"Failed to verify JWT. Invalid token: Expired" when getDocument using setKey permission in nodejs
- 0
- Databases
- Self Hosted
- Web
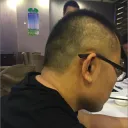
Sometimes not often this error occurs.
What puzzled me was that I used the setKey method for authorization, but there was an error with JWT expiration.
Key Code Fragments:
// libs/nodeAppwrite.ts import { Client, Account, Databases, ID, Permission, Role, Query, Users, } from 'node-appwrite'
// others
const client = new Client() .setEndpoint(process.env.NEXT_PUBLIC_APPWRITE_ENDPOINT) .setProject(process.env.NEXT_PUBLIC_APPWRITE_PROJECT) .setKey(process.env.APPWRITE_API_KEY) const database = new Databases(client) // others
const database = new Databases(client)
// pages/api/getDocument.ts import nodeAppwrite from '@/libs/nodeAppwrite' // others const documentRes = (await nodeappwrite.database.getDocument( process.env.NEXT_PUBLIC_APPWRITE_DATABASE_ID, process.env.NEXT_PUBLIC_APPWRITE_PAYMENTS_COLLECTION_ID, documentId )) as any // others
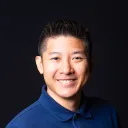
Can you share the exact error?
Btw, it's best to use 3 back ticks with multi-line code. See https://www.markdownguide.org/extended-syntax/#syntax-highlighting
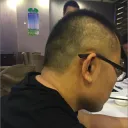
Yes, Some errors in docker logs:
[Error] Timestamp: 2023-04-08T02:04:44+00:00 [Error] Method: GET [Error] URL: /v1/databases/:databaseId/collections/:collectionId/documents/:documentId [Error] Type: Appwrite\Extend\Exception [Error] Message: Failed to verify JWT. Invalid token: Expired [Error] File: /usr/src/code/app/init.php [Error] Line: 865
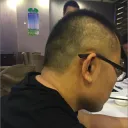
And response is:
{"code":401,"type":"","response":{"code":401,"response":{"size":0,"timeout":0}}}
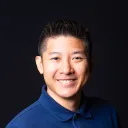
Are you calling setJWT()
anywhere?
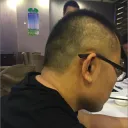
Yes, I had setJWT while user login to server from client to get account info.
But the getDocument request is not a user behavior, it's a behavior similar to a web hook.
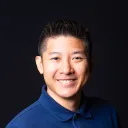
Somehow the same client that had setJWT()
is being used
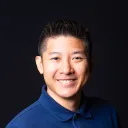
Oh...client is probably global and being reused across requests
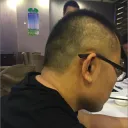
Oh, what should I do.
When both methods are used simultaneously and initiated by two different clients, cannot it be distinguished.
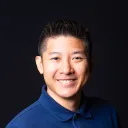
Best to use different clients with API key vs jwt. Also, make sure to use a request scoped client when using jwt token
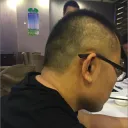
I confirm that these two requests occurred on different clients. Jwt is only intended for users, while API keys are used in other situations.
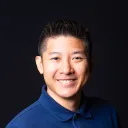
What's the code for setting the JWT?
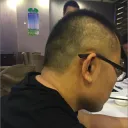
Although it is a different client request, is it related to setKey and setJWT being executed on the same server.
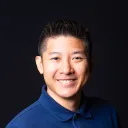
So that's probably why it's being reused...
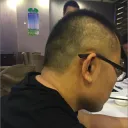
When user request login.ts api I handle the request with iron-session:
import { withSessionRoute } from '@/helper/withSession'
import nodeAppwrite from '@/helper/nodeAppwrite'
import type { NextApiRequest, NextApiResponse } from 'next'
const handler = async (req: NextApiRequest, res: NextApiResponse) => {
const { jwt, appwriteSessionId } = req.body
if (!jwt || !appwriteSessionId) {
res.status(401).json({ message: 'Unauthorized' })
}
try {
nodeAppwrite.client.setJWT(jwt)
await nodeAppwrite.account.get().then(
async (response) => {
const nowTime = Date.now()
const newUser = {
...response,
appwriteSessionId,
}
req.session['user'] = newUser
req.session['jwt'] = {
value: jwt,
expiresAt: nowTime + 15 * 60 * 1000,
}
await req.session.save()
res.send(newUser)
},
(error) => {
res.status(error.code ?? 401).json({ message: error.message })
}
)
} catch (error) {
res.status(error.code ?? 500).json({ message: error.message })
}
}
export default withSessionRoute(handler)
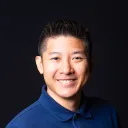
You're importing the same global nodeAppwrite.client
instance here...
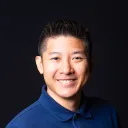
Try to instantiate a new Client and Account instead
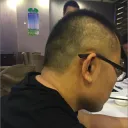
Thank you Steven. I will try your suggestion.
Recommended threads
- how many Teams can be created?
I am creating an app where I will let users create groups. This could mean there will be many groups created by user, to isolate those groups properly I am thin...
- Cannot create a user
Hi, I am using a lowcoder frontend and trying to create a user in Appwrite (python function). Unfortunately, all I got is an error: "Raw body: Error". It means...
- React native app login via Safari
Hi! I deployed for debug my React Native app in web, chrome everythink works well but in safari on mac and ios I cant login. I found this one error in safari co...
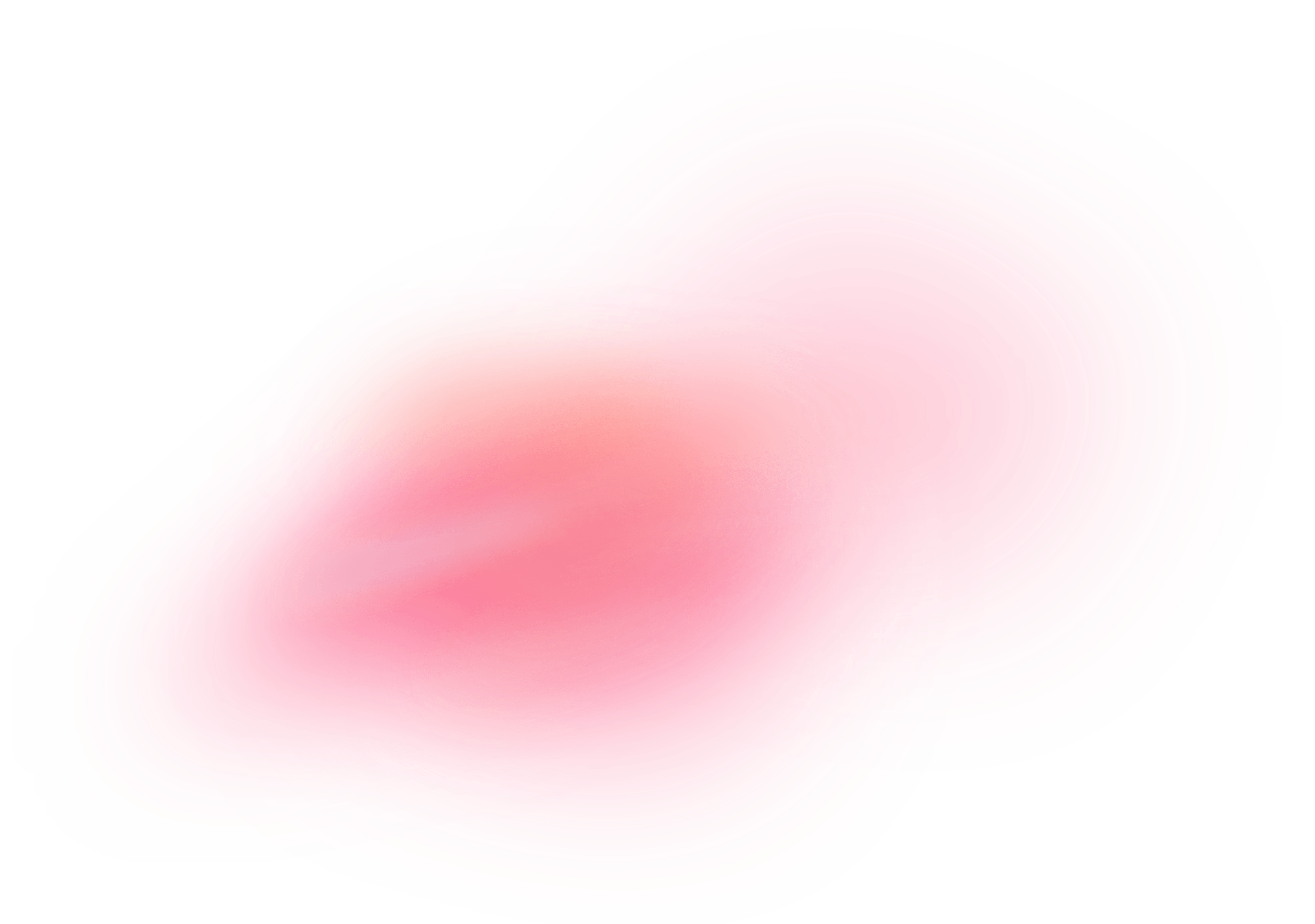