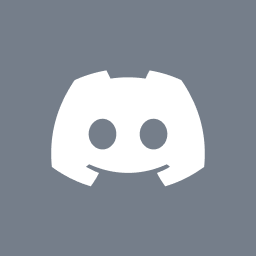
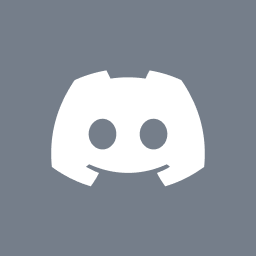
Now how to solve this?
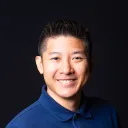
You never called client.setEndpoint()
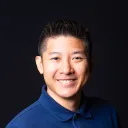
what is account.create_session(email, password)
?
did chatGPT generate this?? 😅
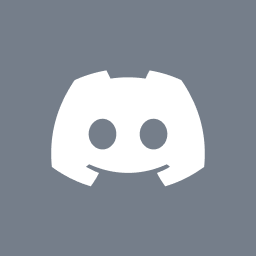
How to call client.setEndpoint()
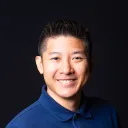
this might be helpful: https://appwrite.io/docs/getting-started-for-server
We have lots of docs so make sure to go through them thoroughly
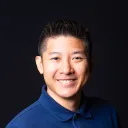
Getting started with the python SDK
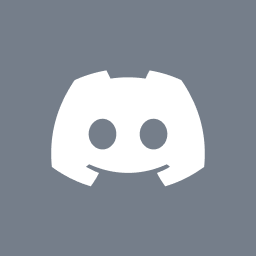
I have set endpoint
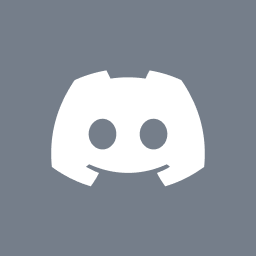
But still it is showing error
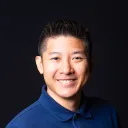
please share the exact error and your updated code
Recommended threads
- Sharing cookies
Hi, I’m using Appwrite Cloud, and I have a setup where my Appwrite backend is hosted on a subdomain (e.g., api.example.com), while my frontend (Next.js app) and...
- Organization not exists anymore
Hello! We have a problem with a cloud database. We are on the Free plan, but after a refresh the site wants me to create a new organisation, and I not see the c...
- JSON and Object Support in Collection do...
I am working with Next.Js and Appwrite Cloud, I am relatively New to Appwrite but i have noticed there is no direct support of JSON and Object support in attrib...
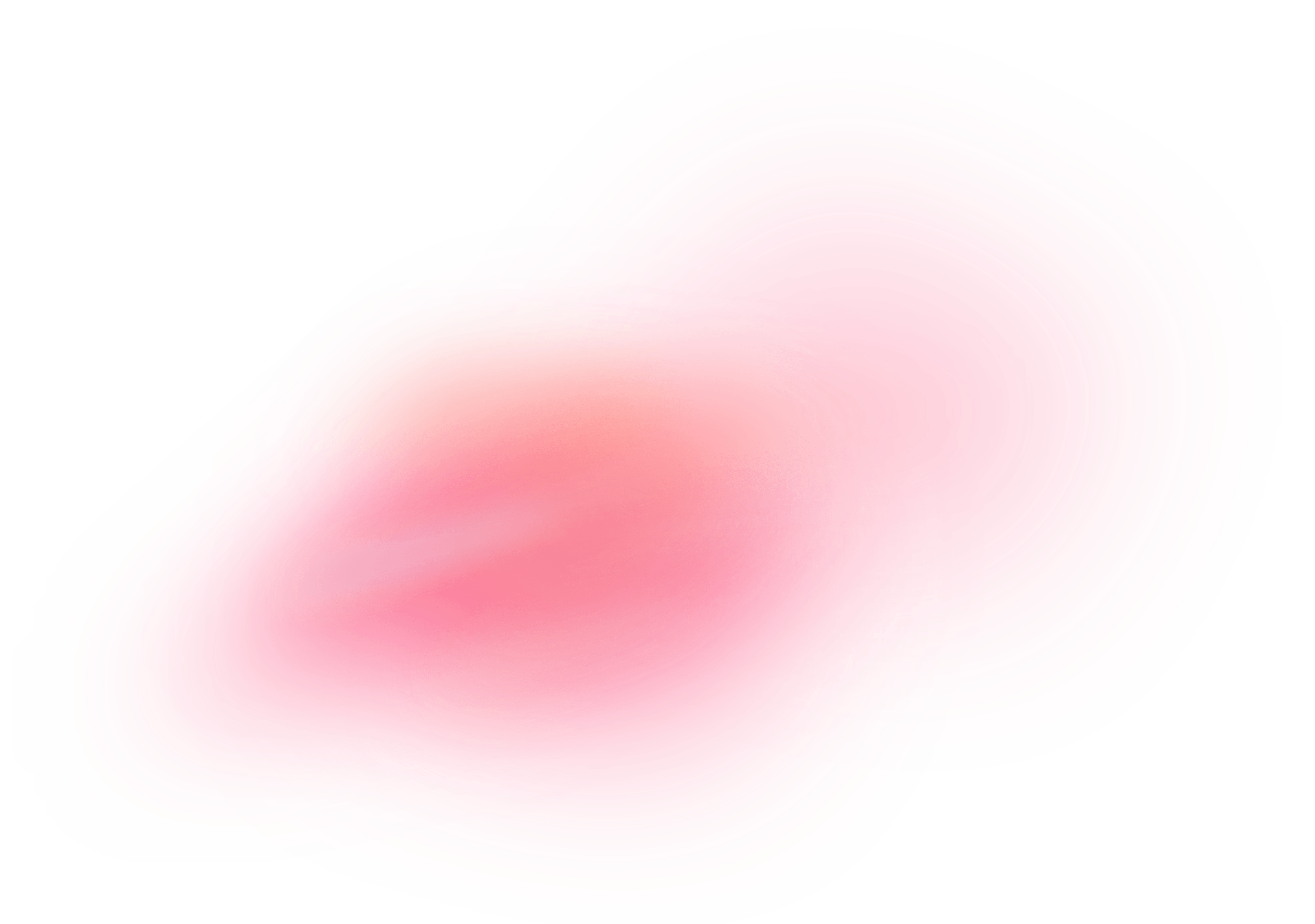