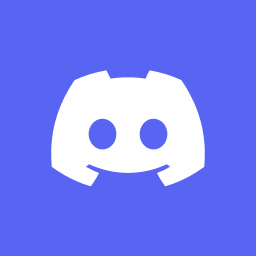
I am trying to read and write data in appwrite for my expense manager app ,since this is my first time using a database i am unable to get it right. i have written these two functions :-
try {
final document = await databases.createDocument(
databaseId: constants.database_ID,
collectionId: constants.collection_ID,
documentId: ID.unique(),
data: {'Reason': reason, 'Date': DateTime.now(),'Amount': amount,},
permissions: [
Permission.read(Role.any()),
Permission.write(Role.any()),
],
);
} on AppwriteException catch (e) {
print(e);
}
}```
and
``` Future<List<Document>> getData() async {
final Future<DocumentList> result = databases.listDocuments(
databaseId: constants.database_ID,
collectionId: constants.collection_ID,
);
result.then((result) {
return result.data.documents;
}).catchError((error) {
print(error.response);
});
return [];
}```
and from another class i am calling getdata as
//Function to map data from document of Appwrite
``` void fetchData() async {
try {
final List<dynamic> data = await AuthState().getData();
setState(() {
// Convert the fetched data into Expense objects and update the expenses list
expenses = data.map((item) {
return expense(
amount: item['amount'],
date: item['date'],
type: item['type'],
);
}).toList();
});
} catch (e) {
print('Failed to fetch expenses: $e');
}
}```
but i am getting an error stating that the list that iam trying to print is of 0 length
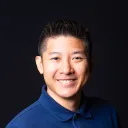
Btw, it's best to use 3 back ticks with multi-line code. See https://www.markdownguide.org/extended-syntax/#syntax-highlighting
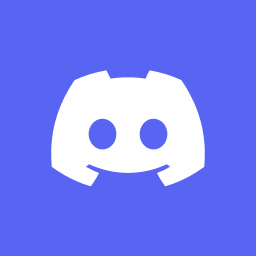
thankyoufor letting me know i will do the same
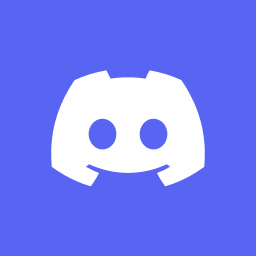
should i resend the code blocks with the same
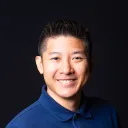
Your getData() returns an empty list. To make things less confusing, I suggest you avoid using them/catchError and use try/await/catch instead
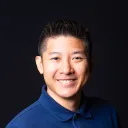
You can edit the post
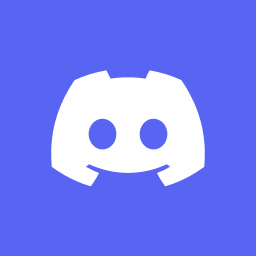
I this right .
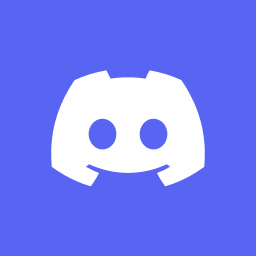
try {
final DocumentList result = await databases.listDocuments(
databaseId: constants.database_ID,
collectionId: constants.collection_ID,
);
return result.documents;
} catch (error) {
print(error);
return [];
}
}}```
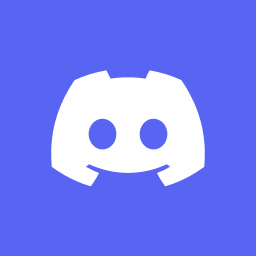
but it is still showing the same error
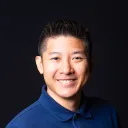
What exactly is the error?
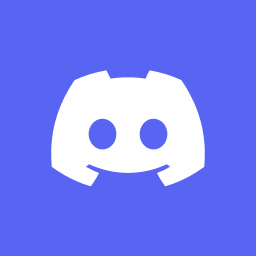
this is the error that i got :- RangeError (index): Invalid value: Valid value range is empty: 0
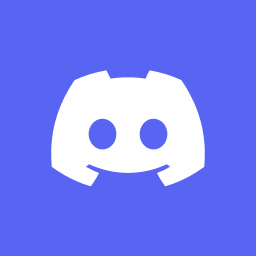
and the screen crashes
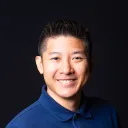
Where is this occurring?
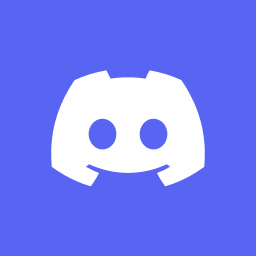
this occurs when i try to call listview.builder
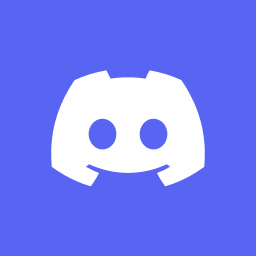
child: ListView.builder(itemBuilder:(context,index){
itemCount: expenses.length;
final expense = expenses[index];
final amount = expense.amount;
final reason = expense.type;
return ListTile(
leading: Text(reason),
title: Text(amount),
);
}),
)```
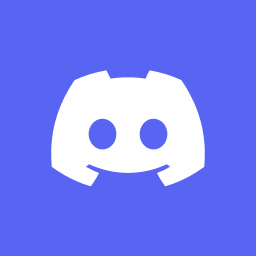
I have cleared the error but i still not getting the expense and type to be on screen
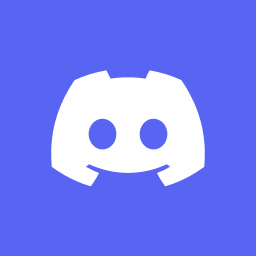
child: ListView.builder(
itemCount: expenses.length,
itemBuilder: (context, index) {
final expense = expenses[index];
final amount = expense.amount;
final reason = expense.type;
return ListTile(
leading: Text(reason),
title: Text(amount),
);
}),
)```
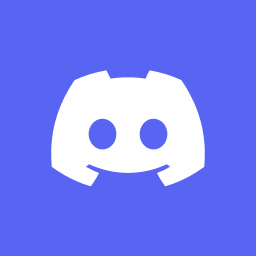
this is the updated code
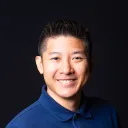
Set a breakpoint at the returns here to see which return is called and what is returned by the list documents call
Recommended threads
- Apple OAuth Scopes
Hi Hi, I've configured sign in with apple and this is the response i'm getting from apple once i've signed in. I cant find anywhere I set scopes. I remember se...
- Sign In With Apple OAuth Help
Hi All! I've got a flutter & appwrite app which Im trying to use sign in with apple for. I already have sign in with google working and the function is the sam...
- [SOLVED] OAuth With Google & Flutter
Hi all, I'm trying to sign in with google and it all goes swimmingly until the call back. I get a new user created on the appwrite dashboard however the flutte...
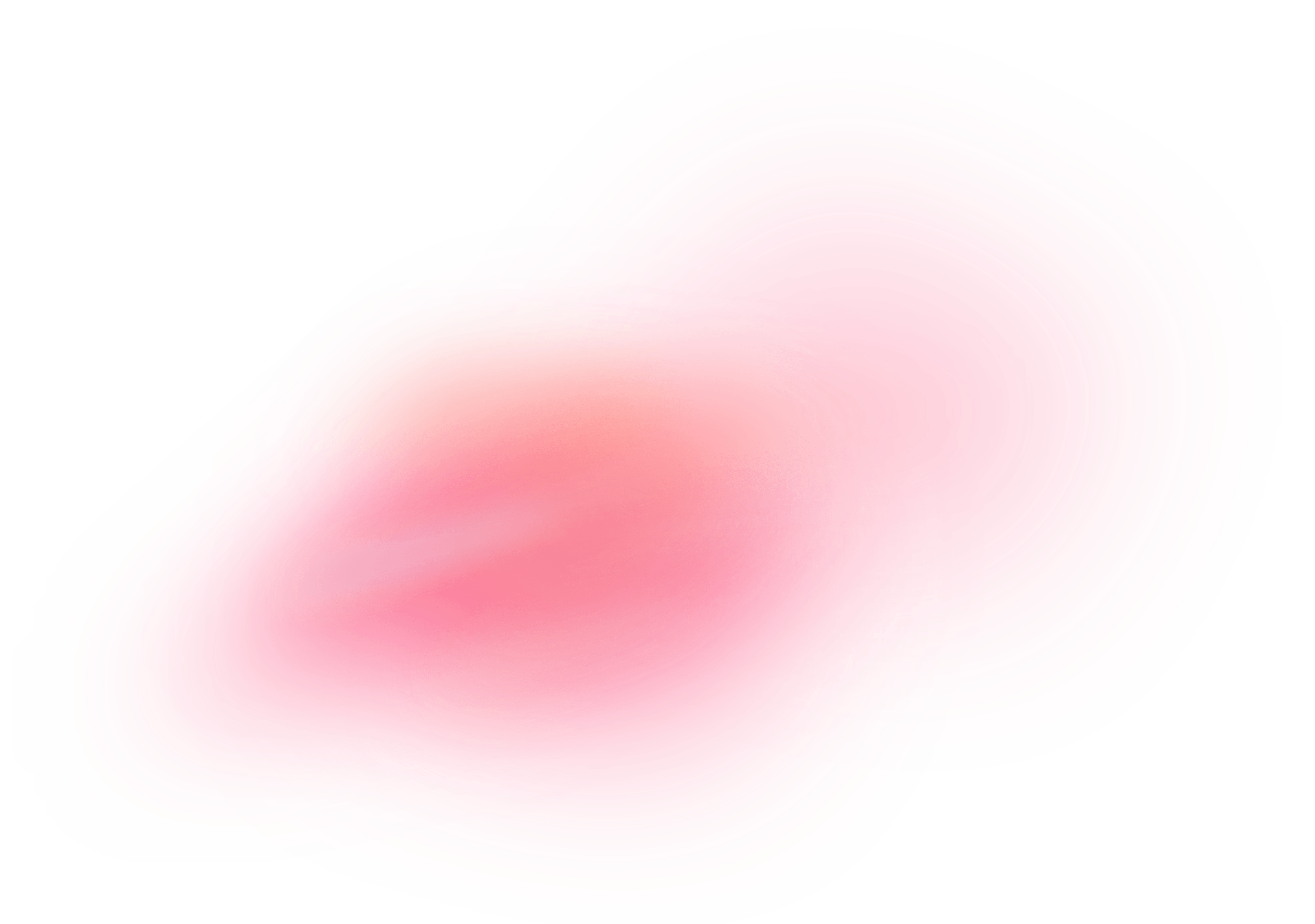