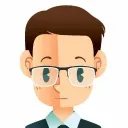
You want to be able to set document permission for the current user is that right?
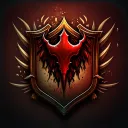
yes
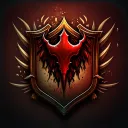
I am having trouble figuring out how to hand off the info to the server side once its created on the client side
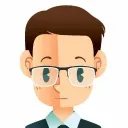
Then the easiest solution would be send the either the JWT
or the userID
to the backend.
Other option it's only what Steven suggested to fully managed the session in the backend
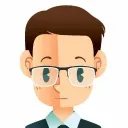
Can you show the code example of when you're sending the data from the front to the back?
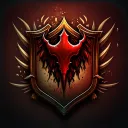
Im using svelte so it weird
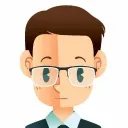
It's a bit different, yes
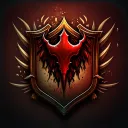
/login/+page.svelte (client Side)
import "../../app.css";
import loginimg from "$lib/assets/login.jpg";
import { Client, Account } from "appwrite";
import { enhance } from "$app/forms";
import { goto } from "$app/navigation";
import { accountId } from "$lib/stores.js";
const client = new Client();
const accountClient = new Client();
const account = new Account(client);
client
.setEndpoint("http://localhost:3080/v1") // Your API Endpoint
.setProject("63db6c4c0a25ec0b287d"); // Your project ID
async function handleSubmit() {
const createJWT = account.createJWT();
const formData = new FormData(event.target);
const email = formData.get("email");
const password = formData.get("password");
accountClient
.setEndpoint("http://localhost:3080/v1")
.setProject("63db6c4c0a25ec0b287d")
.setJWT(createJWT);
const accountDetails = await account.get();
accountId.set(accountDetails.$id);
// Form Input
try {
const response = await account.createEmailSession(email, password);
console.log(response); // Success
goto("/msform");
return response;
} catch (error) {
console.log(error); // Failure
console.log(email, password);
throw error;
}
}```
/msfrom/+page.server.js (Server Side)
import sdk, { ID } from 'node-appwrite'; import { accountId } from '$lib/stores';
// Init SDK const client = new sdk.Client();
const databases = new sdk.Databases(client);
client .setEndpoint('http://localhost:3080/v1') // Your API Endpoint .setProject('63db6c4c0a25ec0b287d') // Your project ID .setKey(' ') // Your secret API key ;
export const actions = { default: async ({request}) => {
const fromData = await request.formData();
const name = fromData.get('name');
const DOB = fromData.get('DOB');
const feet = fromData.get('feet');
const inch = fromData.get('inch');
const weight = fromData.get('weight');
const school = fromData.get('school');
const sport = fromData.get('sport');
const position = fromData.get('position');
const age = fromData.get('age');
const better = fromData.get('better');
const input = {
Name: name,
DOB: DOB,
Feet: feet,
Inch: inch,
Weight: weight,
School: school,
Sport: sport,
Position: position,
Age: age,
Better: better
};
const promise = databases.createDocument('64502243369b884d71f8', '6450225bbca140600f16', ID.unique(), input, );
console.log(JSON.stringify(accountId));
}
}```
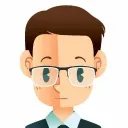
Okay, I see
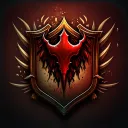
I was trying to use svelte stores but it was not working
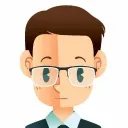
You need to change a few thing in your code logic, I can point them to you.
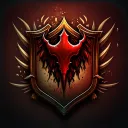
Ok
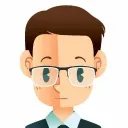
In the /login/+page.svelte
page
- You don't need to have more then on client.
account.creaeJWT
returns a promise.account.get
will throw error in case the user is not logged in. and in case the user indeed logged in then you don't need to use thecreateEmailSession
function as the user already exist.- the
setJWT
need to be used only in the server side of the SDK, although you can set it in the client side, it's not necessary as the session will be handled for you.
So this file can be something like this
import "../../app.css";
import loginimg from "$lib/assets/login.jpg";
import {Client, Account} from "appwrite";
import {enhance} from "$app/forms";
import {goto} from "$app/navigation";
import {accountId} from "$lib/stores.js";
const client = new Client();
const accountClient = new Client();
const account = new Account(client);
client
.setEndpoint("http://localhost:3080/v1") // Your API Endpoint
.setProject("63db6c4c0a25ec0b287d"); // Your project ID
async function handleSubmit() {
let userLoggedIn = false;
try {
// Get account if logged in
const accountDetails = await account.get();
accountId.set(accountDetails.$id);
userLoggedIn = true;
} catch (e) {
}
if (!userLoggedIn) {
try {
const formData = new FormData(event.target);
const email = formData.get("email");
const password = formData.get("password");
const response = await account.createEmailSession(email, password);
console.log(response); // Success
} catch (e) {
console.log(error); // Failure
console.log(email, password);
throw error;
}
}
const createJWT = await account.createJWT();
try {
goto("/msform");
return response;
} catch (error) {
}
}
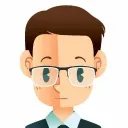
And in your server side
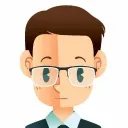
You can get the user ID also from the form data, and you won't need to use store
import sdk, {ID} from 'node-appwrite';
// Init SDK
const client = new sdk.Client();
const databases = new sdk.Databases(client);
client
.setEndpoint('http://localhost:3080/v1')
.setProject('63db6c4c0a25ec0b287d')
.setKey(''); // Create and API key from the console.
export const actions = {
default: async ({request}) => {
const fromData = await request.formData();
const name = fromData.get('name');
const DOB = fromData.get('DOB');
const feet = fromData.get('feet');
const inch = fromData.get('inch');
const weight = fromData.get('weight');
const school = fromData.get('school');
const sport = fromData.get('sport');
const position = fromData.get('position');
const age = fromData.get('age');
const better = fromData.get('better');
//TODO: Add the user in the client side
const userID = fromData.get('user_id');
const input = {
Name: name,
DOB: DOB,
Feet: feet,
Inch: inch,
Weight: weight,
School: school,
Sport: sport,
Position: position,
Age: age,
Better: better,
};
const promise = databases.createDocument(
'64502243369b884d71f8',
'6450225bbca140600f16',
ID.unique(),
input,
[
Permission.write(Role.user(userID)),
]
);
}
}
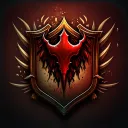
Do I just need to use this? https://appwrite.io/docs/client/account?sdk=web-default#accountGet
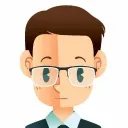
Yes you can use this to check if the user is logged in
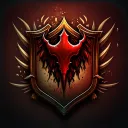
So I dont need the jwt?
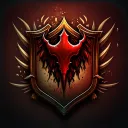
to do what I am doing
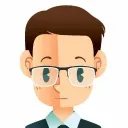
In this specific use case you don't have to use the JWT way.
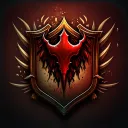
So I have to do now it export it correct? to the serverside?
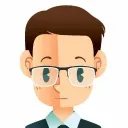
I think so, yes
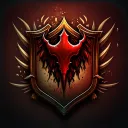
@Steven This is solved thank you
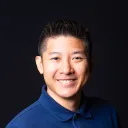
FYI, you can update the post title to prefix with [SOLVED]
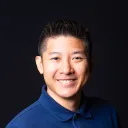
[SOLVED] How do I get sessionId for api endpoint
Recommended threads
- Use cloudflare origin certificate instea...
Hello! Is it possible to use cloudflare origin certificates instead of let's encrypt? We manage all ssl certs in our cloudflare account and our VMs has a firewa...
- Unable to View / Edit Bucket Files
Hi! I am unable to view / edit Bucket Files. While Previews work just fine, clicking the actual file to view or edit it produces the errors seen in the attache...
- How to remove the Sign up link after cre...
Greetings, i just installed appwrite on a VPS and created an account but now i do not want others to have access to the sign-up page. Is there any way to hide o...
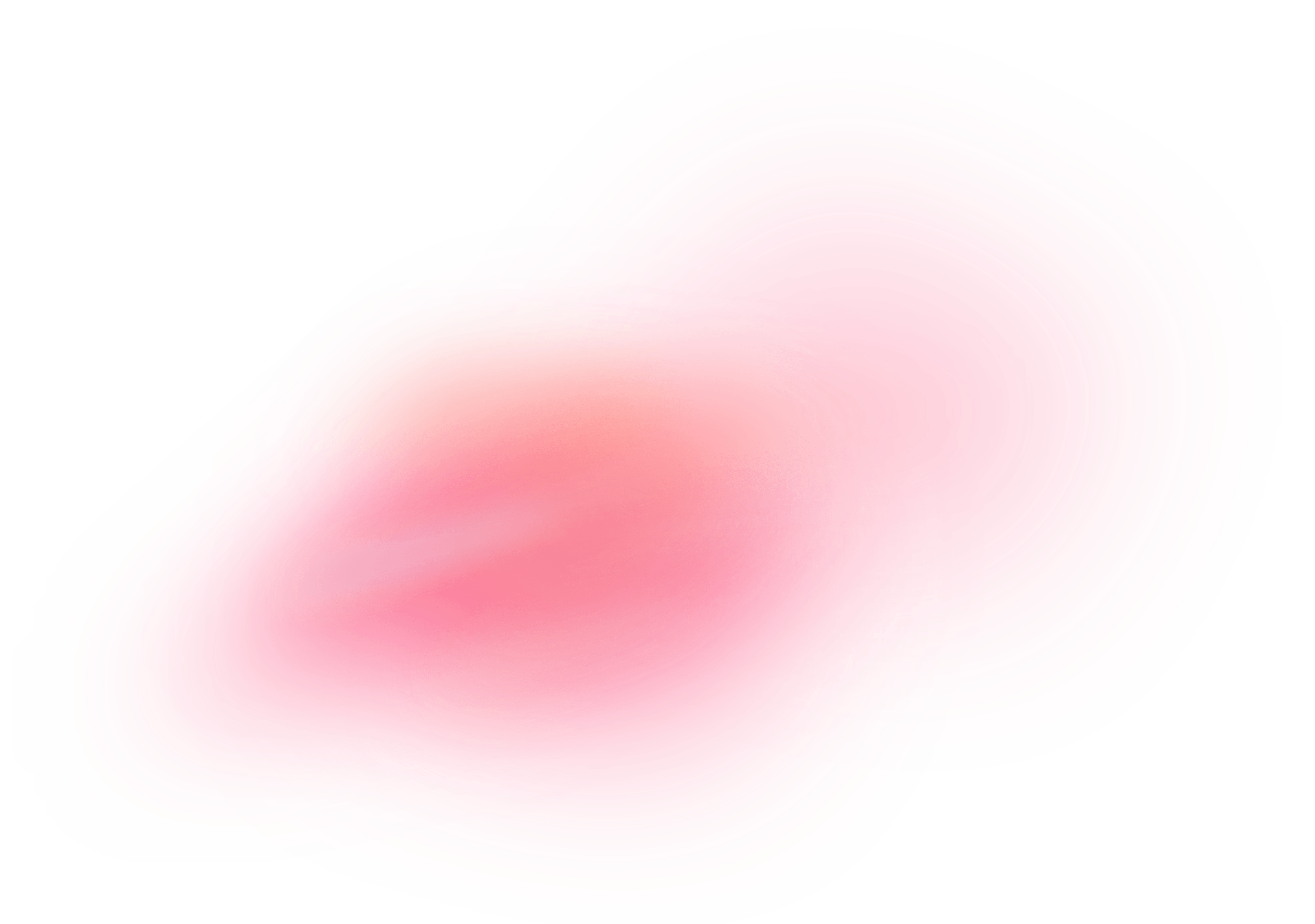