
I'm making a React web-app where users can log in, add a document, but I want them to only be able to view documents they have created. So far I'm just running it locally. Here's how they're being saved:
try { return databases.createDocument( DATABASE_ID, COLLECTION, ID.unique(), newRecipe, [ Permission.delete(Role.user(currUser.$id)), Permission.update(Role.user(currUser.$id)), Permission.read(Role.user(currUser.$id)) ]); }
I'm using account.get() once a user is logged in and saving the user in context, that's how I access the ID.
I look at the new document and it shows the correct permissions based on the ID.
I have document security enabled.
I'm retrieving the documents with: (await databases.listDocuments([DATABASE_ID], [COLLECTION_ID])).documents
But it returns all of the documents, not just the one the current user should be able to view. Should I be filtering the documents somehow? Should I add an attribute that's populated with the user's ID and filter by that? Is it unsecure to be having the user's information on the client side in context...?
I've looked at the appwrite example (https://github.com/appwrite/demo-todo-with-react/tree/main) but can't figure out where I'm going wrong.
I'm only front-end (self-taught) but have made some Jamstack web-apps; I keep trying to turn one into something where users can securely log into and access their data, but have yet to succeed. Don't know if I'm overthinking things or just completely wrong in my attempts.
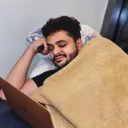
- Does the created document have the correct permissions in the Appwrite console GUI? Just to be sure.
- Saving user info in context shouldn't be an issue, but I suggest storing only the data that is needed. If you're not going to use the hashed password (which is returned when you run
account.get()
, then don't store it. - About the
listDocuments
endpoint returning all the documents, I'm not so sure. Could you provide some more code? Might be a semantic issue that just needs another set of eyes lmao

Thank you so much for your reply!
- I believe so, attached an screenshot of the permissions from a document. I'm using two demo accounts to log in/save data but I see all documents regardless of the account I'm using.
- Excellent point, thanks! I'll change it to save just the user ID.
- I could be goofing up something simple and missing it, I *definitely *didn't waste 30min yesterday tracking down something dumb I'd done lmao
So here's how I get the documents: Helpers for account and database:
const client = new Client(); client .setEndpoint('https://cloud.appwrite.io/v1') .setProject(PROJECT_ID);
export const account = new Account(client); export const databases = new Databases(client, DATABASE_ID);
When a user logs in and goes to the page to view the documents, retrieve them via useEffect:
useEffect(() => { let data = getData();
data.then(
function (response) {
setRecipes(response);
},
function (error) {
console.log('fetch error:', error);
}
);
}, []);
the getData helper:
export const getDocs = async () => { try { return (await databases.listDocuments(DATABASE, COLLECTION)).documents } catch (e) { console.log('get curr user data error:',e) } };
And then the documents are mapped to display them.
Let me know if you want more code than that, thanks again!

Threw the code into my editor to make it less of an eyesore to read, sorry about that.
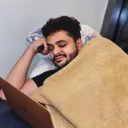
export const databases = new Databases(client, DATABASE_ID);
I don't think that's the format for initializing an instance of Databases
. Try to remove the DATABASE_ID
. You can pass in the database ID when doing createDocument
or something else from the Databases API.
Something I do just to be safe is separating the call to .documents
from the listDocuments
call. It's just a habit. Try doing something like:
try {
var getDocs = await databases.listDocuments(DATABASE, COLLECTION);
return getDocs.documents
}
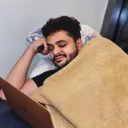
These are just suggestions. I'm not able to spot any real error over here that might be causing the issue.
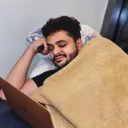
Not an issue! You can use 3 backticks to format code (`) ๐

I tried that but it didn't make the color changes while typing the comment, so I wasn't sure if it worked here. I doubt myself a lot ๐
I made the suggestions you said and I'm still getting all of the documents from both accounts. When setting the read permissions when creating a document, that's all that's needed to allow/restrict viewing of a document, correct? It seems so simple and easy, so what do I have going on wrong lmao I've console logged the user id when logged in, each account has a different one and the docs have different user permissions. I can console log the response from listDocuments
and it has the permissions array with each document object, which is cool to see and again shows the restrictions. Could it have anything to do with running the project locally..?
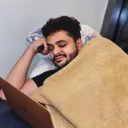
Seems like you're ding everything right tbh. I doubt it's got anything to do with running it locally.
I haven't had to mess with perms for my projects so I might be missing something. Let me do some testing on my end and see if I can get it to work.
Also just to be sure, what version of Appwrite are you using? Is it the cloud version, or a self-hosted one?
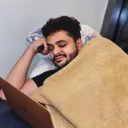
The appwrite version is shown in the bottom right corner when you use the console GUI.

That's kind of what I'd thought, so I was hesitant to post asking for help because I couldn't figure out how I could mess up something so well designed ๐ I'm using react-router if that matters at all, to have protected paths.
I'm using version 1.1.2, cloud version.

Hey @alleycaaat Seems like a same like as mine #[SOLVED] Protect User's Data

It does! Glad I'm not the only one stumped with this. I wonder if it's because for the whole collection the User has permissions? If that is removed, and then specific permissions are granted when the user creates a document? I'll test it out, can't hurt. Edit: that definitely isn't the issue lmao resulted in an error saying the user isn't authorized to perform the requested option, oddly enough ahaha

I don't know what's the actual solution but after setting up Persmission and Role.user() the issue still persists

I just checked by logging out and then logging in from a different account

Same for me, I'm using two accounts and can see all the documents regardless of the account logged in. I might add an attribute, set that to the user's ID and run a Query based on that as an interim solution.

let me know if that works

It does! I created a string attribute to plug the user ID into when saving a document, the index is fullText type to use the search Query, then I send the user ID to the function and query it like so: Query.search('userID',id)
.
I'm not sure if it's the most efficient option, but it's getting the job done.

I did the same kind of thing and it works

[SOLVED] Viewing document based on user's ID
Recommended threads
- Struggling with Sessions
Understanding check: createAnonymousSession() registers the session with the backend setSession() creates a cookie on the userโs computer(?) getSession(โcurr...
- MCP and VSCode Docs
I like your tools, but would like to set up Copilot in VSCode, but these docs don't offer that guide. Did I miss something? https://appwrite.io/docs/tooling/mcp
- How to set wildcard at custom doamin
For OAuth2 redirect, I have set the appwrite api endpoint as appwrite.example.com, and added the CANME in cloudfare where I bought the domain name. And I hosted...
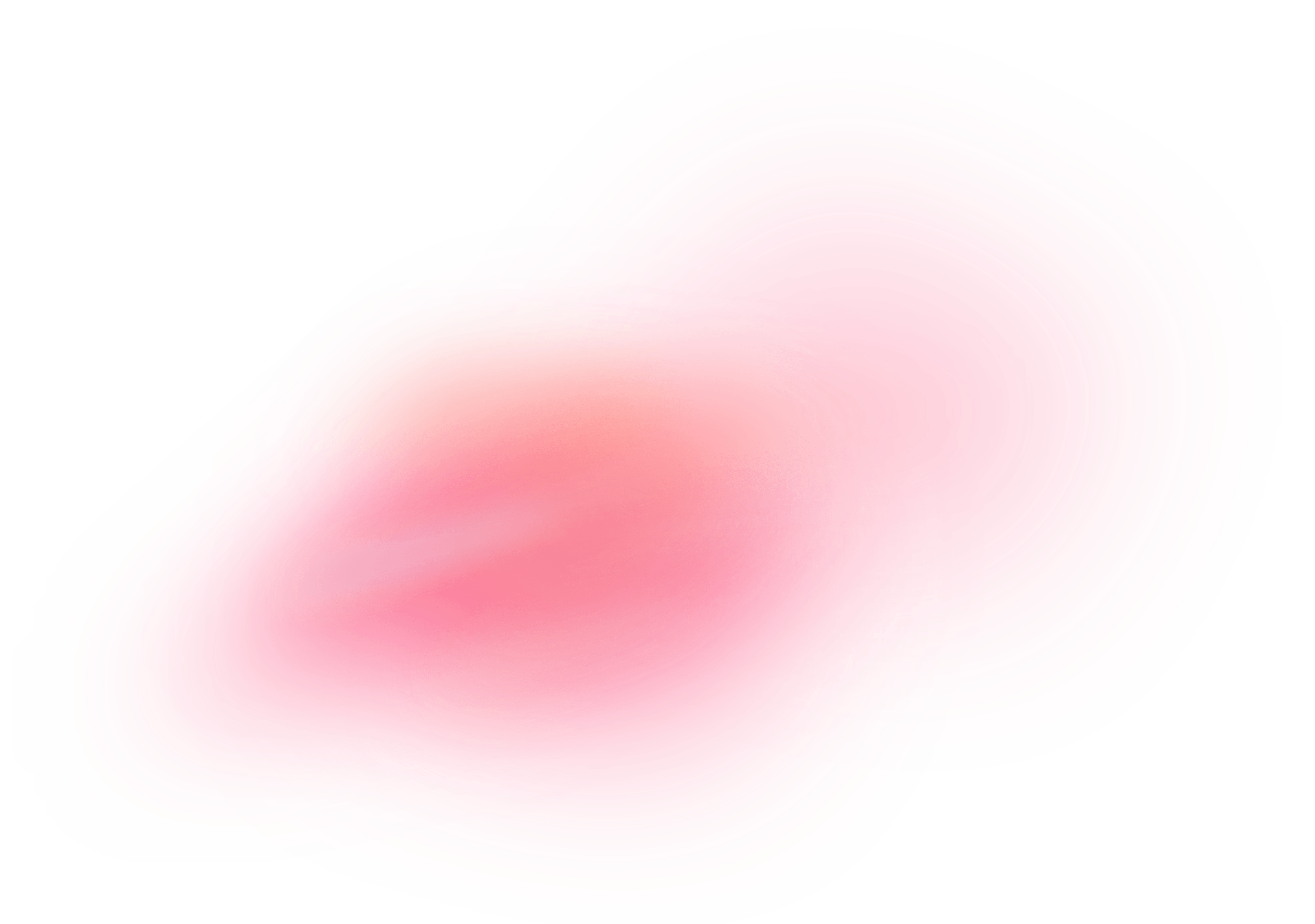