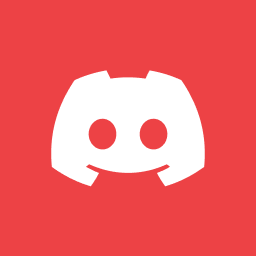
I am building a migration function that removes and recreates database collections and their indexes, permissions and records on demand.
The wrapper function:
export async function migrate (database, client, app) {
await remove(database)
await create(database)
await attributes(database)
await indexes(database)
await permissions(database)
await seed(database)
}
In the #attributes
function, I create several attributes:
export async function attributes (database) {
const promises = [
database.createStringAttribute(DATABASE, COLLECTION, 'code', 16, true),
database.createBooleanAttribute(DATABASE, COLLECTION, 'active', true),
database.createStringAttribute(DATABASE, COLLECTION, 'person', 16384, true),
database.createStringAttribute(DATABASE, COLLECTION, 'personLabel', 128, true),
database.createEmailAttribute(DATABASE, COLLECTION, 'personEmail', true),
database.createStringAttribute(DATABASE, COLLECTION, 'personPhone', 16, true),
database.createStringAttribute(DATABASE, COLLECTION, 'organization', 16384, true),
database.createStringAttribute(DATABASE, COLLECTION, 'organizationLabel', 128, true)
]
return Promise.all(promises)
}
NOTE these are async functions, so I await Promise.all(promises)
By my understanding, the library fn #create<TYPE>Attribute
should resolve when the attribute is created ....
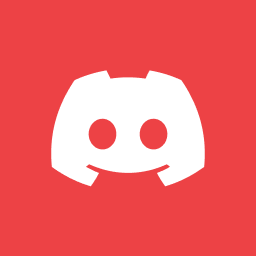
However, in the subsequent #indexes
function, an error is thrown:
export async function indexes (database) {
const promises = [
database.createIndex(DATABASE, COLLECTION, 'code', 'key', [ 'code' ], [ 'asc' ])
]
return Promise.all(promises)
}
... with the error:
AppwriteException [Error]: Attribute not available: code
at Client.call (/Users/darren/Development/svelte/register/node_modules/.pnpm/node-appwrite@8.2.0/node_modules/node-appwrite/lib/client.js:171:31)
at processTicksAndRejections (node:internal/process/task_queues:96:5)
at async Databases.createIndex (/Users/darren/Development/svelte/register/node_modules/.pnpm/node-appwrite@8.2.0/node_modules/node-appwrite/lib/services/databases.js:1245:16)
at async Promise.all (index 0)
at async migrate (file:///Users/darren/Development/svelte/register/database/signups/collection.js:18:3)
at async migrate (file:///Users/darren/Development/svelte/register/database/index.js:8:3) {
code: 400,
type: 'attribute_not_available',
response: {
message: 'Attribute not available: code',
code: 400,
type: 'attribute_not_available',
version: '1'
}
}
Is this expected behavior or a bug? Surely when the create<TYPE>Attribute function resolves, the attribute must have been created?
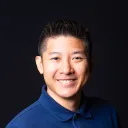
Surely when the create<TYPE>Attribute function resolves, the attribute must have been created?
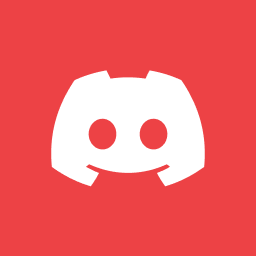
Oooh. No. That's just not cool.
Makes it V-E-R-Y difficult to create migrations that require these attributes in order to create the indexes, don't you think?!
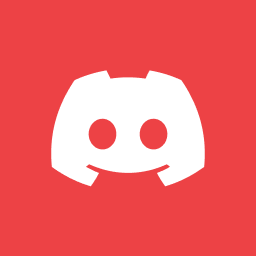
So I guess what ... ? I should fire an event and listener function that polls for this to complete ... ? How are others handling this?
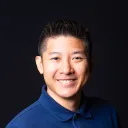
You would have to check the status and proceed like our CLI does
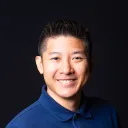
You can poll the collection and check the status
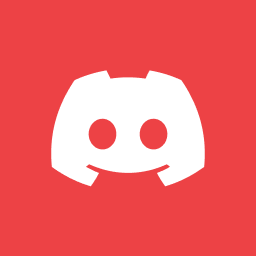
Yeah. I guess I'll have to. It's not tight though.
Recommended threads
- phantom relationships appear on parent c...
i have this bug were my past deleted collection apears as relationship to my parent collection. when i try to delete that relationship from parent it gives me e...
- Attribute stuck on proccessing
i tried creating a new attribute butits stuck on proccessing,i did a hard refresh,cleared cache everything but still stuck on proccessing,also in my functions w...
- Appwrite Cloud Custom Domains Issue
I’m trying to configure my custom domain appwrite.qnarweb.com (CNAME pointing to fra.cloud.appwrite.io with Cloudflare proxy disabled) but encountering a TLS ce...
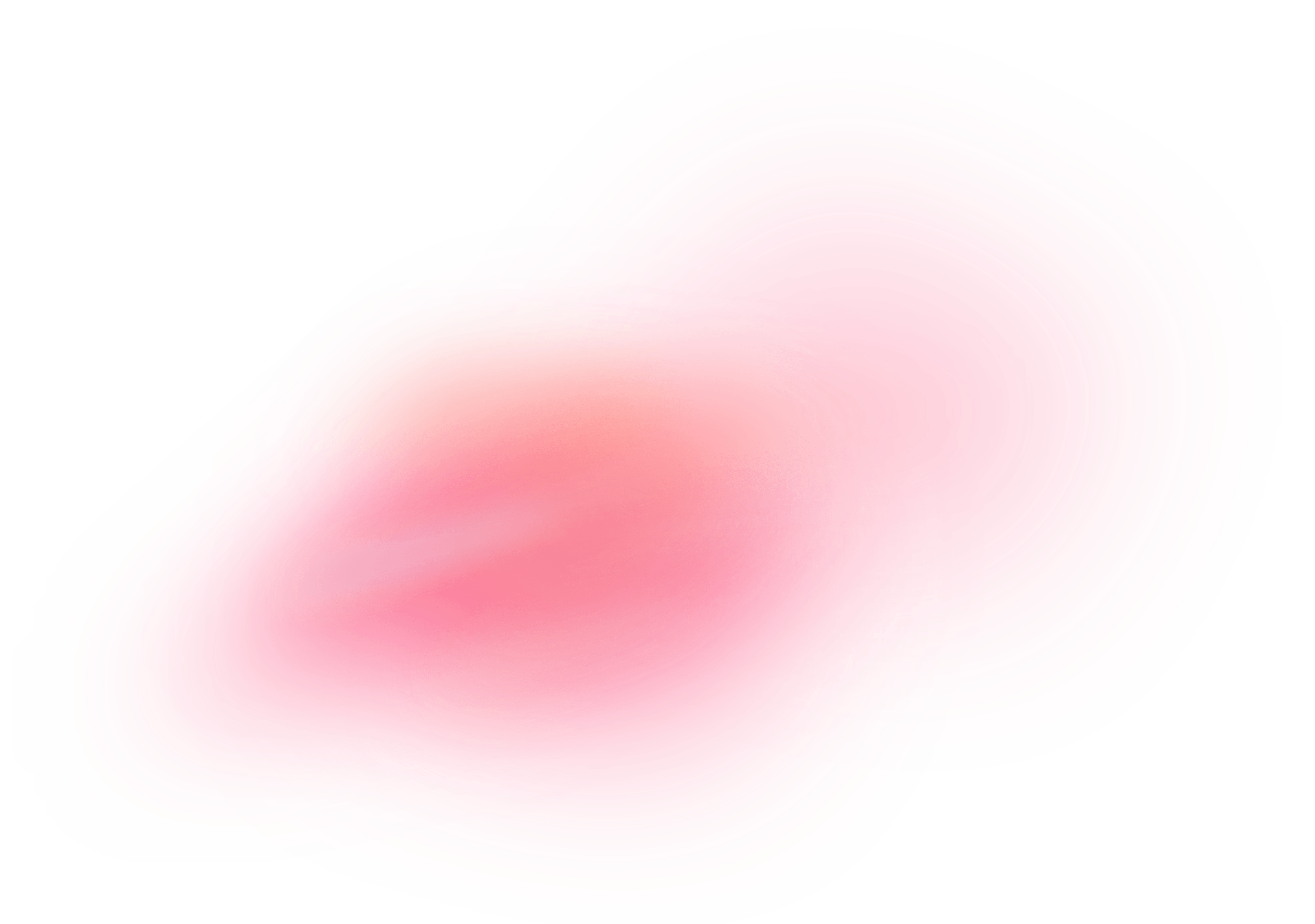