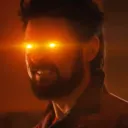
Hi!
I have a project in Flutter and Appwrite.
I only have the ip-address as a URL I created a password recovery page and this is what my page code looks like:
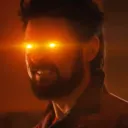
class PasswordResetScreen extends StatelessWidget {
const PasswordResetScreen({Key? key}) : super(key: key);
Future<void> resetPassword(BuildContext context, String email) async {
try {
final account = Account(client);
await account.createRecovery(email: email, url: 'http://192.168.1.1');
showDialog(
context: context,
builder: (_) => AlertDialog(
title: Text('Email sent'),
content: Text(
'An email with password recovery instructions was sent to $email.'),
actions: [
TextButton(
child: Text('OK'),
onPressed: () => Navigator.of(context).pop(),
),
],
),
);
} on AppwriteException catch (e) {
showDialog(
context: context,
builder: (_) => AlertDialog(
title: Text('Error'),
content:
Text('Failed to send password recovery request.'),
actions: [
TextButton(
child: Text('OK'),
onPressed: () => Navigator.of(context).pop(),
),
],
),
);
print('Error: ${e.message}');
}
}
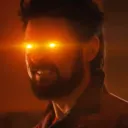
@override
Widget build(BuildContext context) {
final TextEditingController _emailController = TextEditingController();
return Scaffold(
appBar: AppBar(
title: Text('Restore password'),
),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
TextFormField(
controller: _emailController,
keyboardType: TextInputType.emailAddress,
decoration: InputDecoration(
labelText: 'Email',
hintText: 'Enter your email address',
border: OutlineInputBorder(),
),
validator: (value) {
if (value == null || value.isEmpty) {
return 'Please enter your email address';
}
if (!RegExp(r'\b[\w\.-]+@[\w\.-]+\.\w{2,4}\b')
.hasMatch(value)) {
return 'Please enter a valid email address';
}
return null;
},
),
SizedBox(height: 16.0),
ElevatedButton(
child: Text('Send'),
onPressed: () {
if (_emailController.text.isNotEmpty) {
resetPassword(context, _emailController.text.trim());
}
},
),
],
),
),
);
}
}
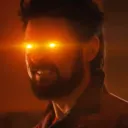
Then the user requests password recovery through this form and successfully receives an email with the following content:
Hello
Follow this link to reset your notekeep password.
If you didn’t ask to reset your password, you can ignore this message.
Thanks notekeep team
Next, the user clicks the link and is redirected to the common Appwrite admin login page:
nothing else happens. The user can't retrieve his password any further.
What else do I need to do to restore the user's password?
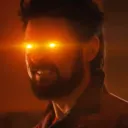
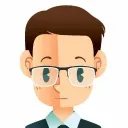
The whole process of resetting a user password is semi-automatic way.
You'll first need to create a reset link and to the createRecovery
function you need to provide URL.
But, the URL shouldn't be the address of your server. This URL should a link to a reset page, And within this reset page you'll need to create a form that let the user to enter new password. Then you'll need to submit that form with the function updateRecovery
providing the reset
token, user ID and password.
Because you're using flutter you have two options
A. Deep-linking / Dynamic links and set the reset URL as something that could be recognized by your app.
B. The easy and more common one is to create mini-site just to for resetting the password.
I've Attached a diagram
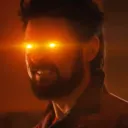
Does anyone have a sample code page for password recovery, for example in php or any other language?
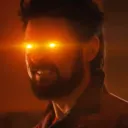
Can I create such a page with the password update form in Flutter for WEB and then just upload it to the hosting?
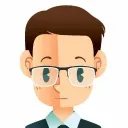
I think you can, yes
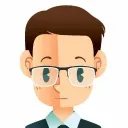
Here you can see an example in <:react:637383195503099915> First sending the recovery request https://github.com/D3nn7/worktime-tracker/blob/b74dd5bf3f91f3ab3f021476ff8910695437b83a/worktime-tracker-dev/pages/account/reset.tsx#L23
And here you can see the how you change the password https://github.com/D3nn7/worktime-tracker/blob/b74dd5bf3f91f3ab3f021476ff8910695437b83a/worktime-tracker-dev/pages/account/reset.tsx#L33
Recommended threads
- Phone auth - how to check if phone numbe...
Hello, I am using flutter and doing phone authentication. I would like to check if the phone number entered in the register screen is already registered and v...
- Unable to open aac files in browser
I am a flutter app, when the user uploads an aac audio file, it gets stored in the bucket The MIME type is audio/x-hx-aac-adts When i try to open that link in...
- CreatePhoneSession Flutter
The method 'createPhoneSession' isn't defined for the type 'Account'. Try correcting the name to the name of an existing method, or defining a method named 'cre...
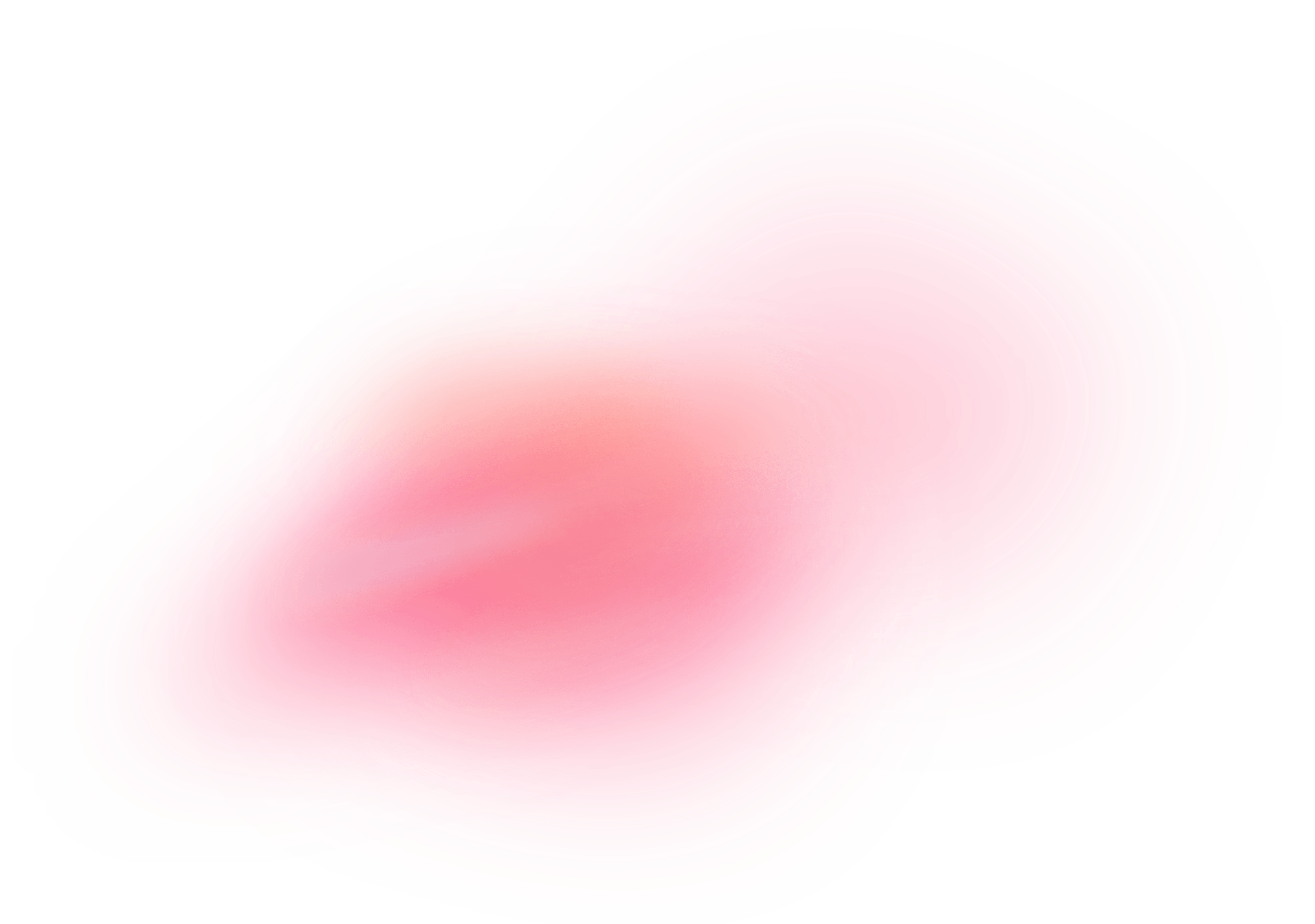