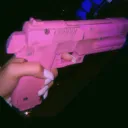
Hey I want to retrieve all documents using my python function, for which I am using pagination
I am getting this error: {"error":"Expecting value: line 1 column 1 (char 0)"}
(Also could you refer me docs/ examples for pagination in python, I couldn't really find anything)
This is my function
def main(req, res):
try:
client = Client()
if not req.variables.get('APPWRITE_FUNCTION_ENDPOINT') or not req.variables.get('APPWRITE_FUNCTION_API_KEY'):
print('Environment variables are not set. Function cannot use Appwrite SDK.')
else:
(
client
.set_endpoint(req.variables.get('APPWRITE_FUNCTION_ENDPOINT', None))
.set_project(req.variables.get('APPWRITE_FUNCTION_PROJECT_ID', None))
.set_key(req.variables.get('APPWRITE_FUNCTION_API_KEY', None))
.set_self_signed(True)
)
databases = Databases(client)
def fetch_documents(cursor):
all_docs = []
query_options = [
Query.orderDesc("age"),
Query.limit(25),
]
while True:
response = databases.list_documents(
'647ac763cf53ed9xxxxx',
'647ac820ba38520xxxxx',
query_options
)
try:
if cursor:
last_id = response['documents'][-1]['$id']
query_options.append(Query.cursorAfter(last_id))
cursor = False
documents = response['documents']
all_docs.extend(documents)
if len(documents) < 25:
break
last_id = documents[-1]['$id']
query_options[-1] = Query.cursorAfter(last_id)
except Exception as e:
all_docs = ["error"]
print(e)
break
return all_docs
return res.json({
"result": fetch_documents(True),
})
except Exception as e:
return res.json({
"error": str(e)
})```
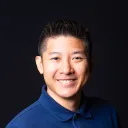
The docs on pagination are here: https://appwrite.io/docs/pagination. The idea is the same, just change the syntax for python
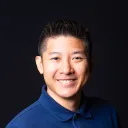
How about you test that fetch documents function locally?
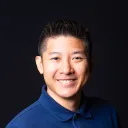
Also, did you create the endpoint variable? If so, what did you set the value to?
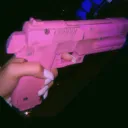
I set it to https://cloud.appwrite.io/v1
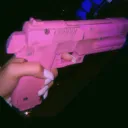
Yeah, actually that's what I tried😅
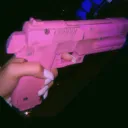
Okay, so I was debugging my code line by line, and I get this error
{"error":"type object 'Query' has no attribute 'orderDesc'"}
this is how I imported query from appwrite.query import Query
What am I doing wrong?
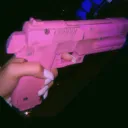
Query.orderDesc("age"),
Query.limit(25),
]
response = databases.list_documents(
'647ac763cf53edxxxxx',
'647ac820ba38520xxxx',
query_options
)```
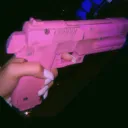
um if I remove that query,
I get type object 'Query' has no attribute 'cursorAfter'
Are the name of functions different in python? Where can I find python version of these functions😢
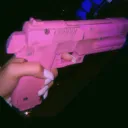
Okay, I figured it out
Recommended threads
- Attributes Confusion
```import 'package:appwrite/models.dart'; class OrdersModel { String id, email, name, phone, status, user_id, address; int discount, total, created_at; L...
- How to Avoid Double Requests in function...
I'm currently using Appwrite's `functions.createExecution` in my project. I want to avoid double requests when multiple actions (like searching or pagination) a...
- Project in AppWrite Cloud doesn't allow ...
I have a collection where the data can't be opened. When I check the functions, there are three instances of a function still running that can't be deleted. The...
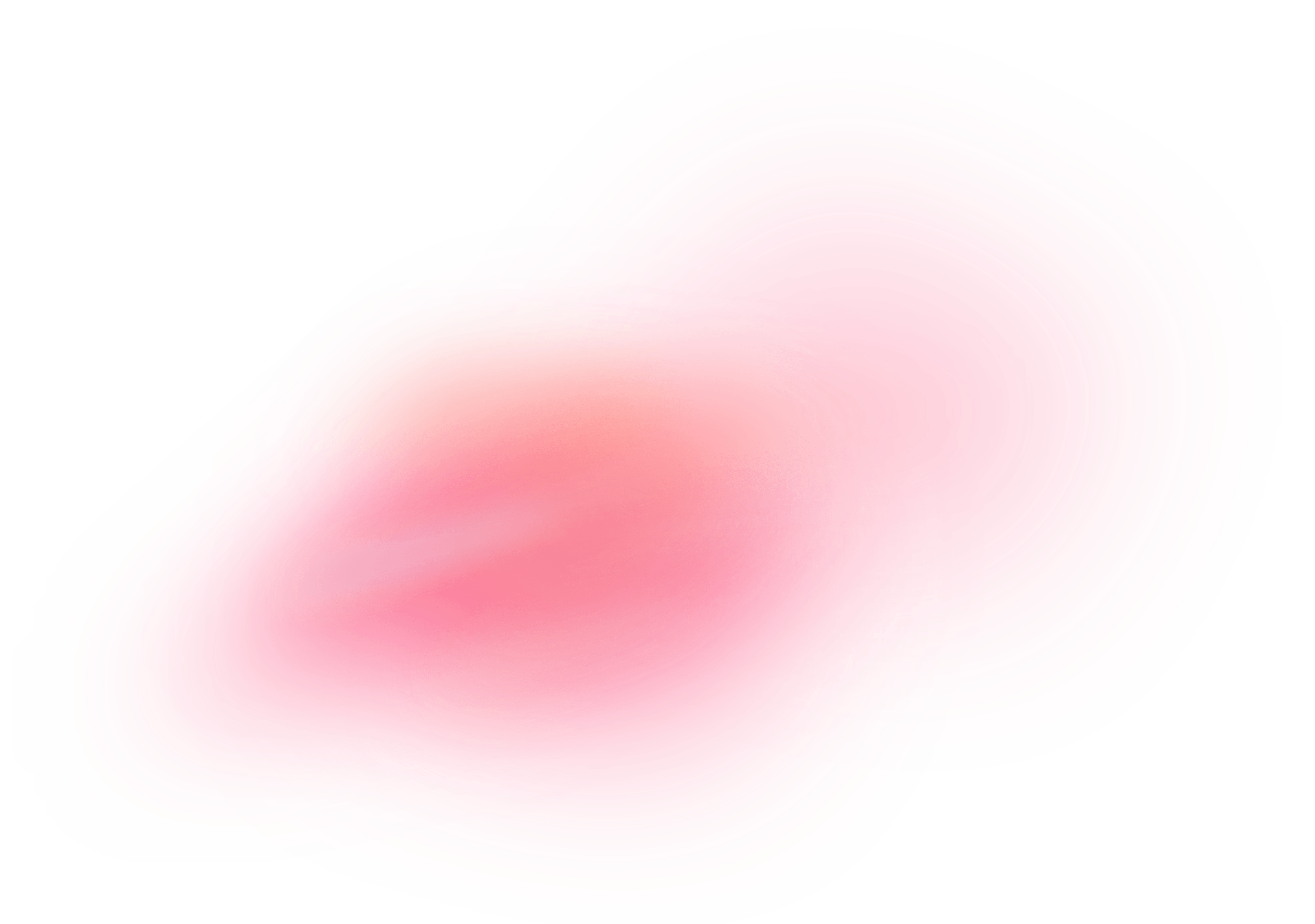