
Hello ! Can you tell me if the function I wrote is well optimized or not ? It have to generate Unique ID like "AZ45DF" for documents when inserting. Thank you for your returns.
const payload = JSON.parse(req.variables["APPWRITE_FUNCTION_EVENT_DATA"]);
const {
$id,
$collectionId
} = payload;
if (!payload.hasOwnProperty("id")) return console.error("No ID attribute in this collection");
let uniqueId = "";
while (true) {
for (let i = 0; i < 6; i++) {
const randomIndex = Math.floor(Math.random() * characters.length);
uniqueId += characters[randomIndex];
}
const idQuery = [
sdk.Query.equal("id", uniqueId),
];
const requestIds = await databases.listDocuments(
req.variables["USERS_DATABASE_ID"],
$collectionId,
idQuery
);
const responseIds = requestIds.documents;
if (responseIds.length === 0) {
break;
} else {
uniqueId = ""
}
}
const json = {
id: uniqueId
};
await databases.updateDocument(req.variables["USERS_DATABASE_ID"], $collectionId, $id, json)
res.json({
success: true,
});
}
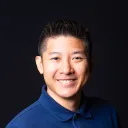
You need to do this because you need the ID to be 6 chars in length?

Yes !
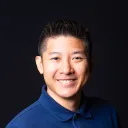
I'm not sure how this collection is used and where you need the speed...some things to consider:
getDocument()
is faster thanlistDocuments()
becausegetDocument()
uses the cache- I would make sure to have a unqiue index to ensure uniqueness at the database level

It's for all collections that have an ID attribute. I need all users, tickets and reservations to have a 6 characters long ID, so it's easily readable and shareable. But getDocument() need the document Id in parameters, so I can't ? And yes I have added an index on the ID attribute but I don't really understand how indexes work...
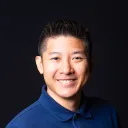
It's for all collections that have an ID attribute. I need all users, tickets and reservations to have a 6 characters long ID, so it's easily readable and shareable. But getDocument() need the document Id in parameters, so I can't ?
Keep in mind, you can use a custom 6 character ID for the document id. Then you can use getDocument()
on that 6 character ID.
I have added an index on the ID attribute but I don't really understand how indexes work...
They just work behind the scenes. You don't really do anything. They speed up queries and provide restrictions (if it's a unique index)

how can I use a custom 6 characters ID as the document ID ? With createDocument()#documentId parameter ?
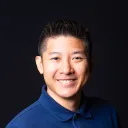
yes, you pass ID.custom(yourId)
instead of ID.unique()

Oh okay I thought It needed to be formatted like default ID.unique(). Thank you very much. So I can add an index on the documentId ?
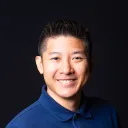
there's no need to add an index on the document ID


How do I know if the document was found please ?

In nodeJS

with then() catch() ?

ty

Steven, can I change a document ID in a function ?

I can't handle making 2 requests in my apps just to check if the ID already exists before creating a document

I think I need to do this with a function
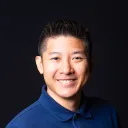
No, you can't change a document id
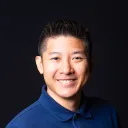
I mean...you can just try to create it...if it throws an error saying it's a duplicate, try again


ty
Recommended threads
- Project in AppWrite Cloud doesn't allow ...
I have a collection where the data can't be opened. When I check the functions, there are three instances of a function still running that can't be deleted. The...
- Get team fail in appwrite function
I try to get team of a user inside appwrite function, but i get this error: `AppwriteException: User (role: guests) missing scope (teams.read)` If i try on cl...
- Function in Node.JS to monitor events ar...
Hello everyone. I'm creating my first Node.JS function, but I don't have much experience with node and javascript. I'm trying to create a function, that monito...
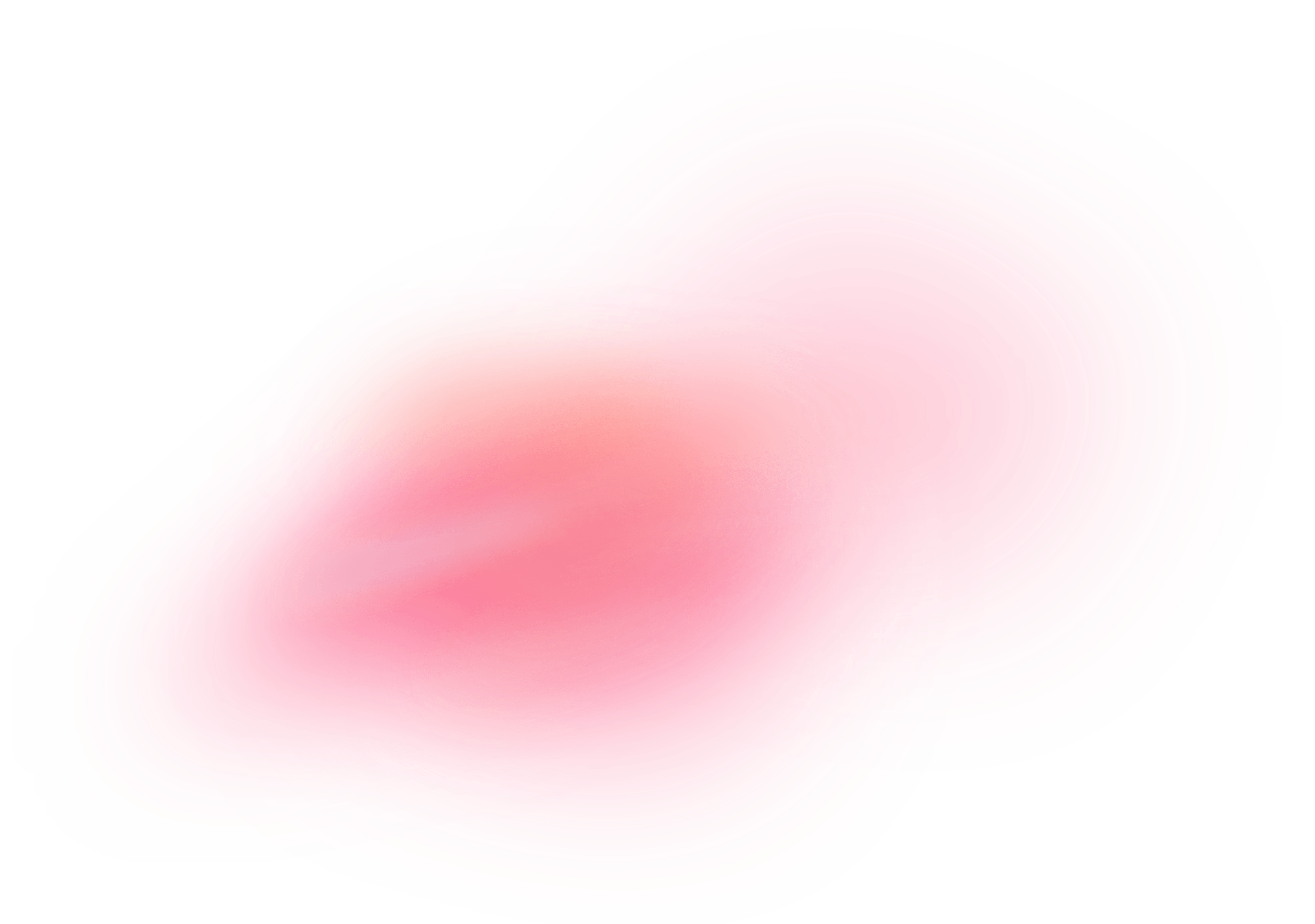