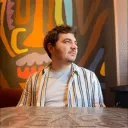
Hi,
I created an SQL dump of users in appwrite, I currently hae users with argon and bcrypt password.
I need values for: bcrypt: $<algorithm version>$<cost>$<salt & hash> argon: $argon2i$v=19$m=4096,t=3,p=1$4t6CL3P7YiHBtwESXawI8Hm20zJj4cs7/4/G3c187e0$m7RQFczcKr5bIR0IIxbpO2P0tyrLjf3eUW3M3QSwnLc
Can someone help get these calculated in nodejs?
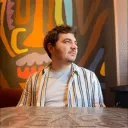
I created this function:
(async () => {
const usersText = readFileSync("./csvjson.json", "utf-8");
const { _1_users: users } = JSON.parse(usersText);
const mappedUsers = users.map((user) => ({
hash: user.hash,
password: JSON.parse(user.password.replaceAll('\\"', '"')),
options: JSON.parse(user.hashOptions.replaceAll('\\"', '"')),
}));
for (const user of mappedUsers) {
console.log(user);
const decryptedData = decryptPasswordData(user);
const passwordDigest = getPasswordDigest(user, decryptedData);
console.log("Generated password digest:", passwordDigest);
}
console.log(mappedUsers);
})();
function decryptPasswordData(user) {
const decipher = crypto.createDecipheriv(
user.password.method,
crypto.scryptSync(secretKey, user.password.iv, 16),
Buffer.from(user.password.iv, "hex")
);
decipher.setAuthTag(Buffer.from(user.password.tag, "hex"));
const decryptedData = Buffer.concat([
decipher.update(Buffer.from(user.password.data, "base64")),
decipher.final(),
]).toString("utf8");
return decryptedData;
}
function getPasswordDigest(user, decryptedData) {
if (user.hash === "bcrypt") {
const cost = user.options.cost;
return `$${user.options.type}$${cost}$${decryptedData}`;
} else if (user.hash === "argon2") {
const params = [user.options.type, user.options.memoryCost, user.options.timeCost, user.options.threads];
return `$${params.join(",")}$$${decryptedData}`;
} else {
throw new Error(`Unsupported hash type: ${user.hash}`);
}
}
However getting this error:
node:internal/crypto/cipher:193
const ret = this[kHandle].final();
^
Error: Unsupported state or unable to authenticate data
Seems like I am not sure what the secret key is used by Appwrite Auth.
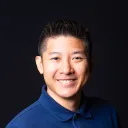
Why are you pulling passwords with SQL dump? What are you trying to do?
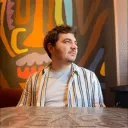
@Steven trying to migrate users to clerk.dev
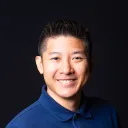
Are you trying to get the users' plain text password? Appwrite doesn't store plain text passwords as that's not secure. You can use the list users API to get the users' and their hashed passwords
Recommended threads
- Is Quick Start for function creation wor...
I am trying to create a Node.js function using the Quick Start feature. It fails and tells me that it could not locate the package.json file. Isn't Quick Start ...
- Appwrite Fra Cloud Custom Domains Issue
Iām trying to configure my custom domain appwrite.qnarweb.com (CNAME pointing to fra.cloud.appwrite.io with Cloudflare proxy disabled) but encountering a TLS ce...
- Appwrite service :: getCurrentUser :: Us...
Getting this error while creating a react app can someone please help me solve the error
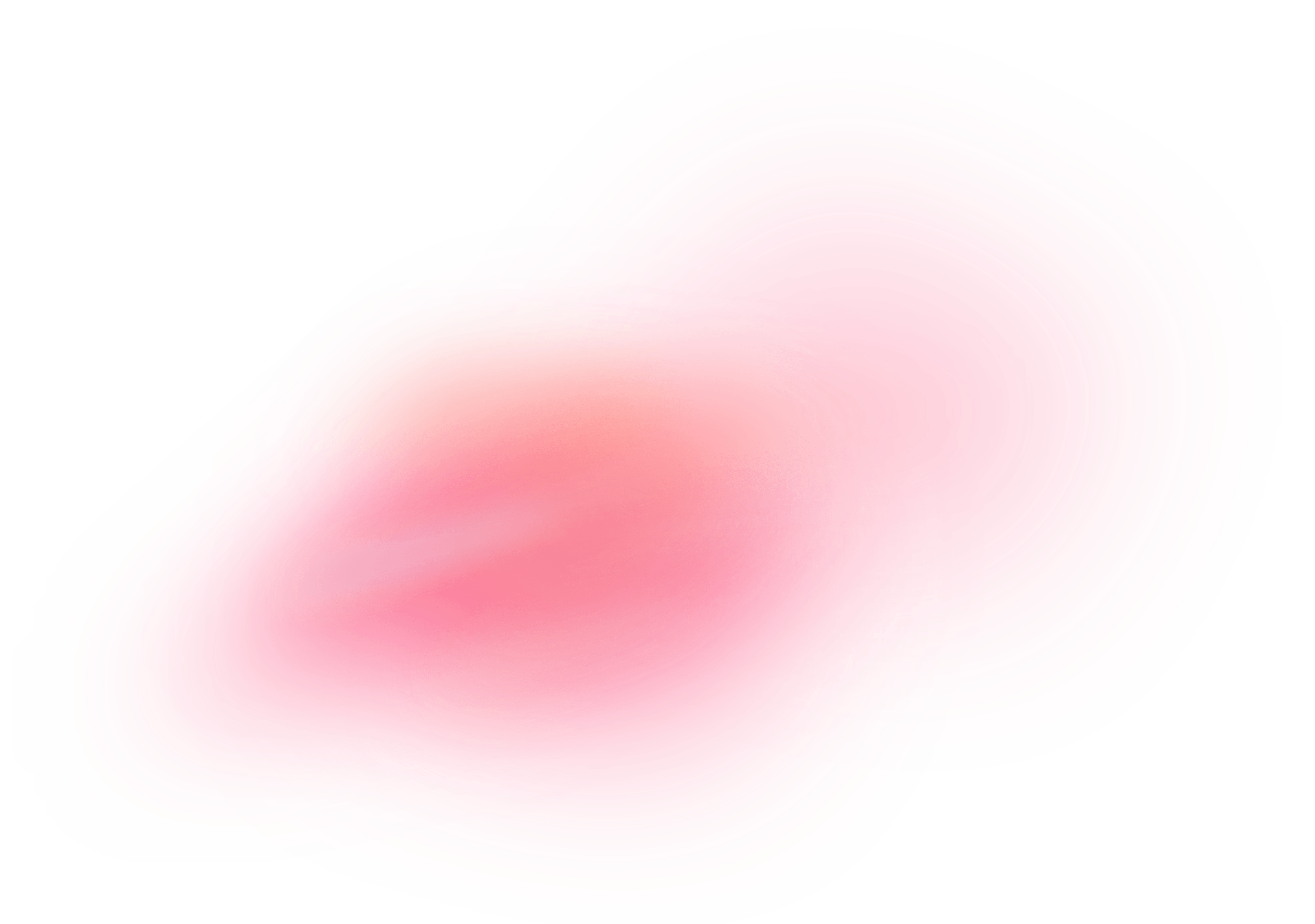