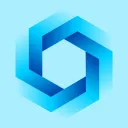
Hello so I am trying to create something so I can add a attribute to a user and I right now have this code but it doesnt seem to be working:
const sdk = require("node-appwrite");
/*
'req' variable has:
'headers' - object with request headers
'payload' - request body data as a string
'variables' - object with function variables
'res' variable has:
'send(text, status)' - function to return text response. Status code defaults to 200
'json(obj, status)' - function to return JSON response. Status code defaults to 200
If an error is thrown, a response with code 500 will be returned.
*/
module.exports = async function (req, res) {
const client = new sdk.Client();
// You can remove services you don't use
const account = new sdk.Account(client);
const avatars = new sdk.Avatars(client);
const database = new sdk.Databases(client);
const functions = new sdk.Functions(client);
const health = new sdk.Health(client);
const locale = new sdk.Locale(client);
const storage = new sdk.Storage(client);
const teams = new sdk.Teams(client);
const users = new sdk.Users(client);
const userId = req.variables["APPWRITE_FUNCTION_USER_ID"];
//get provided token
const token = req.variables["APPWRITE_FUNCTION_DATA"]["token"];
if (
!req.variables["APPWRITE_FUNCTION_ENDPOINT"] ||
!req.variables["APPWRITE_FUNCTION_API_KEY"]
) {
console.warn(
"Environment variables are not set. Function cannot use Appwrite SDK."
);
} else {
client
.setEndpoint(req.variables["APPWRITE_FUNCTION_ENDPOINT"])
.setProject(req.variables["APPWRITE_FUNCTION_PROJECT_ID"])
.setKey(req.variables["APPWRITE_FUNCTION_API_KEY"])
.setSelfSigned(true);
}
var user = await users.get(userId).catch((err) => {
return err;
});
//check if user already has a document in the database predictor-db in colllection users
var userDoc = await database
.listDocuments(`predictor-db`, `users`, [], 1, 0, userId)
.catch((err) => {
return err;
});
// if not create a new one
if (userDoc.documents.length == 0) {
//create a new document in the database predictor-db in colllection users and set the token
await database.createDocument(`predictor-db`, `users`, userId, {
token: token,
});
} else {
//update the token
await database.updateDocument(
`predictor-db`,
`users`,
userDoc.documents[0].$id,
{
token: token,
}
);
}
res.json({
user: user,
});
};
ERROR:
Error: The document payload is missing.
at Client.call (/usr/code-start/node_modules/node-appwrite/lib/client.js:171:31)
at process.processTicksAndRejections (node:internal/process/task_queues:95:5)
at async Databases.createDocument (/usr/code-start/node_modules/node-appwrite/lib/services/databases.js:1055:16)
at async module.exports (/usr/code-start/src/index.js:59:5)
at async /usr/local/src/server.js:68:13```
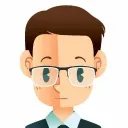
Is the token
const has a value or it's undefined
?
Try to parse the function data as such
const token = JSON.parse(req.variables["APPWRITE_FUNCTION_DATA"] ?? '{}')["token"] ?? '';
Then resend
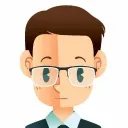
Additionally you can check and send response back if not token was given.
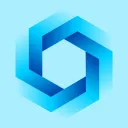
has a value
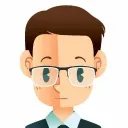
Without parsing?
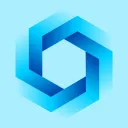
its a long string
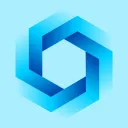
not a array/json
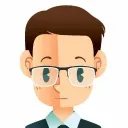
Ohh got it
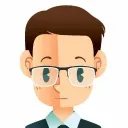
So maybe try to remove the ["token"]
?
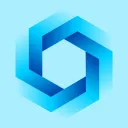
{"variables":{"APPWRITE_FUNCTION_ID":"link_user","APPWRITE_FUNCTION_NAME":"linkUser","APPWRITE_FUNCTION_DEPLOYMENT":"64762868142071342215","APPWRITE_FUNCTION_PROJECT_ID":"hex-predictor","APPWRITE_FUNCTION_TRIGGER":"http","APPWRITE_FUNCTION_RUNTIME_NAME":"Node.js","APPWRITE_FUNCTION_RUNTIME_VERSION":"18.0","APPWRITE_FUNCTION_DATA":"{token:LONGToken"}"}
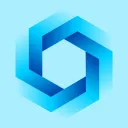
hmmmm it seems to be in a json
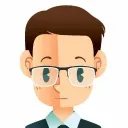
So you just need to parse the token
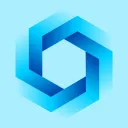
👍
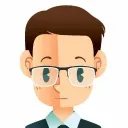
Something like this
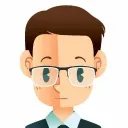
lmn
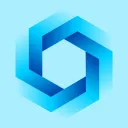
That worked!
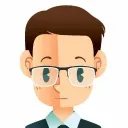
Congrats
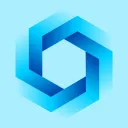
Tnx!
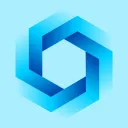
[SOLVED] Function cannot get custom variable
Recommended threads
- self-hosted auth: /v1/account 404 on saf...
Project created in React/Next.js, Appwrite version 1.6.0. Authentication works in all browsers except Safari (ios), where an attempt to connect to {endpoint}/v1...
- delete document problems
i don't know what's going on but i get an attribute "tournamentid" not found in the collection when i try to delet the document... but this is just the document...
- Update User Error
```ts const { users, databases } = await createAdminClient(); const session = await getLoggedInUser(); const user = await users.get(session.$id); if (!use...
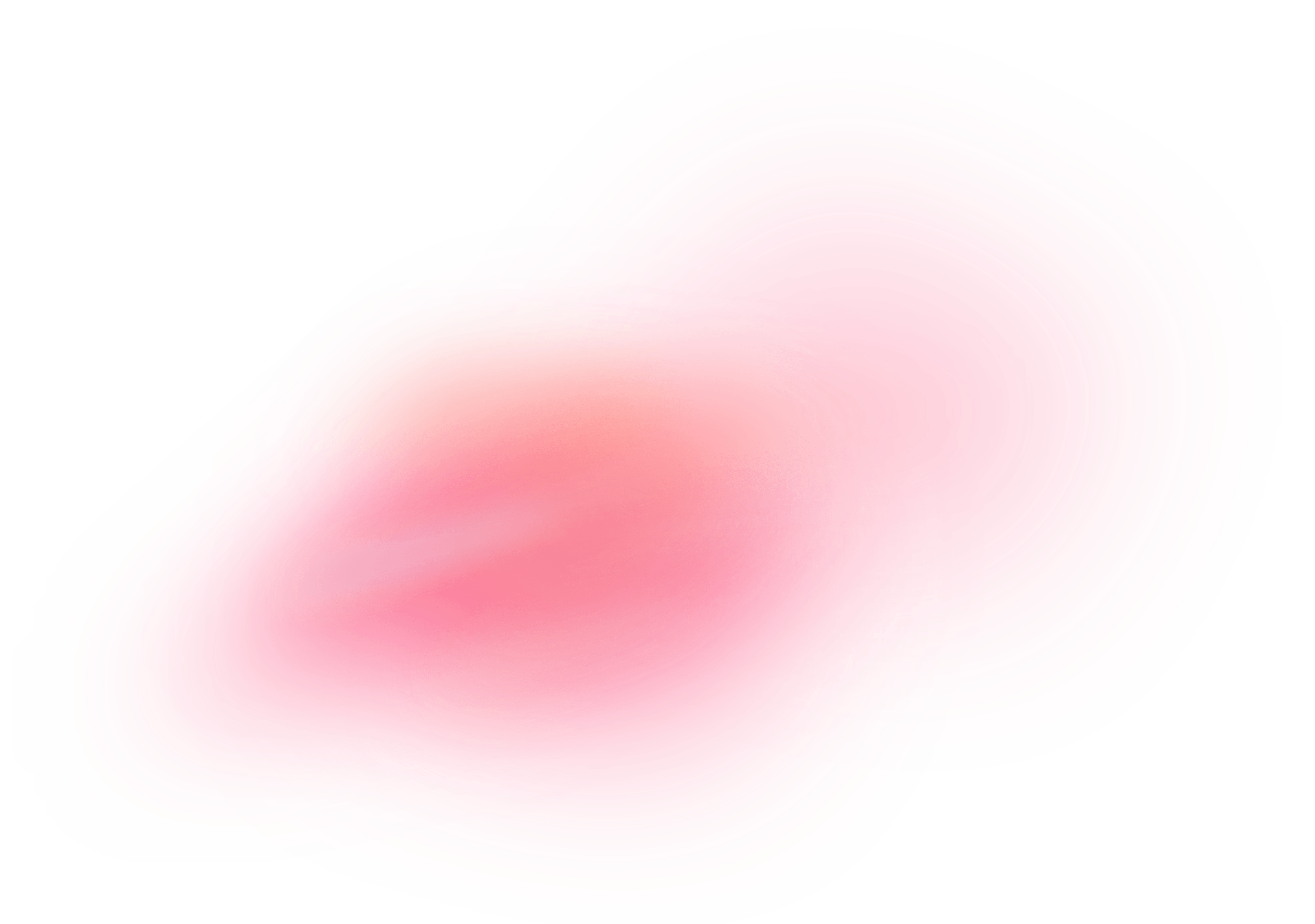