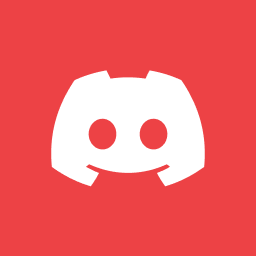
appwrite: ^9.0.0 ## flutter appwrite package version
E/flutter ( 5044): [ERROR:flutter/runtime/dart_vm_initializer.cc(41)] Unhandled Exception: WebSocketException: Connection to [LINK] was not upgraded to websocket.
I am building a flutter app with Riverpod state management.
<code>sample code </code>
final appWriteRealtimeProvider = Provider((ref) { final client = ref.watch(appWriteClientProvider); return Realtime(client); });
final getLatestEweetProvider = StreamProvider((ref) { final eweetAPI = ref.watch(eweetAPIProvider); return eweetAPI.getLatestEweet(); });
@override Stream<RealtimeMessage> getLatestEweet() { return _realtime.subscribe([ 'databases.${KAppWrite.databaseId}.collections.${KAppWrite.eweetsCollection}.documents' ]).stream; }
// UI class EweetList extends ConsumerWidget { const EweetList({super.key});
@override Widget build(BuildContext context, WidgetRef ref) { return ref.watch(getEweetsProvider).when( data: (eweets) { return ref.watch(getLatestEweetProvider).when( data: (data) { return ListView.builder( itemCount: eweets.length, itemBuilder: (c, i) { return Text(i.toString()); }, ); }, error: (e, st) { log("STack trace is ${st}"); return ErrorText(error: e.toString()); }, loading: () { return ListView.builder( itemCount: eweets.length, itemBuilder: (c, i) { return TweetCard(tweet: eweets[i]); }, ); }, ); }, error: (e, st) { log("STack trace is ${st}"); return ErrorText(error: e.toString()); }, loading: () => Loader(), ); } }
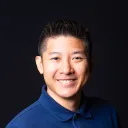
FYI, it's best to wrap code in backticks to format a bit nicer. You can use 1 backtick for inline code (https://www.markdownguide.org/basic-syntax/#code) and 3 backticks for multiline code (https://www.markdownguide.org/extended-syntax/#syntax-highlighting.
And, it's Appwrite, not AppWrite. 😛
Lastly, what's your code for the appwriteClientProvider? And do you have anything running in between your app and Appwrite?
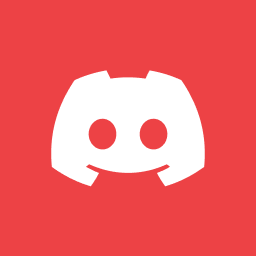
Ok that is good advice. I am just a beginner that is why.
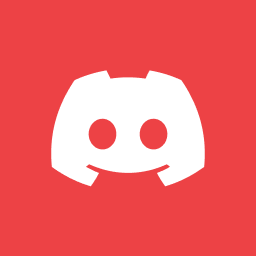
final appWriteClientProvider = Provider((ref) {
Client client = Client();
return client
.setEndpoint(KAppWrite.baseEndPointUrl)
.setProject(KAppWrite.projectId)
.setSelfSigned(status: false);
});
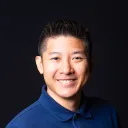
no worries! welcome!
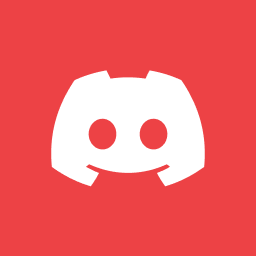
And there is noting in between the appwrite and the app. i am running from docker
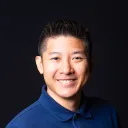
this looks mostly okay...what's your endpoint url?
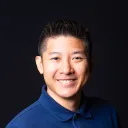
and what do you have set for the _APP_OPTIONS_FORCE_HTTPS
env var for Appwrite?
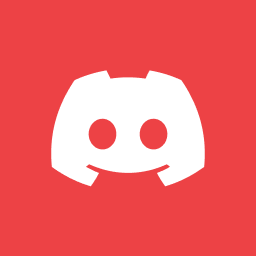
APP_WRITE_END_POINT=http://[MY IP]/80/v1 APP_WRITE_PROJECT_ID=6439bcdd4a97e9e4f785 APP_WRITE_DATABASE_ID=6439be5d13e3905d2cae APP_WRITE_USERS_COLLECTION_ID=643b112314651dfe809a APP_WRITE_EWEETS_COLLECTION_ID=643d33309b8f9a4e369f
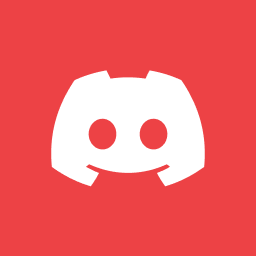
_APP_OPTIONS_FORCE_HTTPS i have not setted anything here
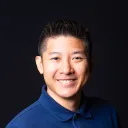
http://[MY IP]/80/v1
doesn't look right. I think it should be http://[MY IP]/v1
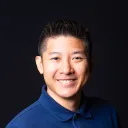
There should be some value set in the .env file
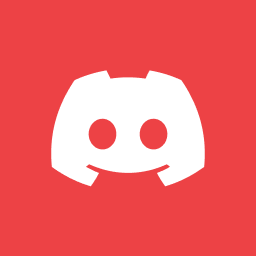
Oh Thank you so much it worked, like you said the problem was the extra 80.
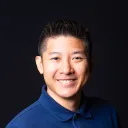
[SOLVED] Unhandled Exception: WebSocketException
Recommended threads
- Apple OAuth Scopes
Hi Hi, I've configured sign in with apple and this is the response i'm getting from apple once i've signed in. I cant find anywhere I set scopes. I remember se...
- Sign In With Apple OAuth Help
Hi All! I've got a flutter & appwrite app which Im trying to use sign in with apple for. I already have sign in with google working and the function is the sam...
- [SOLVED] OAuth With Google & Flutter
Hi all, I'm trying to sign in with google and it all goes swimmingly until the call back. I get a new user created on the appwrite dashboard however the flutte...
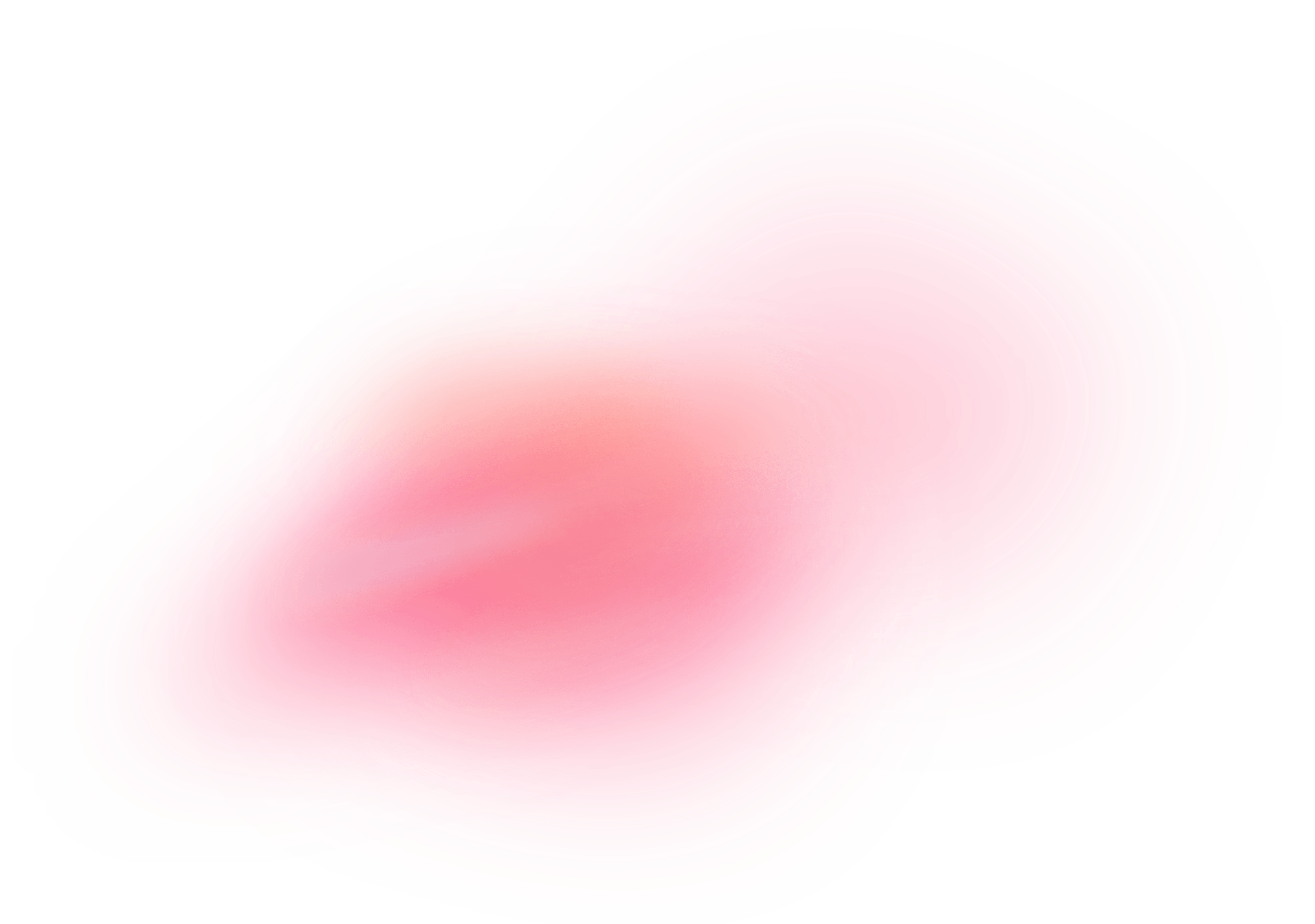