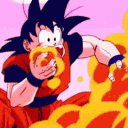
Hey, I'd like to create integration tests in flutter. I have a prod instance of appwrite, and I would like to be able to "clone" my prod db without prod users but instead add some dummy users for testing. How could I achieve this?
I want to spin it up locally, I want to keep configs and functions as they are, but basically remove all users, add a dummy admin user like test@test.com and dummy accounts for testing. I saw this gist https://gist.github.com/stnguyen90/fee636ff652b8ecbf761935b2aa254fb but im still a bit lost
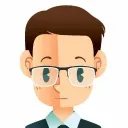
You can go manually
In this gist you can find how to connect directly to your MariaDB instance https://gist.github.com/byawitz/885510a4d9789e97424337e9472276d7
Then you should do something like this
- Do the volume backup part
appwrite_volumes=(uploads cache config certificates functions)
for volume in ${appwrite_volumes[@]}; do
mkdir -p backup && docker run --rm --volumes-from "$(docker compose ps -q appwrite)" -v $PWD/backup:/backup ubuntu bash -c "cd /storage/$volume && tar cvf /backup/$volume.tar ."
done
and restore them in your machine
appwrite_volumes=(uploads cache config certificates functions)
for volume in ${appwrite_volumes[@]}; do
docker run --rm --volumes-from "$(docker compose ps -q appwrite)" -v $PWD/backup:/restore ubuntu bash -c "cd /storage/$volume && tar xvf /restore/$volume.tar --strip 1"
done
- Export all your database from your remote db.
- Import only needed tables
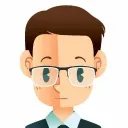
Or you can just edit your local db _users_project
table
note: table name can be different dependents on your project
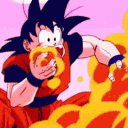
Can I added users via the docker-compose file? Postgres containers e.g. let me define .sql files that I need to put into a specific directory, is there something similar with the provided mariadb container?
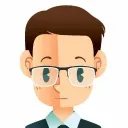
But the postgres container are just defining the Database user not your registered users.
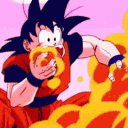
I don't want to have to have to set up users manually, especially because when im going to restore new data, the manual setup would be gone, right?
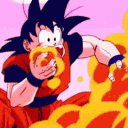
Sorry, I think the right term in appwrite would be accounts
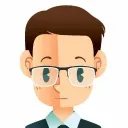
Yes
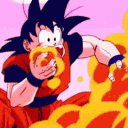
I want to create dummy accounts when booting up appwrite locally and delete all prod accounts
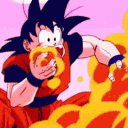
and I would also like to have a admin user, that is different to the prod admin user, but still has access to the project
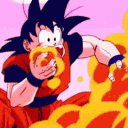
Im not sure where the accounts and users are saved, but it seems like both are saved in mariadb right?
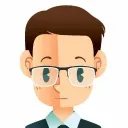
Okay, To do so automatically you can create a code snippet that will use any Appwrite server SDKs Then
- Get the users
- Delete them
- Generate Random users
const sdk = require('node-appwrite');
// Init SDK
const client = new sdk.Client();
const users = new sdk.Users(client);
client
.setEndpoint('https://[HOSTNAME_OR_IP]/v1') // Your API Endpoint
.setProject('5df5acd0d48c2') // Your project ID
.setKey('919c2d18fb5d4...a2ae413da83346ad2') // Your secret API key
;
// Get the users
const res =await users.list();
// Delete all prod users
res.users.forEach(user=>{
await users.delete(user.$id);
});
// Create new users
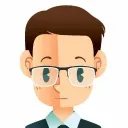
Yes they are
The console users are saved inside table named _console_users
And for each project it's inside _n_users
, For example _1_users
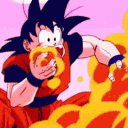
Thank you so much!
Recommended threads
- Error 1.7.4 console team no found
In console when i go to auth, select user, select a membership the url not work. Only work searching the team. It is by the region. project-default- and i get ...
- Is Quick Start for function creation wor...
I am trying to create a Node.js function using the Quick Start feature. It fails and tells me that it could not locate the package.json file. Isn't Quick Start ...
- functions of 1.4 not work on 1.7
Hi, i updated of 1.4 to 1.7 but the function not work i get it error. Do I need to build and deploy the functions again?
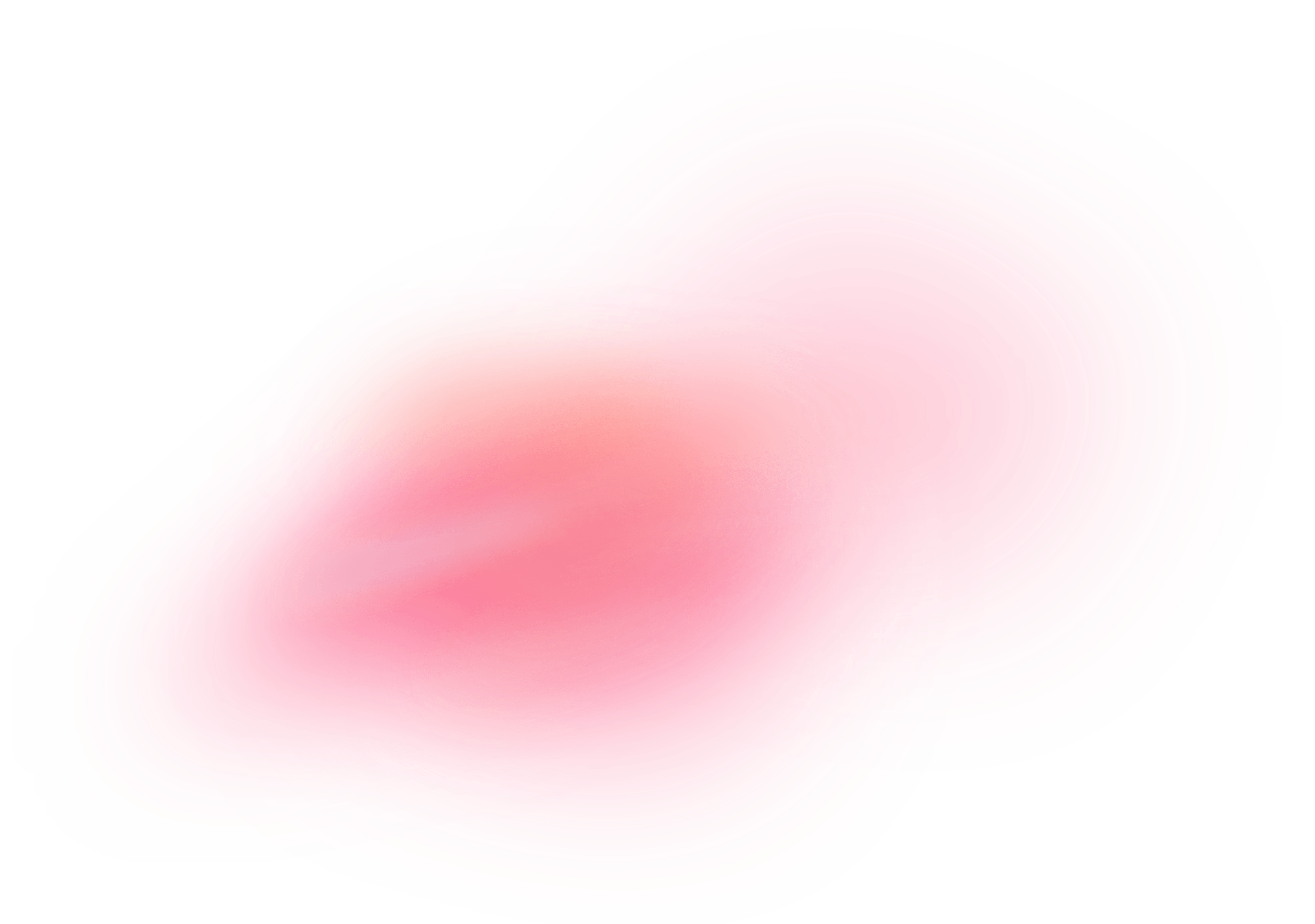