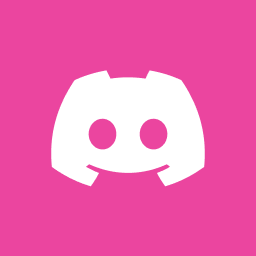
Hello, This is more of a best practice question, in Flutter I retrieve some documents into a list when the user opens the app. When the user clicks on a document it goes to a details page. I only want to listen to realtime events for that specific document, while user is viewing. Where is the best place to subscribe to the realtime events of this document? Should it be in the initState
or soon as the user clicks the item in the list? also I'm assuming on this page I should close the subscription in the dispose()
method. Thanks for any suggestions.
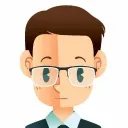
I think the best way would be
init in initState
as this is the best way to interact with the state
And close it in the dispose
method
This way you can assure you will listened to realtime event only and when it needed
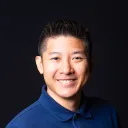
ya, this probably makes sense. @djcali, make sure you're using a new Realtime instance in this details page to make sure there's no race condition problem
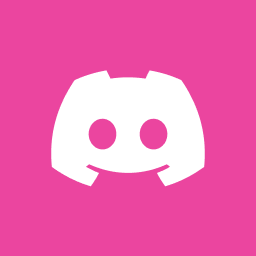
Oh man, I was wondering why I was having an issue with a bunch of realtime connections. It was doubling up. Thanks!
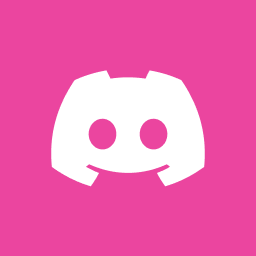
Works perfectly. Thanks for all the help.
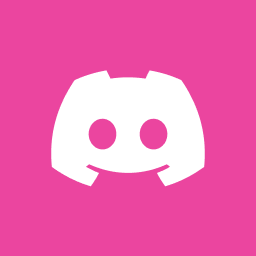
Hi, I have one issue. I'm not sure the correct way to resubscribe when it gets disposed by Flutter randomly. Here is what I have so far. I'm getting error trying to access a deactivated widget, because the way i'm resubscribing.
super.initState();
subscribe();
}
late RealtimeSubscription subscription;
void subscribe() {
debugPrint('Subscribing to realtime');
final client = ref.read(appwriteClientProvider);
final realtime = Realtime(client);
subscription = realtime.subscribe([
'databases.default.collections.events.documents.642ba977cff76a18a36c'
]);
subscription.stream.listen((response) {
print(response);
}, onDone: () {
subscribe();
});
}
@override
void dispose() {
subscription.close();
super.dispose();
} ```
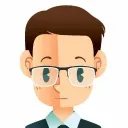
What is the error you're getting
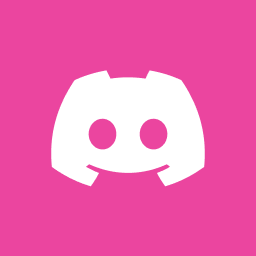
When I leave the Details page the Dispose()
method fires then the onDone
of the listen function fires because I'm trying to resubscribe. I want this to work unless I'm leaving the page. Hope this makes sense.
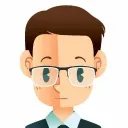
Got you What I usually do in this use-case is creating a flag that I will update in the dispose method like this.
private _isDone = false;
void initState() {
super.initState();
subscribe();
}
late RealtimeSubscription subscription;
void subscribe() {
debugPrint('Subscribing to realtime');
final client = ref.read(appwriteClientProvider);
final realtime = Realtime(client);
subscription = realtime.subscribe([
'databases.default.collections.events.documents.642ba977cff76a18a36c'
]);
subscription.stream.listen((response) {
print(response);
}, onDone: () {
if(!_isDone)
subscribe();
});
}
@override
void dispose() {
_isDone = true;
subscription.close();
super.dispose();
}
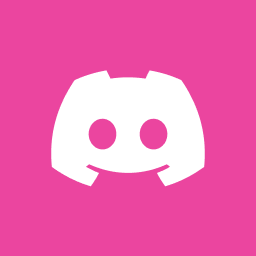
I think I tried something like this but I wasn't using a private variable. Going to try your way and report back. Thanks!
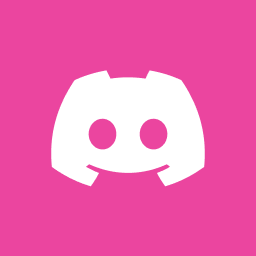
Worked perfectly. Thanks!
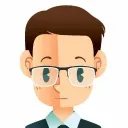
Perfect 😄
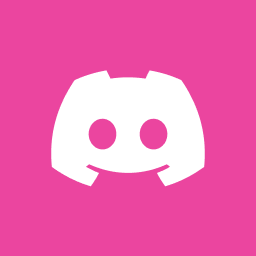
[SOLVED] Realtime in Flutter
Recommended threads
- Can't reach Frankfurt server
I have been developing an IoT device that senses an event, takes a picture and pushes the jpg to an appwrite project. I'm using a Sixfab Pico LTE board that cou...
- Flutter - FCM: App Crashes when receivin...
- Firebase Messaging Causes ANR on Notific...
I am working on a Flutter project where I have set up notifications using Firebase Messaging. Everything was working perfectly until recently. After coming back...
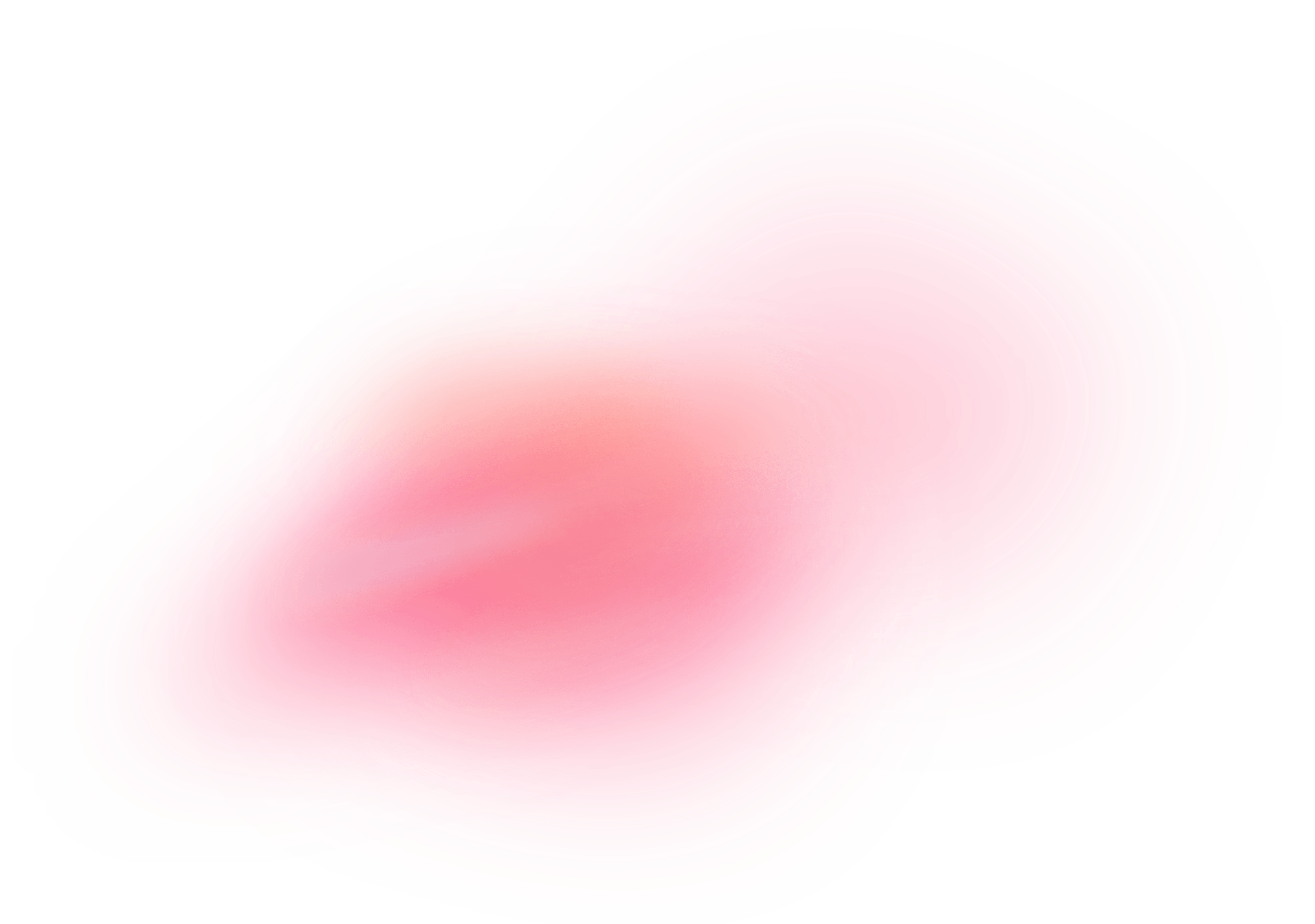