
Image 1: https://i.imgur.com/Mxr5aiU.png
Code:
import { NextResponse } from "next/server"
import client, { Account, ID } from "@/lib/appwrite/appwrite"
export async function GET(request: Request) {
// Parse the URL and get the query parameters
const url = new URL(request.url)
const userId = url.searchParams.get("userId")
const secret = url.searchParams.get("secret")
if (!userId || !secret) {
return NextResponse.redirect(process.env.NEXT_PUBLIC_APP_URL || "", 302)
}
try {
const updateURL = Account.updateMagicURLSession(userId, secret)
updateURL.then(
function (response) {
console.log(response)
return NextResponse.redirect(
process.env.NEXT_PUBLIC_APP_URL + "/dashboard",
302
)
},
function (error) {
console.log(error)
throw new Error(error.message)
}
)
// return NextResponse.redirect(
// process.env.NEXT_PUBLIC_APP_URL + "/dashboard",
// 302
// )
} catch (error) {
// console.error(error)
return NextResponse.redirect(process.env.NEXT_PUBLIC_APP_URL || "", 302)
}
// return NextResponse.redirect(
// process.env.NEXT_PUBLIC_APP_URL + "/dashboard" || "",
// 302
// )
}```
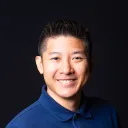
What's the error you're getting? Is this code being executed server side?

clientside
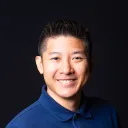
Wait...I see request and next response...this looks like it's executed server side 🧐

its a GET request, i redirect from the login button to the api route and it redirects them back to the dash
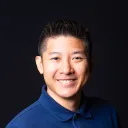
API route...that's executed server side, isn't it?

I'm not sure 😂
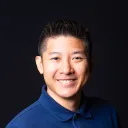
This is the really confusing thing about using frameworks with server side rendering. with server side rendering you have:
Client (browser) <-> NextJS Server-side <-> Appwrite
If you're creating a session at NextJS Server-side, it doesn't get persisted or attached to the user and just gets lost

So how would i do Magic URLs?

I did the first part, i just dont get the confirmation side of things
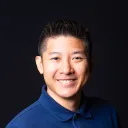
You would need to make sure the code is executed client side.
I highly suggest turning off all server-side rendering if you don't really need it. it complicates things a lot. with server-side rendering enabled magic url and oauth2 may not work because the cookie is set client side and the server-side nextjs code doesn't have access to it.

Sure ill try that 😂

im using next.js 13 for my frontend
/** @type {import('next').NextConfig} */
const nextConfig = {
reactStrictMode: true,
output: 'export',
distDir: 'dist',
images: {
unoptimized: true,
},
}
module.exports = nextConfig
I'm building it as static generated files that any web server can serve That ensures things are client side, if you use the /api routes, then it is definitely server side

I haven't gotten deep into their new app directory stuff yet though, I think that is different

you could also pass through the headers to the appwrite api as well and still do things server side

@jSnake🐍🕊 I appreciate that, still trying to figure out the new Next JS v13

I want to create a template so i can copy paste modules into my projects

and for instance

instantly link login/register stuff
Recommended threads
- Query Appwrite
Hello, I have a question regarding Queries in Appwrite. If I have a string "YYYY-MM", how can I query the $createdAt column to match this filter?
- Different appwrite IDs are getting expos...
File_URL_FORMAT= https://cloud.appwrite.io/v1/storage/buckets/[BUCKET_ID]/files/[FILE_ID]/preview?project=[PROJECT_ID] I'm trying to access files in my web app...
- Invalid document structure: missing requ...
I just pick up my code that's working a week ago, and now I got this error: ``` code: 400, type: 'document_invalid_structure', response: { message: 'Inv...
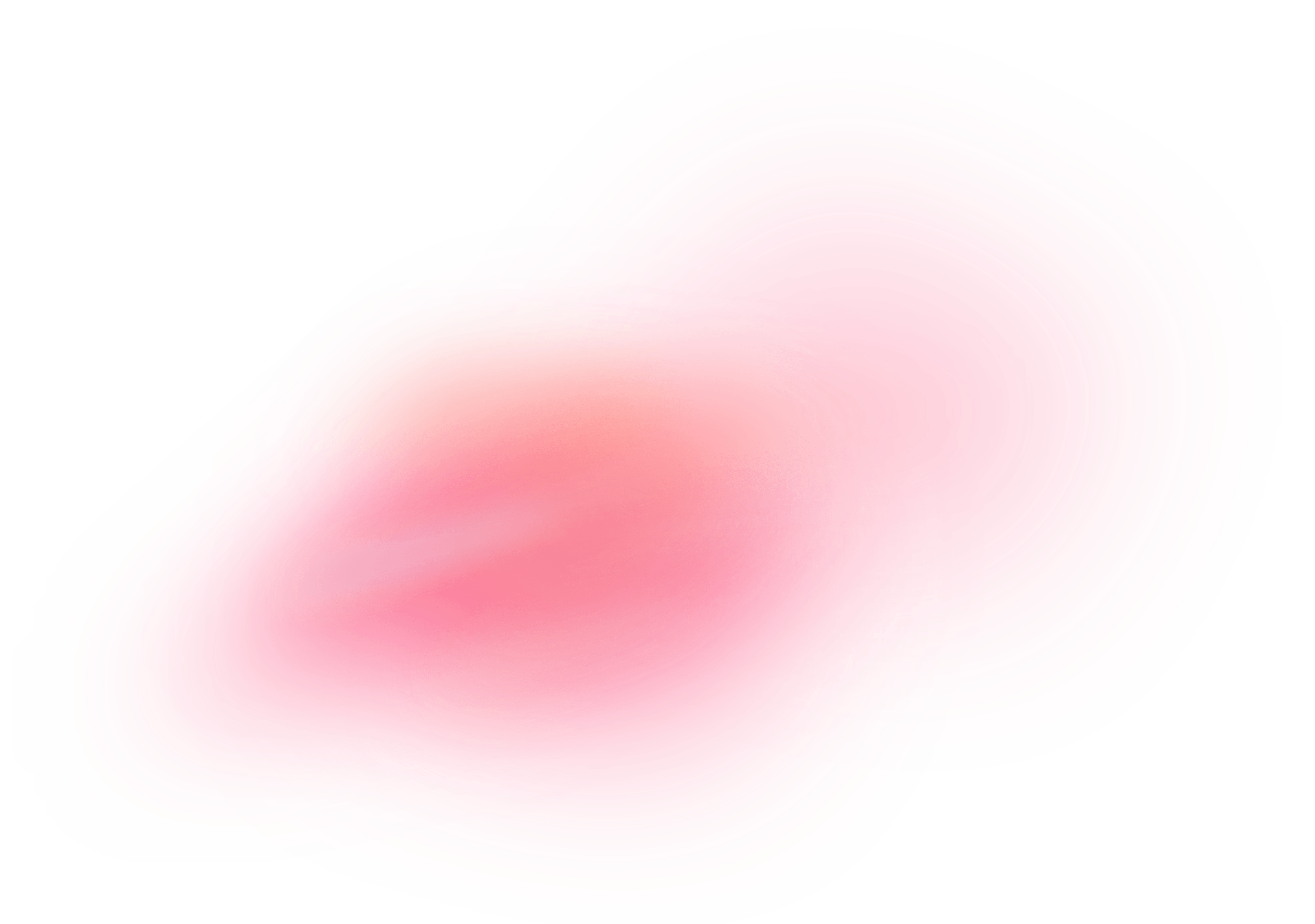